Bind SQL Data to Windows Forms DataGridView using Entity Framework .NET Core
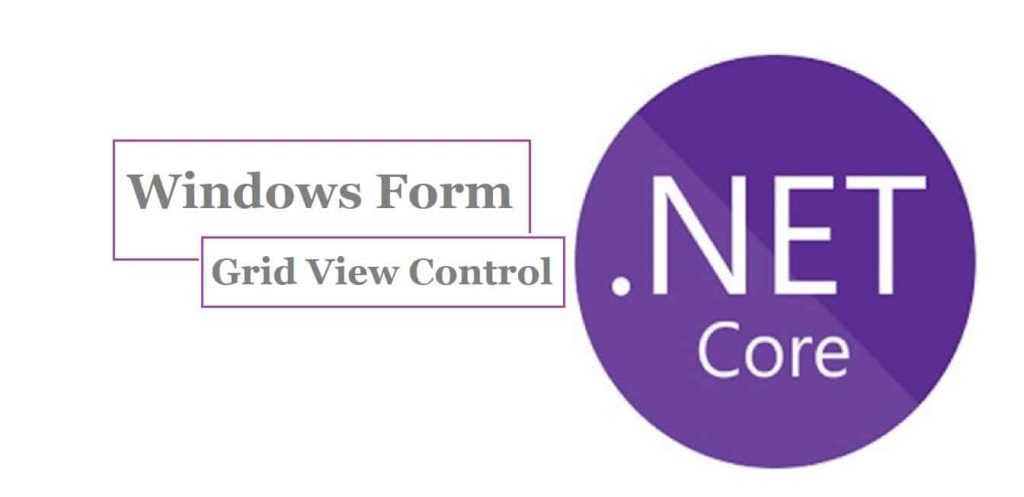
In this article, we will see how to Bind SQL to Windows Forms DataGridView Control in .NET Core-based Windows form application.
We shall use the Entity Framework for connecting to the database.
Today in this article, we will cover below aspects,
I recommend you to go through my previous article for understanding how to use Entity Framework in Windows form app.
We shall be using the .NET Core Windows form application project template to create the application. We shall see two approaches of using and without using DI(Dependency injection) the Entity framework DBContext.
.NET Core 3.1 has provided all the required support to create UI with the help of a Windows form designer tool kit that comes built-in within Visual Studio 2019 IDE and onwards only.
Getting Started
Here I am using the .NET Core Windows Form application,

Let’s add the Button and Grid view control to the Form. Grid View control can be added updates form.designer.cs as below,

Our Form class looks as below,
Using EmployeeContext using DI in Form class
EmployeeContext can be initialized within the master Form class using Constructor injections. We shall be doing DI using generic HostBuilder for EmployeeCotext class.
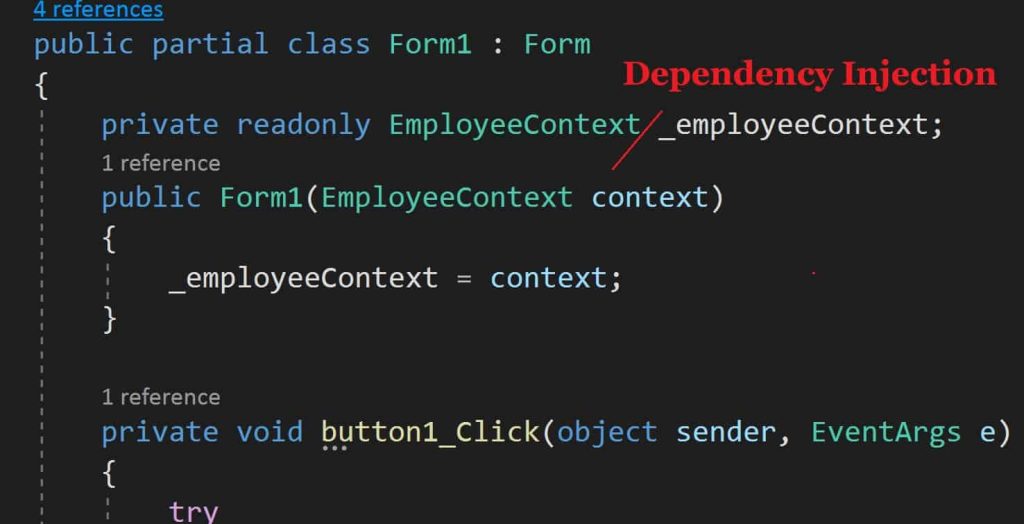
To understand Configuring DBContext in Windows Form, please refer to the below article for complete details.
Please add the below code to the Button Click event to get data from the SQL database and bind it to the Grid View.
We shall be adding sample data as below,
private void LoadEmployee_Click(object sender, EventArgs e)
{
try
{
dataGridEmployee.DataSource = _employeeContext.Employee.ToList(); ;
}
catch (Exception ex)
{
//Log technical exception
MessageBox.Show(ex.Message);
//Return exception repsponse here
throw;
}
}
In the above code _employeeContext.Employee.ToList() will give us all the Employee details from SQL tables.
