Autogenerate C# Class using JSON string or JSON Schema
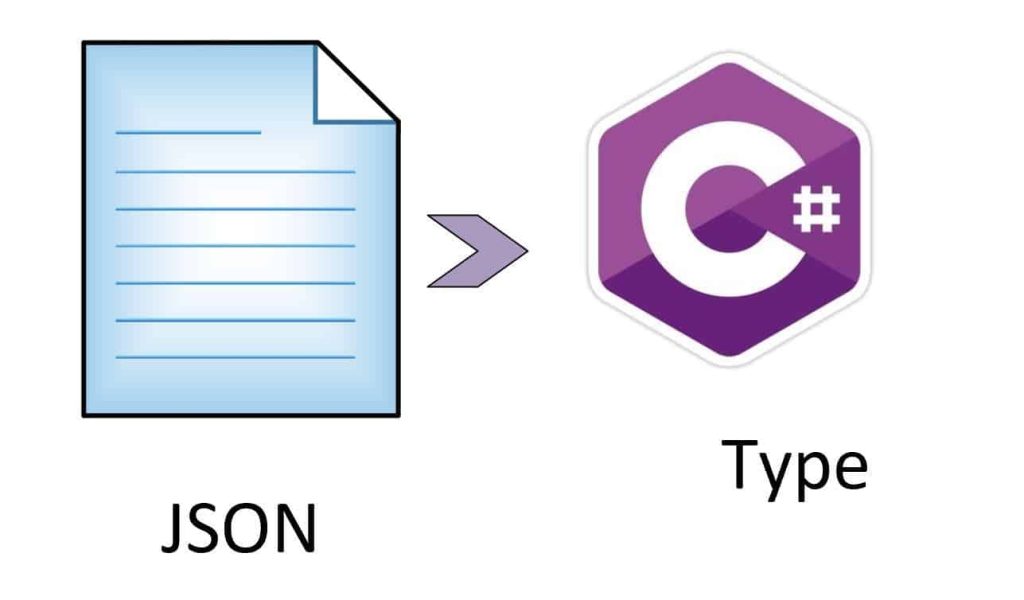
Today in this article, we will see a few techniques of how to autogenerate C# Class using JSON string or JSON Schema.
You might find the need for converting JSON schema to C# types or Typescript types while dealing with JSON format.
As we know JSON is a very popular format for information exchange, especially when dealing with information exchanges between Microservices using RESTFul principles.
We shall see how we can use NJsonSchema a C# library to create C# classes easily.
We will also see Visual Studio ‘Paste Special‘ functionality to achieve the same.
Today in this article, we will cover below aspects,
One can create C# classes for any given JSON in the form of a request or type or schema file.
Using NJsonSchema for creating classes
NJsonSchema is a .NET library to read, generate and validate JSON Schema. It provides basic code generation APIs, which can be used to generate C# classes or generate TypeScript classes or interfaces from a JSON schema.
The library reads a schema from a file or string and validates JSON data against it.
This library can be used to generate a schema from existing .NET classes.
Below are a few high-level features about this library,
- Generate C# code from JSON Schema
- Generate TypeScript code from JSON Schema
- Generate JSON Schema from JSON request or data file
- Generate JSON Schema from .NET classes/type via reflection
- Supports JSON Schema, Swagger and OpenAPI DTO schemas
- Read existing JSON Schemas and validate JSON data
Below the article, we shall see each feature with a simple example.
Getting started
Create any .NET Core application
- Install Nuget package,
Install-Package NJsonSchema.CodeGeneration.CSharp -Version 10.1.2
OR

Please use below schema file to generate the classes. One can use schema or JSON files directly.
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"ID": {
"type": "string"
},
"Name": {
"type": "string"
},
"City": {
"type": "string"
},
"Country": {
"type": "string"
}
},
"required": [
"ID",
"Name",
"City",
"Country"
]
}
OR
Let’s use below sample Employee.json file,
{
"ID": "1002",
"Name": "ABCD",
"City": "city",
"Country": "USA"
}
One can write the below logic to generate classes from a given JSON request.
string json = File.ReadAllText("Employee.json");
var schemaFromFile = JsonSchema.FromSampleJson(json);
var classGenerator = new CSharpGenerator(schemaFromFile, new CSharpGeneratorSettings
{
ClassStyle = CSharpClassStyle.Poco,
});
var codeFile = classGenerator.GenerateFile();
File.WriteAllText("C://Test/Employee1.cs", codeFile);
The generated files will be saved in the path specified above.
