Create JWT Token in ASP.NET Core Step by Step
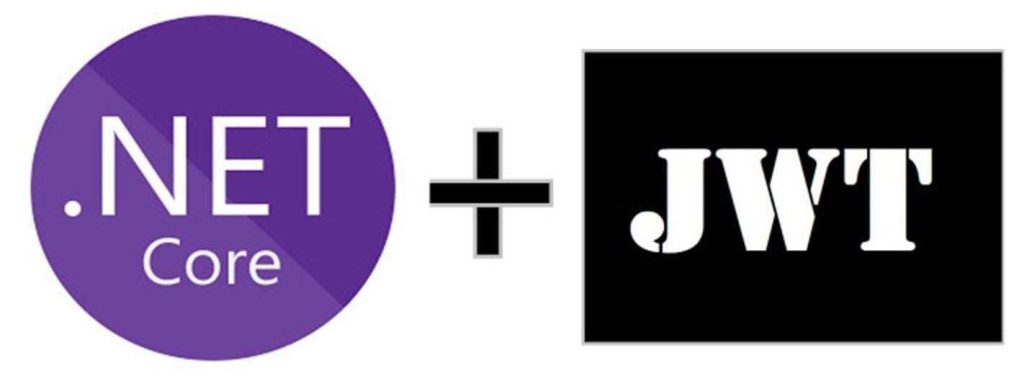
Today in this article we will learn how to generate/Create JWT Token in ASP.NET Core 3.1 or 6.0 using simple easy to understand examples.
In our last article, we already learned, how to add these tokens into the API pipeline and consume it to secure our API. If interested please refer below article on the same,
JSON Web Token( JWT) is an open standard used for securely transmitting information between parties as a JSON object.
JSON Web Tokens are very useful for various scenarios like authorization purposes or Information exchange using digitally signed key-value pairs.
Getting started
We shall be creating a simple .NET Core service that will create a JWT token programmatically for a given set of credentials.
However, you can use any project type as needed.
Create ASP.NET Core 6 project,

Build the JWT Token
We shall be making use of class JwtSecurityToken for initializing new instances of token-based in parameters like Symmterickey, credentials, expiry, etc.
Here I shall be making use above class within a .NET Core Controller so that we are able to expose an API endpoint for returning newly generated JWT tokens to the user.
Let’s define the configuration properties to be used for generating the JWT token.
Here below is an example of a configuration we shall use to generate the JWT.
"JwtToken": { "SecretKey": "SecretKeywqewqeqqqqqqqqqqqweeeeeeeeeeeeeeeeeeeqweqe", "Issuer": "https://localhost:44341", "Audience": "https://localhost:44341", "TokenExpiry": "60" //minutes },
As above I have defined the properties to be used in class JwtSecurityToken.
- SecretKey– SigningCredentials with Secured algorithm and key that signs the security token.
- Issuer – A valid issuer that will be used to check against the token’s issuer.
- Audience – A valid audience that will be used to check against the token’s audience.
- TokenExpiry– Define the lifetime expiry of the token once issued from the current date and timestamp.
Note: The secret key length should be a minimum of 128 bits i.e (16 bytes)
Below is the basic implementation of the custom BuildToken() method.
private string BuildJWTToken() { var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_config["JwtToken:SecretKey"])); var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256); var issuer = _config["JwtToken:Issuer"]; var audience = _config["JwtToken:Audience"]; var jwtValidity = DateTime.Now.AddMinutes(Convert.ToDouble(_config["JwtToken:TokenExpiry"])); var token = new JwtSecurityToken(issuer, audience, expires: jwtValidity, signingCredentials: creds); return new JwtSecurityTokenHandler().WriteToken(token); }
The above generic method can be used in any type of application like batch, or console, etc if needed.
Lets now complete our Controller method using the above logic.
In the below implementation, I have added additional security on the Controller method to validate the user credentials requesting the new JWT token.
I have used the POST method so that login credentials can be sent before issuing the JWT token.
[AllowAnonymous] [HttpPost] public IActionResult CreateToken([FromBody]LoginModel login) { if (login == null) return Unauthorized(); string tokenString = string.Empty; bool validUser = Authenticate(login); if (validUser) { tokenString = BuildJWTToken(); } else { return Unauthorized(); } return Ok(new { Token = tokenString }); }
Below is the method to validate the user requesting a new JWT token,
private bool Authenticate(LoginModel login) { bool validUser = false; if (login.Username == _config["AuthLogin:UserName"] && login.Password ==_config["AuthLogin:Password"]) { validUser = true; } return validUser; }
LoginModel class is defined as below,
public class LoginModel { public string Username { get; set; } public string Password { get; set; } }
I am using SOAP UI to invoke the POST method to create a JWT token,

The generated JWT token is as below,
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9. eyJleHAiOjE1NzEwMDc3MzQsImlzcyI6Imh0dHBzOi8vbG9jYWxob3N0OjQ0MzQxIiwiYXVkIjoiaHR0cHM6Ly9sb2NhbGhvc3Q6NDQzNDEifQ. Ve2x6ueiHPyrP7wLjpmZrhx7QWrQKnkxWsu_1qUKKLA
Now you can use the above token to secure your API to validate the authentication and see if it is working fine.
I have talked about how to consume JWT tokens in ASP.NET Core API in the below article.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Security is an integral part of any application development and today in this article we understood how to create/generate JSON Web Token (JWT) token to secure .NET Core applications like WebAPI or other types in a few simple steps. We also exposed this functionally as an API/Service so that it can be leveraged by other APIs or Applications.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.