How to log or pretty print Headers value requests or responses in .NET Core API
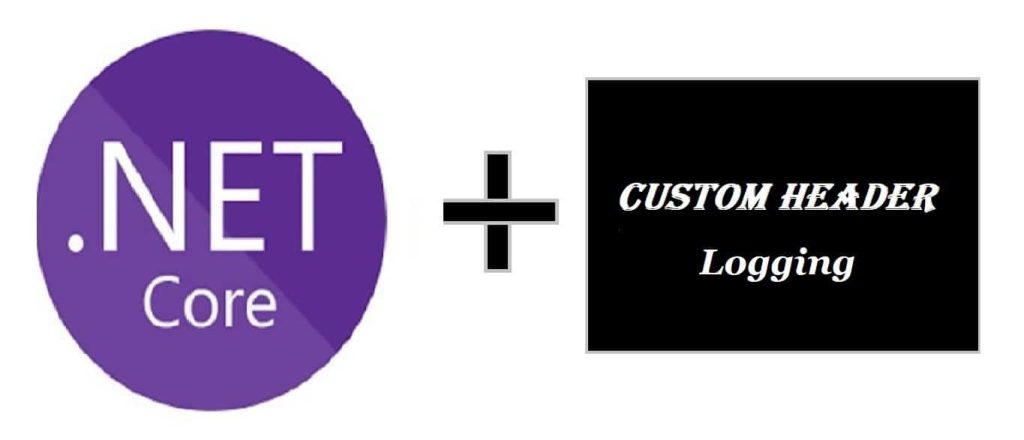
Today in this article, we shall see how to log headers in .NET Core requests or responses.
In your .NET Core API, you may find the need to read the custom header value programmatically for understanding various headers used in the API communication. You may pretty print headers to understand the details of all headers flowing incoming or outgoing with request or response.
You may want to enable logging when headers aren’t transmitted as expected.
You can enumerate the request headers received by the server if the logs don’t provide enough information to troubleshoot the problem.
For more details on how to enable basic custom middleware in .NET Core, please visit the below article,
Today in this article, we will see how to read HttpContext request headers using 2 below-discussed approaches,
Using Inline Middleware for logging custom headers
This is a very simple approach of using inline custom middleware for logging headers details.
Please Inject ILogger<Startup>
into the class as described below in detail.
Here default ILogger interface can be used easily in configure method by updating Configure method as below,
Example
public void Configure(IApplicationBuilder app, IWebHostEnvironment env,
ILogger<Startup> logger)
{
if (env.IsDevelopment())
{
logger.LogInformation("Log here");
app.UseDeveloperExceptionPage();
}
else
{
logger.LogInformation("Log here ");
app.UseExceptionHandler("/Error");
app.UseHsts();
}
,
,
,
,
}
Let’s now add inline middleware as below,
app.Use(async (context, next) =>
{
// Log/Print all Headers
foreach (var header in context.Request.Headers)
{
logger.LogInformation("Header: {Key}: {Value}", header.Key, header.Value);
}
logger.LogInformation("Request Method: {Method}", context.Request.Method);
logger.LogInformation("Request Scheme: {Scheme}", context.Request.Scheme);
logger.LogInformation("Request Path: {Path}", context.Request.Path);
await next();
});
If you are using UseForwardedHeaders in Startup.Configure
then make sure to use this middleware right after ForwardHeader middleware.
Using Custom middleware for logging Headers
Above discussed approach can be used as Custom middleware,
public async Task InvokeAsync(HttpContext context)
{
var _logger = _loggerFactory.CreateLogger<CustomMiddleware>();
try
{
// Log/Print all Headers
foreach (var header in context.Request.Headers)
{
logger.LogInformation("Header: {Key}: {Value}", header.Key, header.Value);
}
await _next(context);
}
catch (Exception ex)
{
_logger.LogError($"Something went wrong: {ex.Message}");
}
}
_logger instance can be injected as below,

Please see the below article on how to inject the ILogger interface into the custom middleware.
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.