Consuming ASMX Web Services in ASP.NET Core
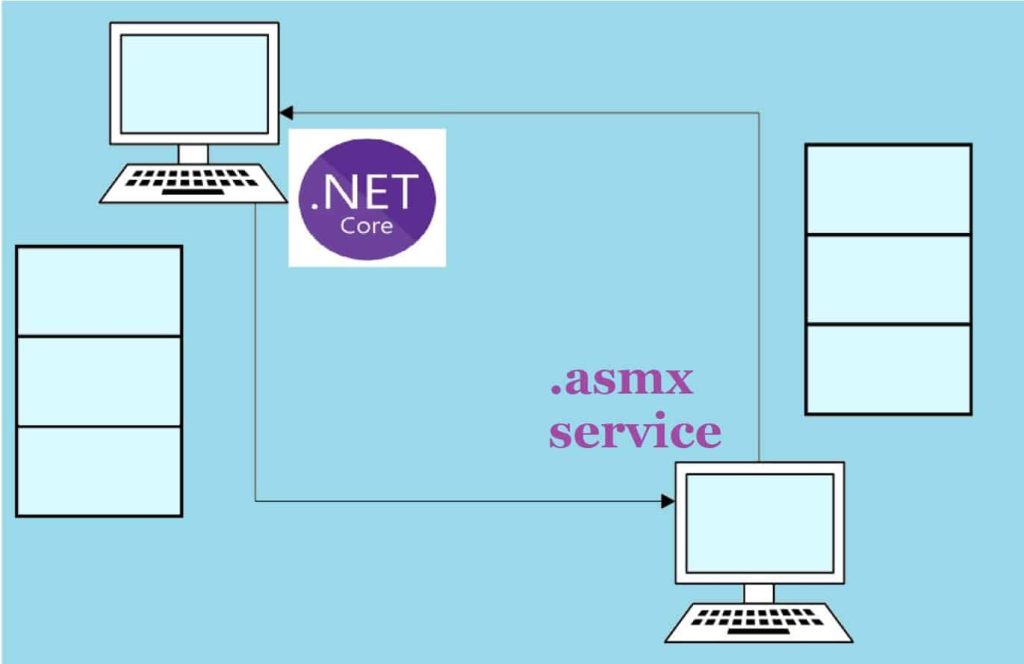
Today in this article on best practices, we shall learn how to consume ASMX Web services easily in ASP.NET Core-based applications.
This technique can be used for any type of ASP.NET Core application like WebAPI or ASP.NET Core Console or batch applications etc.
Today in this article, we will cover below aspects,
If interested in knowing more about how to consume WCF services in the .NET Core application, please see visit article Consuming WCF Web Services in .NET Core – Best Practices.
Getting Started
To get started, you need an ASMX service. I already have a sample .asmx service with contract details as below.
We shall consume the below ASMX service within the C# .NET Core Console Application.
However, you can use the below technique for other types of applications as well like .NET Core API or Form application, ASP.NET Core MVC based on .NET Core 3.1 or .NET 5, etc.
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
public class WebService1 : System.Web.Services.WebService
{
[WebMethod]
public int Add(int a, int b)
{
return (a + b);
}
[WebMethod]
public Single Subtract(Single A, Single B)
{
return (A - B);
}
[WebMethod]
public Single Multiply(Single A, Single B)
{
return A * B;
}
[WebMethod]
public System.Single Divide(Single A, Single B)
{
if (B == 0)
return -1;
return Convert.ToSingle(A / B);
}
}
}
To consume or call the ASMX service, a better technique is to use a proxy client.
Let’s create the proxy for the above-given .asmx service.
Creating Client Proxy Using Connected Services
Client-side can be easily created using Visual Studio’s “Connected Services” feature.
Connected services let you create client-side code easily using direct Service .asmx URI (If available) or using the WSDL file (If shared by the vendor or third party).
I have discussed both approaches in detail which are based on ‘Connected Services’ using WSDL and URI in the below article.
Within your project references, please right-click on the “Dependencies” and Click on the “Add Connected Service”.
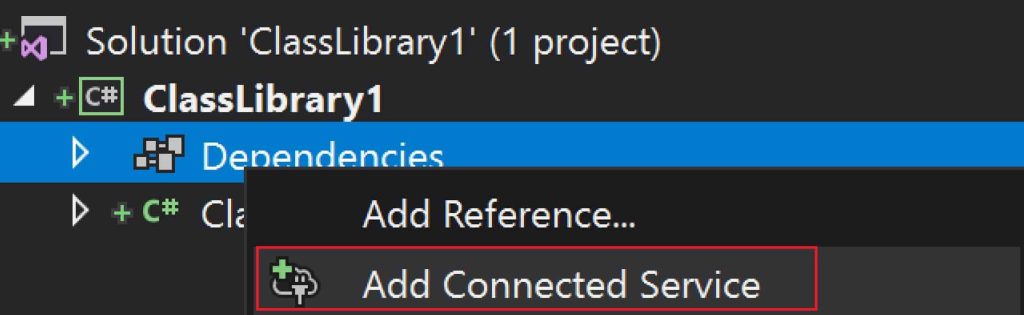
The above option shall create a client proxy class that will help us to invoke a method on the service.
