Swagger OAuth2 Authorize in ASP.NET Core

Today in this article, we shall discuss, how to enable Swagger OAuth2 Authorize in ASP.NET Core. We will use Swagger (Open API) documentation with .NET 6 using IOperationFilter.
If the Oauth2 Security scheme needs to be applied globally, we already looked at it in our previous article.
There are a few differences and design updates in OpenAPI or Swagger 3.0.
ASP.NET Core 3.1 onwards OpenAPI v3.0 document specifications are followed. Swashbuckle tools help us follow these specifications and generate nice documentation for the API.
If you have security schemes that you only want to apply to specific operations, then using IOpertionFilter makes it simple to configure.
IOperationFilter also lets you define multiple security schemes if needed.
Getting Started
Create ASP.NET Core 3.1 or .NET 6 API
Please install below Nuget package,
PM> Install-Package Swashbuckle.AspNetCore -Version <>
This single NuGet package shall add all other required components as shown below and you need not have to add them explicitly,
- Swagger
- SwaggerUI
- SwaggerGen
Update ConfigureServices() method
Please add below AddSecurityDefinition() methods to your swagger middleware.
We need to use the SecurityScheme type as “OAuth2” and define OpenApiOAuthFlows as shown in the below implementation.
AddSecurityDefinition() methods let you define your API security by defining one or more security schemes like OAuth2 or JWT Bearer Scheme or Basic Authentication scheme.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "TheCodeBuzzService", Version = "v1" });
c.AddSecurityDefinition("oauth2", new OpenApiSecurityScheme
{
Type = SecuritySchemeType.OAuth2,
Flows = new OpenApiOAuthFlows
{
Implicit = new OpenApiOAuthFlow
{
AuthorizationUrl = new Uri("https://localhost:44341/oauth"),
//TokenUrl = new Uri("your-auth-url"),
Scopes = new Dictionary<string, string>
{
{ "readAccess", "Access read operations" },
{ "writeAccess", "Access write operations" }
}
}
}
});
c.OperationFilter<OAuth2OperationFilter>();
var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml";
var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile);
c.IncludeXmlComments(xmlPath);
});
}
OAuth2 Swagger – Implement IOperationFilter interface
Above we have used class AuthOperationFilter which is implemented for the OAuth2 security scheme using the IOperationFilter.
Here class AuthOperationFilter is derived from IOperationFilter and we have to override Apply (..) method as below.
public class OAuth2OperationFilter : IOperationFilter
{
public void Apply(OpenApiOperation operation, OperationFilterContext context)
{
var isAuthorized = context.MethodInfo.DeclaringType.GetCustomAttributes(true).OfType<AuthorizeAttribute>().Any() ||
context.MethodInfo.GetCustomAttributes(true).OfType<AuthorizeAttribute>().Any();
if (!isAuthorized) return;
operation.Responses.TryAdd("401", new OpenApiResponse { Description = "Unauthorized" });
operation.Responses.TryAdd("403", new OpenApiResponse { Description = "Forbidden" });
var oauth2SecurityScheme = new OpenApiSecurityScheme()
{
Reference = new OpenApiReference { Type = ReferenceType.SecurityScheme, Id = "oauth2" },
};
operation.Security.Add(new OpenApiSecurityRequirement()
{
[oauth2SecurityScheme] = new[] { "thecodebuzz" } //'thecodebuzz' is scope here
});
}
}
Please, note that we can retrieve API descriptions for relevant information like attribute, route information, etc. using the IOperationFilter interface.
This Interface also lets you define and apply schemes to specific operations.
Please update the Configure() method as below,
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "TestService");
c.OAuthClientId("cleint-id");
c.OAuthClientSecret("client-secret");
c.OAuthRealm("client-realm");
c.OAuthAppName("OAuth-app");
});
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
The generated swagger definition will have the ‘Authorize’ button.
Based on OperationFilter logic this lock icon will be displayed only for specified APIs where criteria match.
Example– In the below example, we are validating the “Authorize” attribute for an operation to apply a given security scheme.
Our WebAPI has two GET methods and only one is secured with the [Authorize] attribute.

Please click on the Authorize Button and provide OAuth2 credentials.
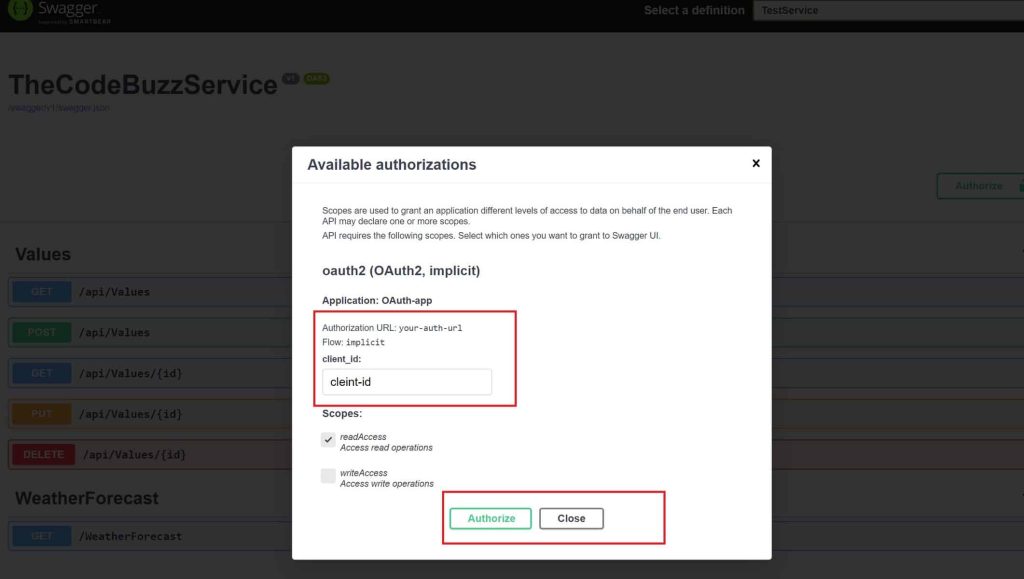
That’s all, you are all set to use Swagger with an OAuth2 authorization token using OperationFilter.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
In this post, we learned how to add OAuth2 Authentication to Swagger (OpenAPI) documentation in the ASP.NET Core application using OperationFilter techniques.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.