
Today in this article, we shall learn how to enable Header/Media API versioning in .NET.
In this type of versioning technique, you add a version number to the URI for each resource using the accept header.
Existing URIs continue to operate as per contract, returning resources that conform to the original schema.
Using Content negotiation REST API allows accepting the request in any format like JSON or XML.
Currently, the parameters used in media types for content negotiation can be content in detail to derive the API versioning on the server side.
Today in this article, we will cover below aspects,
As we understood in our last article Change in API is inevitable and API versioning comes to the rescue to protect your API from any breaking changes or changes in resources for any new requirements more importantly without breaking any existing clients.
However, as I understood, this versioning works with limited features only in the ASP.NET Core.
For more details on other API versioning techniques, please please visit the below article,
The default versioning feature works for standard formats like below few examples.
Example,
accept: text/plain;v=1.0
accept: application/json;v=3.0
Enable Media/Accept Header versioning
Getting started
Let’s create ASP.NET Core API using ASP.NET Core 3.1 or .NET 5,
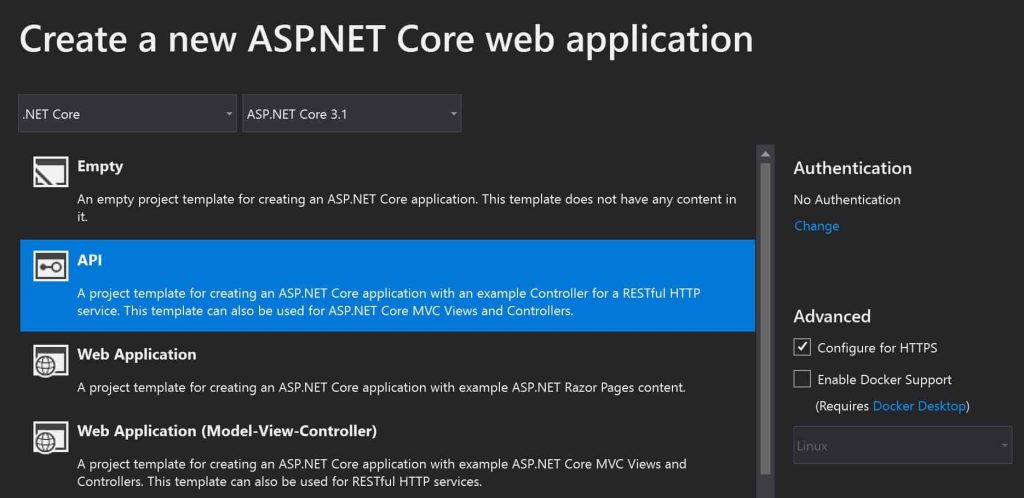
Please install below NuGet package,
PM> Install-Package Microsoft.AspNetCore.Mvc.Versioning -Version 4.1.1
Or
Install from NuGet Package Manager,
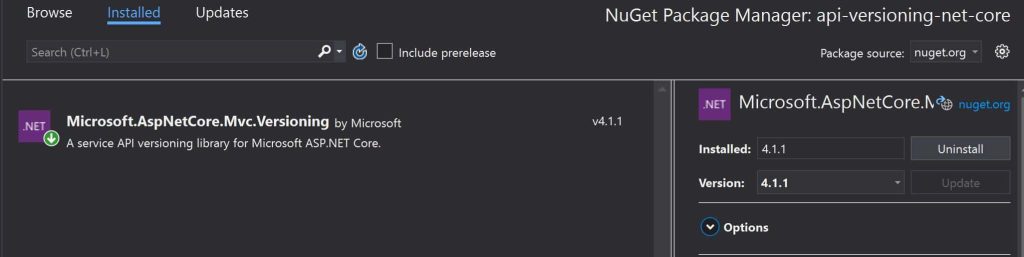
Please update the ConfigureServices method to add the MediaTypeApiVersionReader service to the service collection as below,
Step1
option.ApiVersionReader = new MediaTypeApiVersionReader("v");

Assign annotation [ApiVersion] at the Controller/Method level and then use any of the versioning techniques as enabled in the ConfigureServices method.
Step2
Here is the complete code,
[ApiController]
[Route("api/[controller]")]
[ApiVersion("1.0")]
[ApiVersion("2.0")]
[ApiVersion("3.0")]
public class WeatherForecastController : ControllerBase
{
private static readonly string[] Summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
private readonly ILogger<WeatherForecastController> _logger;
public WeatherForecastController(ILogger<WeatherForecastController> logger)
{
_logger = logger;
}
[HttpGet]
[MapToApiVersion("1.0")]
public IEnumerable<WeatherForecast> GetWatherUSA()
{
var rng = new Random();
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = rng.Next(-20, 55),
Summary = Summaries[rng.Next(Summaries.Length)]
})
.ToArray();
}
[HttpGet]
[MapToApiVersion("2.0")]
public IEnumerable<WeatherForecastNew> GetWeatherUK()
{
var rng = new Random();
return Enumerable.Range(1, 5).Select(index => new WeatherForecastNew
{
DateOfForcast = DateTime.Now.AddDays(index),
Temperature = rng.Next(-20, 55),
Summary = Summaries[rng.Next(Summaries.Length)]
})
.ToArray();
}
[HttpGet]
[MapToApiVersion("3.0")]
public IEnumerable<WeatherForecastIndia> GetWeatherIndia()
{
var rng = new Random();
return Enumerable.Range(1, 5).Select(index => new WeatherForecastIndia
{
ForcastDay = DateTime.Now.AddDays(index),
Temperature = rng.Next(-20, 55),
Summary = Summaries[rng.Next(Summaries.Length)]
})
.ToArray();
}
}
Let’s see v1 version execution,

So here V1.0 produces below JSON result,
{
"dateOfForcast": "2020-05-08T18:10:03.1268402-04:00",
"temperature": 22,
"temperatureFerehnite": 71,
"summary": "Bracing"
}
Let’s try executing the V2.0 version,
{
"date": "2020-05-08T18:11:00.1929376-04:00",
"temperatureC": 32,
"temperatureF": 89,
"summary": "Bracing"
}
References :
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.