EmployeeContext class was Scaffolded using EFCore DBContext commands.
EmployeeContext class needs to register in the IoC container as below,
///Generate Host Builder and Register the Services for DI
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddDbContext<EmployeeContext>(options =>
{
options.UseSqlServer("Server=localhost\\SQLEXPRESS;Database=master;Trusted_Connection=True;");
});
});
var host = builder.Build();
For complete code on configuring DBContext in the Windows Form app, please refer to the below article,
Using DBContext without Dependency Injection
If you do not want to use DI, it is pretty straightforward to use DBContext anywhere in the project. In fact, DBContext becomes accessible in your classes by adding ‘using’ statements and creating its instance.
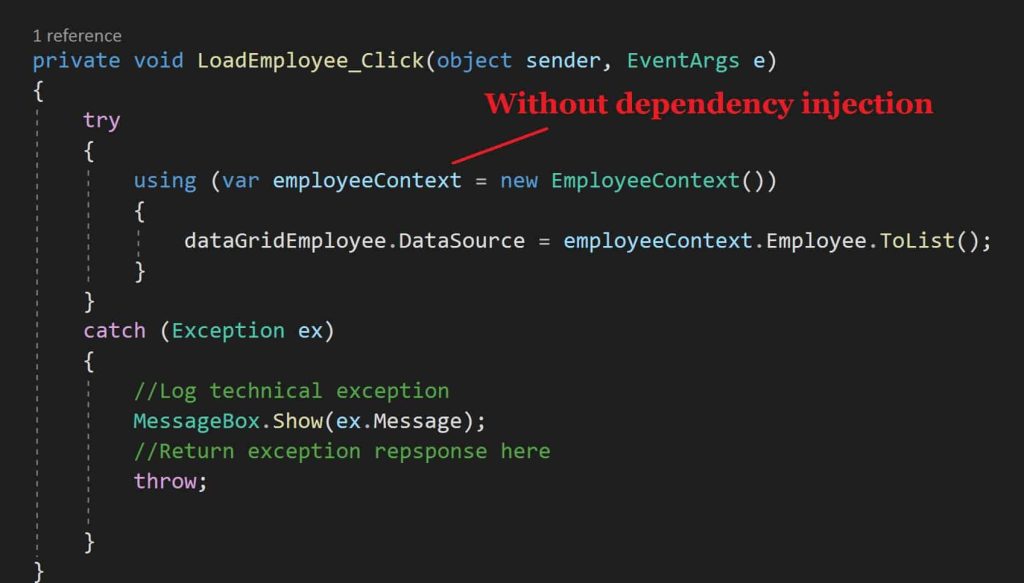
Form.Designer.cs code base should initialize the DataGridView using the below,
private DataGridView dataGridEmployee;
Please define any additional properties for Gride View as required
this.dataGridEmployee.Dock = DockStyle.Bottom;
Finally, let’s launch the application and check the result by clicking ‘LoadEmployee Data’
Data loading and binding will be successful!
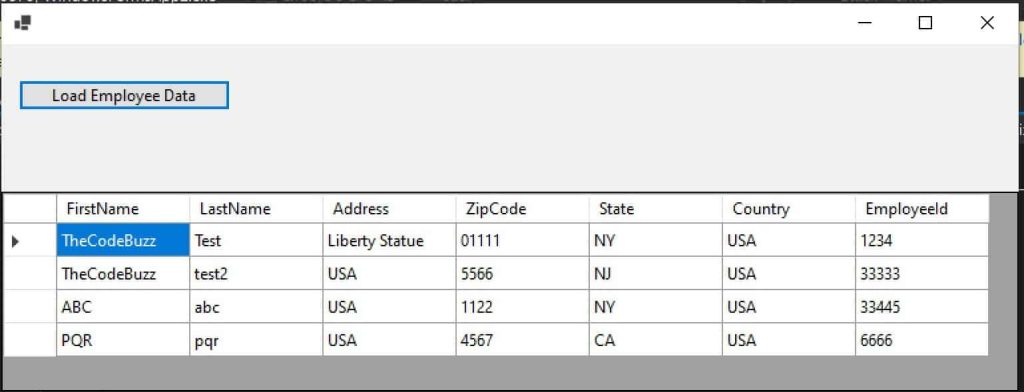
You are all set!
Reference: DI(Dependency Injection) in the Windows Form application
That’s All !! Happy Coding!
Do you see any improvement to the above code ?. Please let me know your suggestion if any.
Summary
In this article, we looked at how to Bind Data to the Windows Forms DataGridView Control in .NET Core. We used the Entity Framework to connect to the database and bind the data to the Grid View Control. You get pretty much the same feel of development using Windows forms in .NET Core with the added advantage of performance, lightweight, etc.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.