How to Merge Multiple Files into one – C# .NET

Today in this article, we will see how to Merge multiple files into one using C# .NET and create a full file appended with content from all files.
We will cover below a few approaches to achieve file merge functionality.
This could be a legitimate need for many scenarios.
We will see a very generic approach that can be used for any file type like TEXT or DAT file etc.
We will see the basic approach and also use the FileStreams approach to deal with a stream of bytes to read and write the content.
Let’s get all files from a given Directory using the below code.
var inputFiles = Directory.GetFiles(@"C:\Test\Chunks");
We have a total of 10 files in the example path
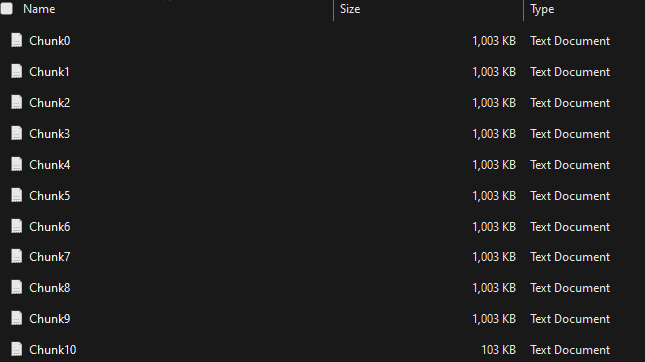
Approach 1 – Using File.ReadAlltext and File.AppendAllText
This approach is pretty simple and very handy if you are dealing with a limited size of files (maybe a few MBs).
var inputFiles = Directory.GetFiles(@"C:\Test\Chunks"); foreach (var file in inputFiles) { //Read all the lines from file var contents = File.ReadAllText(filePath); //Open file and Append to the end of file File.AppendAllText("FullFile.txt", contents, Encoding.Default); }
In the above approach, we are copying all the content of the file and append it to a new file.
Approach 2 – Using FileStreams and bytes
The below approach is also useful if you are dealing with a merge of big files (size in GBs) into a single file.
You may have bigger files containing GB of data like 1GB, 2GB, or more.
We will define chunk size so that we can operate on any big-size file in chunks.
It will let you process the specified amount of data at a time and lets you create a single file with combined data.
This approach also provides you with better control over file processing and access.
Example:
Chunk size is defined as 1 MB
const int chunkSize = 1024 * 1024; // 1MB
var inputFiles = Directory.GetFiles(@"C:\Test\Chunks"); using (var output = File.Create("FullFile.txt")) { foreach (var file in inputFiles) { using (var input = File.OpenRead(file)) { var buffer = new byte[chunkSize]; int bytesRead; while ((bytesRead = input.Read(buffer, 0, buffer.Length)) > 0) { output.Write(buffer, 0, bytesRead); } } } }
Above we are taking the highest care to read files with no sharing of file access while reading the content of the file.
File property i.e FileShare.None is useful to avoid accidental changes to input files while files is being read at the same time.
File property FileAccess.Read – provides the Read access to the file. Data can be read from the file.
Please see below the full file generated with a total of approximately 10.3 MB after the merge as below,

Please see below best practices while performing read and write on Files,
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.