Logging in ASP.NET Core

Logging in ASP.NET Core framework is simplified further. Today in this article we will cover basic aspects of Logging in ASP.NET Core.
I found that version 3.1 or 5.0 has similar support for logging and follows a similar type of provider as in the previous older version of .NET Core. The overall recent framework uses Generic HostBuilder(which I experienced keeps changing with each new .NET Core version. We will see that below in detail.)
Logging makes use of the same dependency injection (DI) principle to inject the generic logging interface ILogger. The configuration will be used as defined in Startup.cs or Programs.cs.
Logging using the Default provider
.NET Core framework comes with default built-in logging providers.
That means you really need any third-party components until existing providers suffices the requirement.
Please make a note that logging will support built providers like Console, Debug, EventSource.
That means as long as you are using the existing providers, logging will not require any changes and the framework will take care of logging on Console or Debug or EventSource as configured.
This configuration will work for the Log Level of your choice as specified in appsetting.json etc with some basic changes by enabling logging in either Startup.cs or ConfigureServices() method.
Logging providers support in the framework by default,
- Console
- Debug
- EventSource
Let’s understand how to enable logging and how to do any customization to existing behavior using an example.
Create an API using ASP.NET Core
Please create API using ASP.NET Core 3.1 or 5.0,
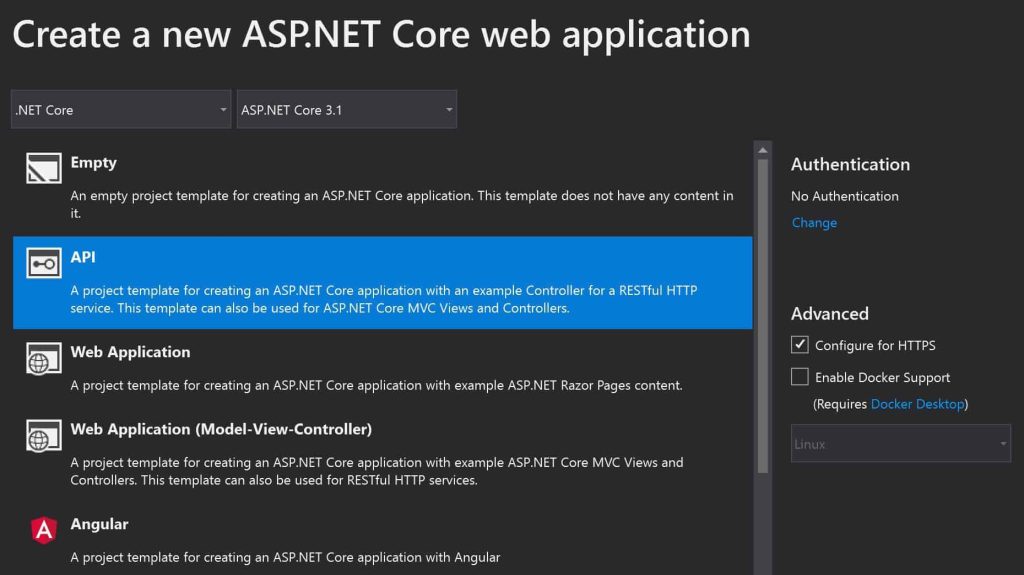
Once the project is created and please open Program.cs

As we discussed logging will be enabled with default providers. As shown above, the default project template calls CreateDefaultBuilder() which adds preconfigured logging providers like Console, Debug, and EventSource(new).
CreateDefaultBuilder a generic Host builder in .NET and ASP.NET Core plays an important role in initializing the Host and its configuration like getting access to applications host details including app configuration, logger configuration, user secretes, getting access to environmental configuration, etc.
For more details, please see visit CreateDefaultBuilder and Configuration Management in .NET/ASP.NET Core
So let’s create a logger instance within the controller. Logger instance can be explicitly DI (Dependency Inversion) as below,

