FTP SSH.NET – Move or Archive Files with examples
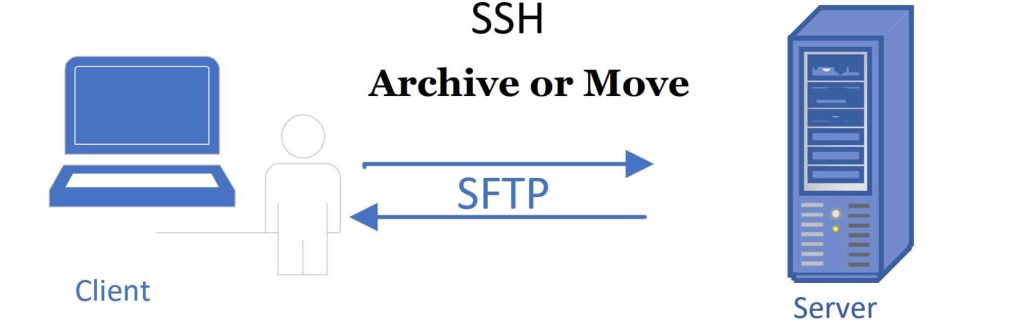
Today in this article, we will see how to perform FTP SSH.NET – Move or Archive Files with examples using C#.NET code.
We will explore this option using the SSH.NET library for .NET/.NET Core.
What is SFTP
SFTP (SSH File Transfer Protocol) is a secure file transfer protocol. It runs over the SSH protocol.
It supports the full security and authentication functionality of SSH.
Below is the list of high-level features supported by the SSH library (but not limited to),
- Provide SFTP functionality for both synchronous and asynchronous operations.
- Provides SCP functionality
- Execution of SSH command using both synchronous and asynchronous methods
- Provide a status report for upload and download SFTP operations to allow accurate progress bar implementation.
- Supports public-key, password, and keyboard-interactive authentication methods.
- Supports two-factor or higher authentication
- Supports SOCKS4, SOCKS5, and HTTP Proxy
Getting access to all the files using the SSH.NET library is pretty simple.
Getting Started
Create any .NET Core application like a console application,
Add SSH.NET Nuget package as below,

Package Manager Console,
Install-Package SSH.NET -Version 2020.0.2
Note: Please use the latest version.
Connect to SFTP using SFTPClient
SSH.NET provides SFTPClient class that lets you connect to the SFTP server securely and access files for
read, write, upload, or download purposes,
Below the sample code read the FTP file and write their name on the console.
using (var client = new SftpClient("****", "user", "****"))
{
client.Connect();
ListDirectory(client, ".");
foreach (var file in files)
{
Console.WriteLine(file.FullName);
}
}
The above ListDirectory uses the default path to fetch a file from the SFTP server.
File Move Archive using FTP
File move or archive file from a remote file path is pretty simple once the connection is established successfully.
Use the below code to Move or Archive the file via SftpClient
using (var client = new SftpClient("****", "user", "****"))
{
client.Connect();
ListDirectory(client, ".");
foreach (var file in files)
{
Console.WriteLine(file.FullName);
var fileMove = client.Get(file.FullName);
fileMove.MoveTo(destinationPath);
}
client.Disconnect();
}
In the above code,
destinationPath – This is the file path where the file will be moved via SFTP/FTP.
Get method gives a reference of the file from the remote location by returning Renci.SshNet.Sftp.SftpFile file object.
MoveTo– Moves a specified file to a new location on a remote machine. You can use the same file name or provide a name of your choice.
Copy OR Move File using SSH.NET using DownloadFile or Rename
One can easily use the DownloadFile or Rename method to copy or archive the files as explained below in the code.
using (var client = new SftpClient(host, username, password)) { client.Connect(); using (var fileStream = System.IO.File.Create(localDestinationPath)) { client.DownloadFile(remoteFilePath, fileStream); } client.Disconnect(); }
OR
using (var client = new SftpClient(host, username, password)) { client.Connect(); // Rename the file to move it client.RenameFile(sourceFilePath, destinationFilePath); client.Disconnect(); }
FTP how to get a list of all files in a Path to Move?
Below is the complete implementation for the ListDirectory() custom method which returns all the files available from the specified root folder and subfolders.

Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.