IOException: The process cannot access the file ‘file path’ because it is being used by another process
Issue Description
Today in this article, we will cover below aspects,
While performing an operation on file produces an error as below,
IOException:The process cannot access the file 'file' because it is being used by another process.
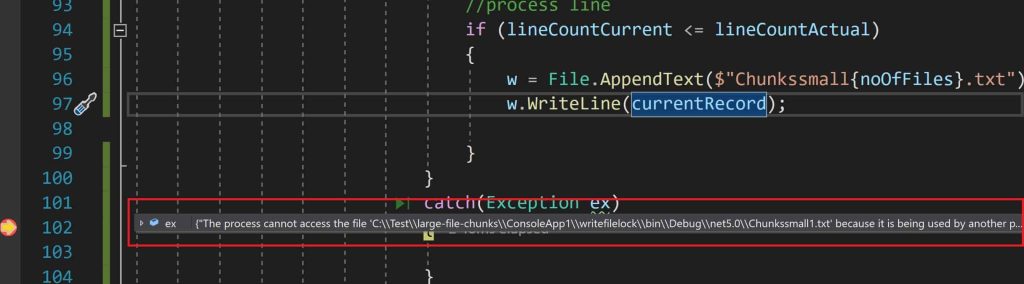
Resolution
This error means, the file which you are trying to access is not accessible because,
- The file may be in use by some other process.
- The file is open by the Own process (maybe due to coding issues).
- The file property is set to not share while reading or writing the file.
This issue could occure when perfroming any operations like
– Read
– Write
– Delete
This issue can be resolved by carefully following all possible approaches based on how your application is dealing with files.
Solution 1 –The file may be in use by some other process
- Please make sure to verify the file is not opened by another process.
This could be any specific process on the system or program opening the file at the time when your program or application is already using it.
Its important how different methods are being used in the code. There are multiple ways to deal with File Open, Create, Read, or write operations.
A few examples of File related methods but not limited are as below,
File.Create
File.Open
File.ReadAllText()
File.WriteAllText()
File.ReadAllLines()
File.WriteAllLines()
Example
// Create the file, or overwrite if the file exists.
using (FileStream fs = File.Create(path))
{
byte[] info = new UTF8Encoding(true).GetBytes("This is test file.");
// Add details to the file.
fs.Write(info, 0, info.Length);
}
Note: When using File.Create, please note FileShare value of None is used as default behavior i.e no other process or code can access the file until the original file handle is closed.
Understand File Enumeration to Solve File access issues,
While using FileStream class, you get the ability to specify the FileShare access type another process can have while dealing with File Processing. FileStream class supports the below types,
- FileShare.ReadWrite
- FileShare.Write
- FileShare.Read
- FileShare.None
For more information and best practices, please visit the below article.
Proper use of FileShare enumeration will help you resolve the most common issues while dealing with files.
Solution 2 – Implements IDisposable for Files handles
It’s always safe to use the “using” statement while dealing with files instead of creating and managing the instance ourselves.
Wrap all your code inside “Using” statements.

For more information and best practices, please visit the below article.
Solution 3 – Implement File Lock, Unlock – Thread Synchronization
- The file can be accessed by multiple threads within the application or by external process threads.
- If you have multiple threads attempting to access the same file, consider using a Synchronization mechanism using Lock or another threading synchronization mechanism.
- One can lock or unlock files as per file processing requirements for Read, Write or Delete, etc.
For more information and best practices, please visit the below article.
Solution 4 – Implement a Retry pattern for File processing
This is not mandatory but always come in handy while dealing with file exceptions or temporary glitch accessing the file.
For more information and best practices, please visit the below article.
References:
For more information and best practices, please visit the below article.
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.