Dependency Injection using Generic HostBuilder in .NET Core Windows Form

In this post, we shall learn and attempt to perform Dependency Injection in .NET Core Windows Form using Generic HostBuilder.
We already learned the one approach of DI using Service collection and ServiceProvider for Windows forms app which works perfectly fine.
Until now and still many implementation-prefer and rely on service collection classes for registering the dependency and using it through DI container.
Today in this post we will be using Generic HostBuilder to create DI Container and inject the required dependency within the Windows Forms app.
Today in this article, we will cover below aspects,
Getting started
Here I am using a Forms/Windows .NET Core 3.1 application.

Creating Generic HostBuilder
Generic HostBuilder was introduced in .NET Core 2.1 and designed to be used for both Non-HTTP and HTTP workloads.
The HostBuilder class is available from the following namespace,
using Microsoft.Extensions.Hosting;
HostBuilder implements the IHostBuilder interface.
Please install the NuGet package from Nuget Package manager or PMC,
PM> Install-Package Microsoft.Extensions.Hosting -Version 3.1.1
Please create Generic HosBuilder and register the dependencies that need to inject. These changes can be done in the Main() method.
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddSingleton<Form1>();
services.AddLogging(configure => configure.AddConsole());
services.AddScoped<IBusinessLayer, BusinessLayer>();
services.AddScoped<IDataAccessLayer, CDataAccessLayer>();
})
Initialize the Host
Initialize the Host with all dependencies,
var host = builder.Build();
Or
By using generic host builder CreateDefaultBuilder
host = Host.CreateDefaultBuilder()
.ConfigureServices((hostContext, services) =>
{
}).Build();
Configure IoC Container- Dependency Injection in .NET Core Windows Form
We shall be injecting Logger and BusinessLayer objects for more precise results. Please see below the complete code which we need to put in in Main() method application,
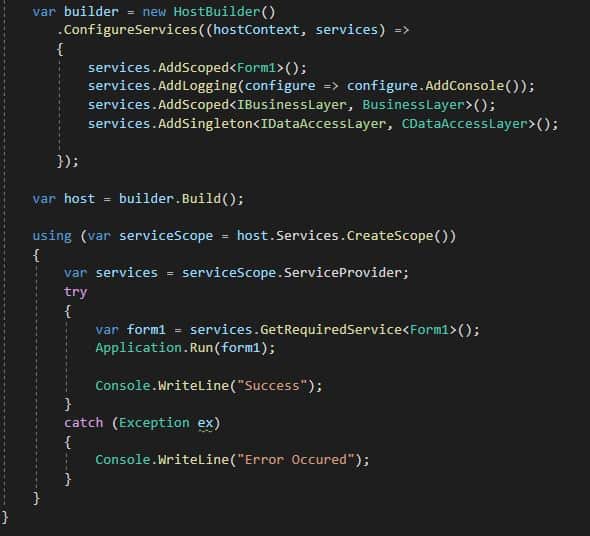
Here we are assuming Form1 as the master Form that will be an entry point for all other forms and their action.
However, you can add an additional layer separating actual UI components if needed.
All the services registered using HostBuilder are now available to use as per need. The lifetime management of those services will be taken care of by HostBuilder and we need not have disposed off them.
Form class for your references as below,
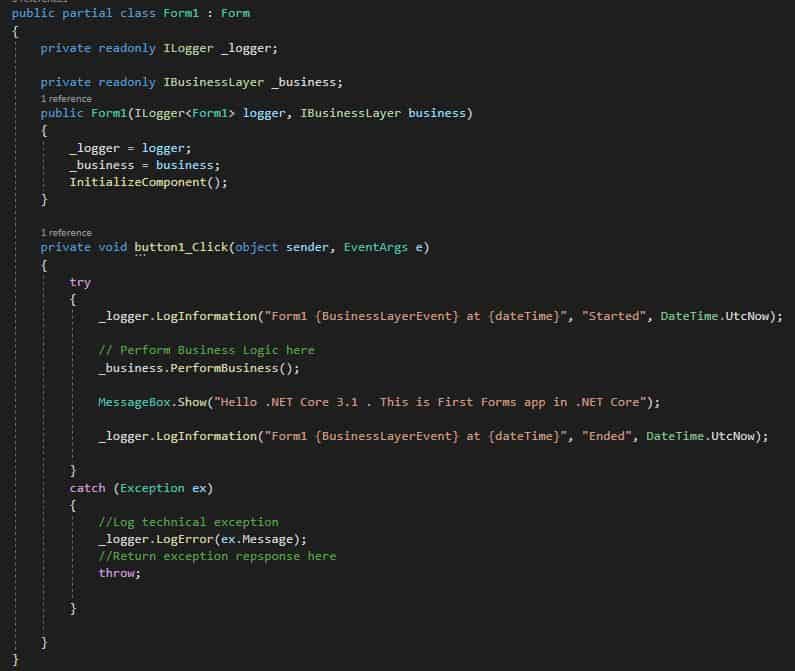
Let’s execute the application and try a button click action,
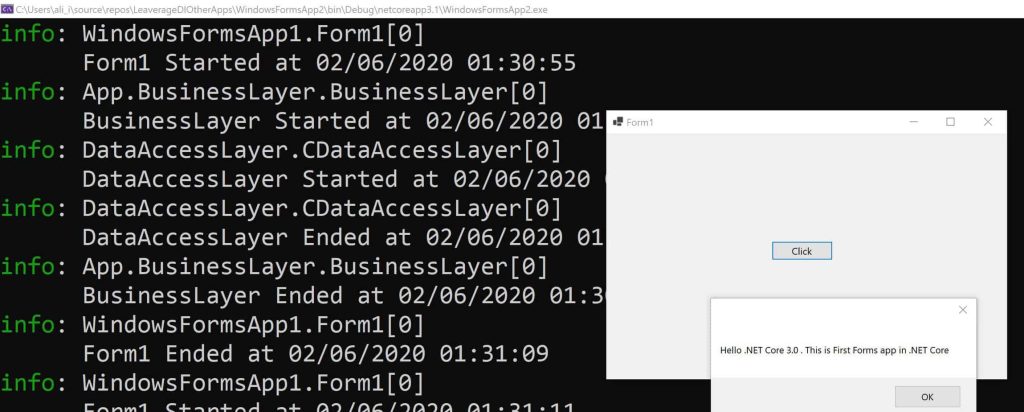
Note: Above console logs were intentionally made it to the console view so that events can be captured.
If interested to know on one more approach, please see below post,
Can we make the above code better?
Please let me know if you have any better suggestions for performing DI in the Desktop application.
Please sound off your comments below.
Summary
Today we learned and attempted one more approach for leveraging the Dependency Injection (DI) in newly supported Windows forms applications in the .NET Core ecosystem. We learned that using Hostbuilder we can easily leverage DI in Desktop or Forms application and can create highly maintainable and extensible Win Form or Desktop applications.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.