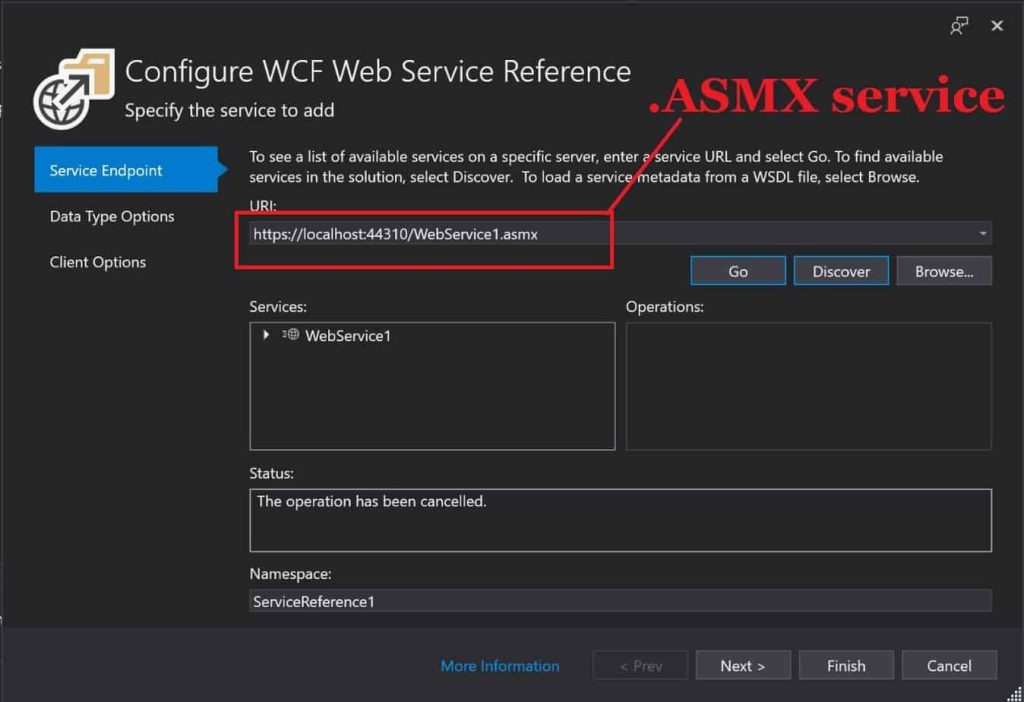
The generated client proxy class WebService1SoapClient definition will look as below. (The file is trimmed as below).
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.CodeDom.Compiler.GeneratedCodeAttribute("Microsoft.Tools.ServiceModel.Svcutil", "2.0.1")]
public partial class WebService1SoapClient : System.ServiceModel.ClientBase<ServiceReference1.WebService1Soap>,
ServiceReference1.WebService1Soap
{
static partial void ConfigureEndpoint(System.ServiceModel.Description.ServiceEndpoint serviceEndpoint, System.ServiceModel.Description.ClientCredentials clientCredentials);
public WebService1SoapClient(EndpointConfiguration endpointConfiguration) :
base(WebService1SoapClient.GetBindingForEndpoint(endpointConfiguration), WebService1SoapClient.GetEndpointAddress(endpointConfiguration))
{
this.Endpoint.Name = endpointConfiguration.ToString();
ConfigureEndpoint(this.Endpoint, this.ClientCredentials);
}
..
..
}
Create Proxy using WSDL file
Kindly refer to the WSDL section to generate the proxy in the below article.
Create Proxy using svcutil tools
If Visual Studio ‘Connected Service’ is not feasible for you, you can create proxy-client code using ‘svcutil’ tool easily.
This tool is available through NuGet packages. For more details please see the below article,
Client-Side code
namespace asmxclient { class Program { static async Task Main(string[] args) { WebService1SoapClient client = new WebService1SoapClient(EndpointConfiguration.WebService1Soap); var result = await client.AddAsync(5, 8); } } }
That’s all! The above code will give us the expected results.
Note: You may get some issues or challenges while creating a proxy for old legacy code. If the generated proxy code is not compatible, please try trial and error and you may want to update the generated proxy.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References :
- Consuming WCF Web Services in .NET Core – Best Practices
- Consuming WCF Web Services in .NET Core using Global Tool
Summary
Today in this article we learned how to consume ASMX Web services easily in an ASP.NET Core-based application. We looked at the “Connected Services” and dotnet-svcutil tools to create two techniques that help us create proxy scaffolding for any ASMX service. This technique can be used for any type of ASP.NET Core application like ASP.NET Core API Console apps or Desktop applications.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Hi!
How can i call a asmx service that has windows auth?
how can we keep open state for second call ?
Hello Abhilash- You can create a singleton instance of proxy service object and reuse it as needed. Please return for more information if I misunderstood your questions.
How did you create the .asmx service?