Expose WCF web services as REST API in 5 minutes
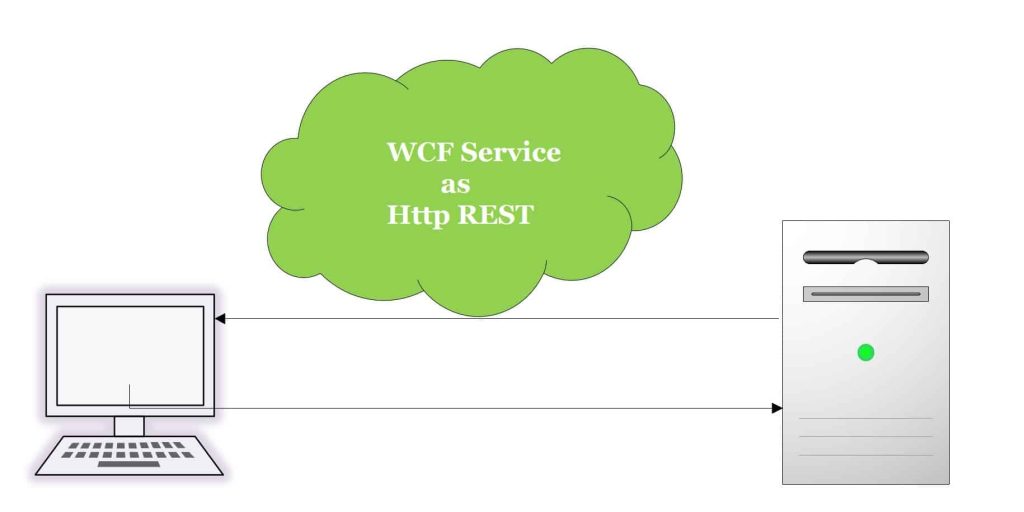
In this article, we will see a simple approach to Enable WCF web services as REST API.
These could be legitimate use cases for most of you where you would like to expose the existing WCF service as REST without breaking any existing client which might be using SOAP, a TCP way of communication with the service.
This approach, however, can be considered a temporary resolution or workaround.
WCF(Windows communication foundation) was a rich SOA framework(Until the last decade). It allowed us to create enterprise SOA(service-oriented architecture) applications easily.
However recent popularity of Restful API in the direction of Microservices architecture and adoption has overtaken WCF as a favorite framework for Service/API development.
Today in this article, we will cover below aspects,
For any organization, 70% and above portfolios still rely on legacy services. That means Old is still gold – at least in the value proposition.
The legacy business value is still ahead of any new technology stack initiative and it would take maybe another decade to overcome any legacy existing framework. And we don’t know if even today’s green technology could be obsolete in few years down the line.
Don’t Break existing Clients
Most of you you must be either trying to migrate all your WCF services to either RESTFull .NET Core API/ services Or trying to create a wrapper around existing legacy services.
In this article, we shall be leveraging the WCF framework’s ability to expose multiple endpoints for a given contract.
So that means if you already have a client using SOAP endpoint or NET TCP endpoint they will remain unaffected.
WCF Services Support Multiple Endpoints?
WCF Services Support Multiple Endpoints which is a built-in framework feature.
One can expose the same contract or multiple contracts on the same or multiple endpoints and it is pretty simple to do so.
Today I am going to talk about an approach of enabling WCF service as REST API without breaking any existing client.
Getting started
I already have a sample WCF service that exposes a contract with simple API GetEmployee

Step1 – Convert WCF Contract as REST API
I now will be trying to decorate the existing WCF contract to act like a REST API interface as below,
Please decorate your method with WebInvoke annotation as below,
[WebInvoke(Method = "GET", UriTemplate = "Employee/{empId}",
RequestFormat = WebMessageFormat.Json,
ResponseFormat = WebMessageFormat.Json)]

WebInvoke can also be defined for POST, DELETE or PUT methods as required.
RequestFormat can also be used to specify XML as a message format if needed.
[WebInvoke(Method = "GET", UriTemplate = "Employee/{empId}",
RequestFormat = WebMessageFormat.Json,
ResponseFormat = WebMessageFormat.Json)]
Step2 – Configure REST endpoint and Behaviour
The Web.config file needs to register for additional endpoints for REST. Each endpoint will be supporting the same contract IEmployeeService at different addresses using different bindings. The base address can remain the same.

<services>
<service name="WcfEmployeeService.EmployeeService">
<!--Base address for the host-->
<endpoint binding="basicHttpBinding" contract="WcfEmployeeService.IEmployeeService"/>
<!--Secured call endpoint-->
<endpoint address="authorize" binding="wsHttpBinding" contract="WcfEmployeeService.IEmployeeService"/>
<!--RESTFul endpoint-->
<endpoint address="api" binding="webHttpBinding" contract="WcfEmployeeService.IEmployeeService"
behaviorConfiguration="jsonBehaviour"/>
</service>
</services>
In the above configuration, we have defined three endpoints. One of the endpoints is RestFul which we added recently.
The behavior is defined as below,
<endpointBehaviors>
<behavior name ="jsonBehaviour">
<webHttp automaticFormatSelectionEnabled="true"/>
</behavior>
</endpointBehaviors>
Finally, Execute the API using the normal proxy way, and using HttpClient, you shall see the same results.
Using Proxy client
EmployeeServiceClient client = new EmployeeServiceClient(); var details = await client.GetEmployeeDataAsync(id);

Using HttpClient
private static HttpClient _client = new HttpClient();
.
.
[HttpGet("{employeeId}")]
public async Task<IActionResult> Get(string employeeId)
{
var uri = new Uri($"{ServiceUrl}/{employeeId}");
var response = await _client.GetAsync(uri);
if (response.IsSuccessStatusCode)
{
return Ok(response.Content.ReadAsStringAsync().Result);
}
else
{
return StatusCode(500, "Server error");
}
}

The above service URL should also match the JSON route.
I have used the below route for HTTP calls API :
ServiceUrl = “http://localhost:65524/Service1.svc/api/Employee”;
.NET Core Client support?
You can very much use the .NET Core client. In fact above client application, I have used is the ASP.NET Core API application.
Other useful references,
That’s All! Happy Coding!!
Summary
It is possible to enable and expose WCF service as REST API without breaking the existing clients. WCF allows you to expose multiple endpoints and we can very much leverage that concept. This approach can be a handy workaround in many scenarios.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Thanks a lot for sharing. Saved me at least 10% of what few brain cells I have left. 🙂
Hello Allen- Thanks . Glad it helped you !
Very Helpful. Thank you!
Thanks Andy. Glad it helped you!
Great ! You saved me many dollars !!
Awesome! Thanks James. Glad it helped you..