Read CSV and Convert to JSON in Python
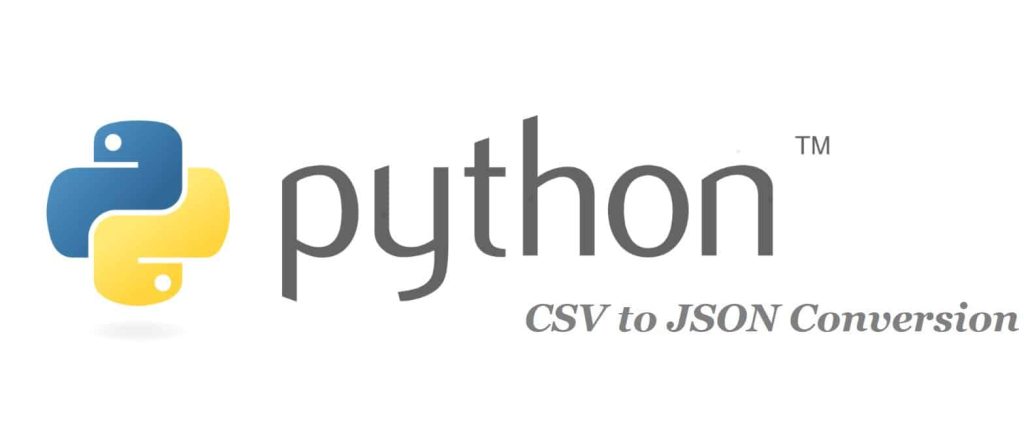
Today in this article, we will see how to Read CSV and Convert to JSON using Python. Then we will see how to convert a CSV to JSON so that it becomes consumable by API or different other applications.
Today in this article, we will cover below aspects,
Today we shall see how to use Pandas library for performing simple read and write.
Getting started
Please create Python application.
Please install below pandas package,
pip3 install pandas
CSV to JSON array
Lets use simple CSV file example as below,
We shall read below file using Python and convert it to JSON array

import pandas as pd df = pd.read_csv ("Input File path") df.to_json ("Out File path')
Thats all , above 3 liners are what you need to convert a CSV to json file.
Lets run the above logic and produce a result,
{
"FirstName": {
"0": "TEST1",
"1": "TEST2",
"2": "TEST3",
"3": "TEST4"
},
"LastName": {
"0": "Test2",
"1": "Test3",
"2": "Test4",
"3": "Test5"
},
"Address": {
"0": "USA",
"1": "USA",
"2": "USA",
"3": "USA"
}
}
You can pretty much control the output format using other overloaded methods using parameters like orient=’records’ as below example.
Sample Example
import pandas as pd df = pd.read_csv (r'C:\Users\source\repos\PythonApplication1\Address.csv') df.to_json (r'C:\Users\source\repos\PythonApplication1\Address.json', orient='records')
Output JSON for the above example as below,
[
{
"FirstName": "TEST1",
"LastName": "Test2",
"Address": "USA"
},
{
"FirstName": "TEST2",
"LastName": "Test3",
"Address": "USA"
},
{
"FirstName": "TEST3",
"LastName": "Test4",
"Address": "USA"
},
{
"FirstName": "TEST4",
"LastName": "Test5",
"Address": "USA"
}
]
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.