Below is the file generated using the above code,

Note:
You might get the below error due to conflicts between Newtonsfot Vs NJSonschema classes.
Unable to cast object of type ‘Newtonsoft.Json.Schema.JsonSchema’ to type ‘NJsonSchema.JsonSchema’.
Please use the namespace as below to fix the issue,
using JsonSchema = NJsonSchema.JsonSchema;
Using Visual Studio to Generate Classes
Alternatively, Visual Studio has the “Paste Special” capability to create c# classes from JSON.
Visual Studio – -> Edit — > Paste Special
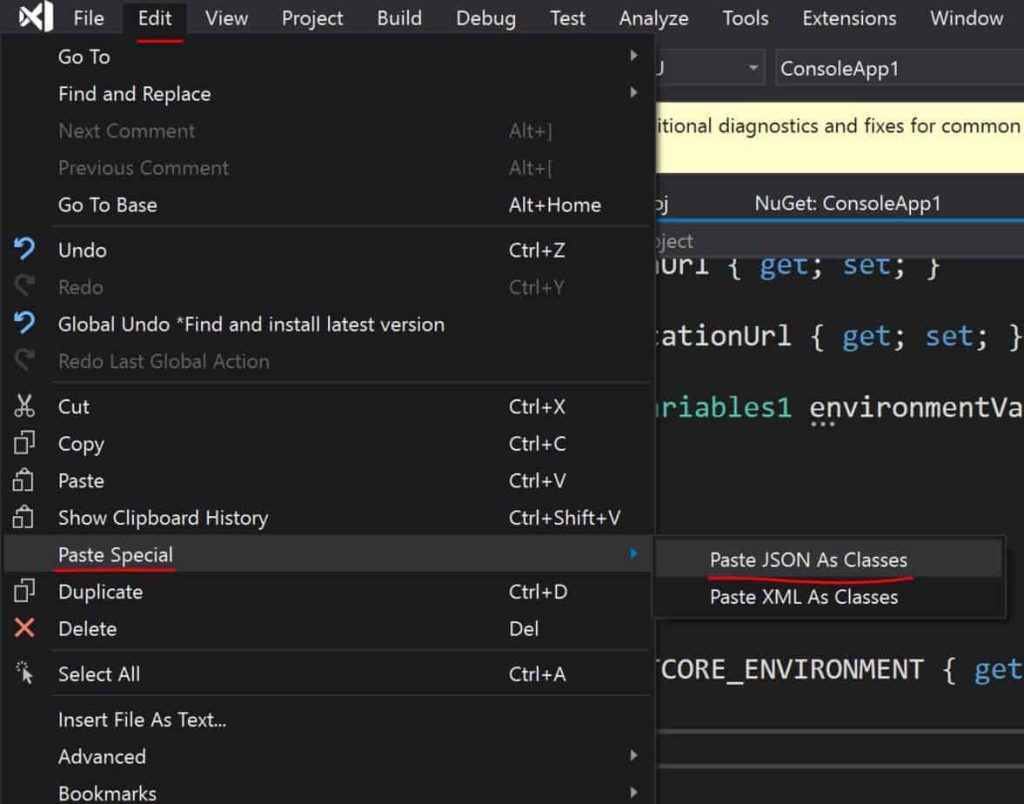
The generated class looks as below for the same Employee.json schema,
public class Rootobject
{
public string ID { get; set; }
public string Name { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
Above you need to change a root object to the appropriate name of your choice.
Happy Coding !!
Other useful references,
- Generate TypeScript code from JSON Schema
- Generate JSON Schema from JSON request or data file
- Generate JSON Schema from .NET classes/type via reflection
Summary
Today in this article, we looked at a few easy techniques for creating C# classes from a given JSON schema. We saw how to use the NJsonSchema CodeGeneration library and also looked at Visual Studio Paste special features to generate required C# types.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.