Basic Authentication in Swagger (OpenAPI) ASP.NET Core

In today’s article, we shall discuss, how to enable basic authentication in Swagger (OpenAPI) documentation in ASP.NET Core 3.1 or .NET 5 API applications.
Today in this article, we will cover below aspects,
I already talked about ASP.NET Core 2.2-based Basic authentication Swagger usages in the below article.
We had used Swagger v2.0 specifications while adding the swagger documentation in supporting ASP.NET Core 2.2.
ASP.NET Core 3.1 and above onwards OpenAPI v3.0 document specifications are followed. Swashbuckle tools help us follow these specifications and generated nice documentation for the API.
So, Let’s get started,
Create ASP.NET Core API application
Please create ASP.NET Core 3.1 or .NET 5 application,

Add Swashbuckle.AspNetCore NuGet package
Please add the below Nuget package to your WebAPI using a Command prompt or PMC(package manager console)
PM> Install-Package Swashbuckle.AspNetCore -Version 5.3.3
Note- Please use the latest available version
This NuGet package shall add all other required components as shown below and you need not have to add them explicitly,
- Swashbuckle.AspNetCore.SwaggerUI
- Swashbuckle.AspNetCore.Swagger
- Swashbuckle.AspNetCore.SwaggerGen
Update ConfigureServices() method as below
/// <summary>
/// ConfigureServices
/// </summary>
/// <param name="services"></param>
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "TheCodeBuzz-Service", Version = "v1" });
c.AddSecurityDefinition("basic", new OpenApiSecurityScheme
{
Name = "Authorization",
Type = SecuritySchemeType.Http,
Scheme = "basic",
In = ParameterLocation.Header,
Description = "Basic Authorization header using the Bearer scheme."
});
c.AddSecurityRequirement(new OpenApiSecurityRequirement
{
{
new OpenApiSecurityScheme
{
Reference = new OpenApiReference
{
Type = ReferenceType.SecurityScheme,
Id = "basic"
}
},
new string[] {}
}
});
});
The above methods like AddSecurityDefinition() and AddSecurityRequirement(), let you define your API security by defining one or more security schemes.
It lets you enable the below schemes to Swagger (Open API) documentation,
Update Configure() method
Let’s register the Swagger UI in the API pipeline. Please use UseSwaggerUI() extension method as below,
/// <summary>
/// Configure
/// </summary>
/// <param name="app"></param>
/// <param name="env"></param>
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "TestService");
});
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
The generated swagger definition will look as below.

The ‘Authorize‘ button will be displayed as above.
Please click on the Authorize button and provide basic authentication credentials i.e ‘Username’ and ‘Password’

Response – Success ( 200)
Let’s execute one of the methods with Basic authentication credentials,

Response – Unauthorized ( 401)
If the basic with credentials are is invalid – You would receive 401: Unauthorized error.
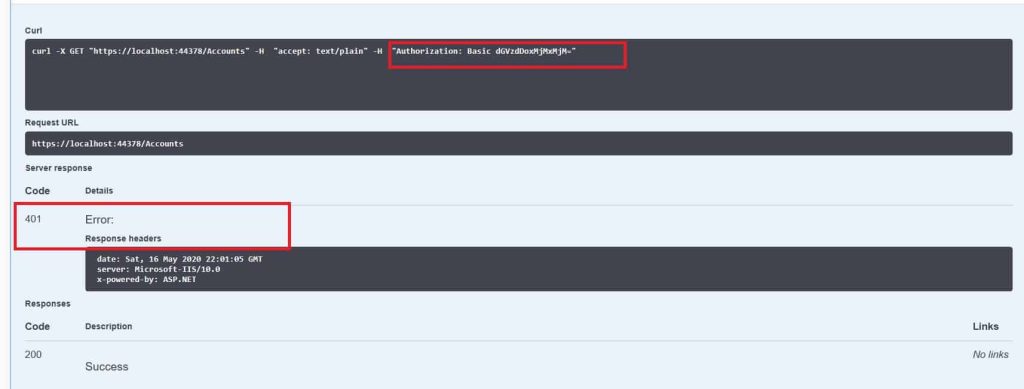
If the credentials are invalid you should receive 401: Unauthorized error.
Note: Swagger uses already enabled BasicAuthenticationHandler to verify the basic authentication credentials if implemented. For more details on adding Basic authentication, please visit article.
ASP.NET Core 5 – Basic Authentication in ASP.NET Core API with example
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Other references :
Summary
In this post, we learned how to add Basic Authentication to Swagger(OPEN API) documentation to ASP.NET Core 3.1 application. Swagger or OpenAPI describes the standards and specifications for RESTFul API descriptions. Today we looked at enabling authentication schemes i.e BasicAuthScheme using the Swashbuckle within the ASP.NET Core API.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.