Changing Default Logging provider
The default logging providers can be modified in two possible ways.
Below are two techniques that demonstrate how to add new providers or make changes to existing default providers if required.
Please make sure to add below using namespace reference to Startup.cs.
"using Microsoft.Extensions.Logging"
1. Using Program.cs to Enable logging
Here we will try to remove the default provider and will enable only the Console provider.
public static IWebHostBuilder CreateWebHostBuilder(string[] args) =>
WebHost.CreateDefaultBuilder(args)
.UseStartup<startup>()
.ConfigureLogging((hostingContext, logging) =>
{
logging.ClearProviders();
logging.AddConsole();//Add Console logging
});
}
As we discussed above CreateDefaultBuilder() method will add support for Console, Debug, and EventSource providers.
This method will also load the logging configuration provided in appsettings.json or appsettings.{env.EnvironmentName}.json.
You can load custom configurations if needed using configuration management techniques.
In the above example since we only need a Console logging provider, we need to remove all default providers by calling logging.ClearProviders() followed by adding AddConsole().
2. Using Services i.e ConfigureServices() to enable logging
Here again, we will try to remove the default providers and will enable only the Console logging provider using services. In Startup.cs please update the ConfigureService() method as below to be used within the pipeline.
Using ConfigureServices is a preferred technique as it will not pollute your Program.cs.
This technique also helps to centralize the code related to cross-cutting concerns.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_2);
services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddConsole();
});
}
Both above techniques will produce the console logging result as below.
I have used the below command to verify console logging.
dotnet run
The above commands can be run through Command prompt within the project file. I have used Fiddler for the client-side call.

Built-in Logging providers
ASP.Net Core supports overall built-in logging providers.
Please note that using namespace( Microsoft.Extensions.Logging) which we added above will provide supported methods like Clearprovider() or AddConsole() etc.
- Console – To enable only console logging please use the below code within ConfigureServices() method.
services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddConsole();
}
- Debug – To enable only Debug logging please use the below code to ConfigureServices() method.
services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddDebug();
}
- EventSource – To enable only EventSource logging please use the below code to ConfigureServices() method.
services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddEventSourceLogger();
}
- EventLog – Please add the Nuget package “Microsoft.Diagnostics.Tracing.EventSource”

To enable Event logging please use the below code in ConfigureServices() method.
services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddEventSourceLogger();
}
- TraceSource – To enable TraceSource please add below Nuget package called “Microsoft.Extensions.Logging.TraceSource“. This logger logs messages to a trace listener by writing messages with System.Diagnostics.TraceSource.TraceEvent()

services.AddLogging(loggingBuilder =>
{
loggingBuilder.ClearProviders();
loggingBuilder.AddTraceSource(switchName);
}
[/cc]
Additionally below logging providers more specific to Azure are also supported,
- Azure App Service provider -Please use NuGet package
Microsoft.Extensions.Logging.AzureAppServices
- Azure App Service provider– Please use NuGet package
Microsoft.Extensions.Logging.AzureAppServices
- Azure Application Insights trace logging– Please use NuGet package
Microsoft.Extensions.Logging.ApplicationInsights
Logging configuration in appsettings.json for all above samples used as below,
{
"Logging": {
"LogLevel": {
"Default": "Debug"
}
}
}
Logging configuration provided in appsettings.json or appsettings.{env.EnvironmentName}.json will be loaded automatically by CreateDefaultBuilder () method.
For more details, please see the visit article on CreateDefaultBuilder and Configuration Management in .NET/ASP.NET Core
Custom Configuration for Logging
One can load the custom configuration using ConfigureAppConfiguration() method.
As shown below AddEnviornmentVariables will load environment-specific variables etc.
In a similar way please add a provider of your choice as required to load custom configuration accordingly.

Please see more details below on loading custom configuration,
Above we did see a different provider support different logging requirements. But I still don’t see much-needed i.e File or Rolling file logging provider.
However, I have talked about how to enable File/Rolling File logging in .NET Core WebAPI article if you are interested.
Log
Let’s run the application and see logging,

The above GET method once executed will log the required details to Console, Debug, or EventSource, etc. This logging, we get out of the box from the framework.
Here is an example of Debug log,

Similarly, Console log,

Other References :
- Logging in .NET Core Console application – DI (dependency injection) technique.
- How to implement dependency injection (DI) in a .NET Core Console application for logging and other business requirements
Summary
In this post, we learned the basics of logging in to ASP.NET Core. We also learned about a few updates to the logging framework in ASP.NET Core 3.1 or 5.0. We examined different logging providers and learned how to enable logging using different types of logging providers.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.