ASP.NET Core Middleware Read Configuration settings
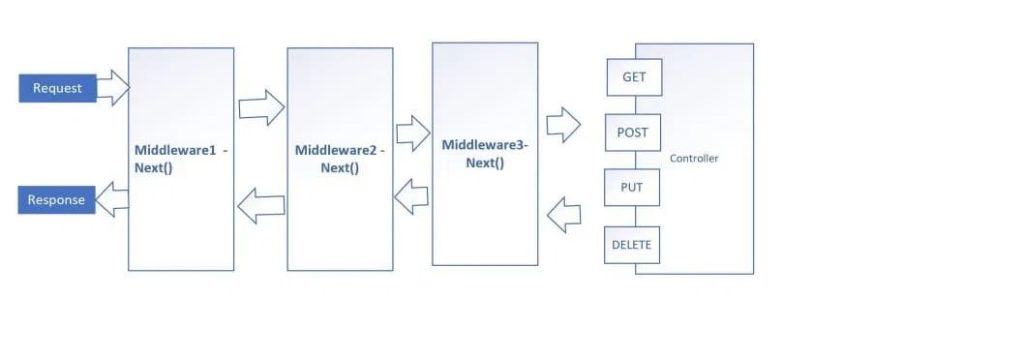
In this article, we will see how to access or Read Configuration in the Middleware component in the ASP.NET Core application.
Today in this article, we will cover below aspects,
As we know middleware is a component that is used in the application pipeline to handle requests and responses which can help perform pre and post-operation within the request and response API pipeline.
We shall use the approach of the middleware component for handling IConfiguration within the ASP.NET Core API pipeline.
Getting Started
Let’s create an ASP.NET Core 3.1 application.
Let’s create a very basic middleware component as below,
Create Middleware component
Lets create CustomMiddleware class as below,
Please visit the below article for more details on how to create middleware. In Visual Studio there is ready to use a template that creates the middleware component with just one click.
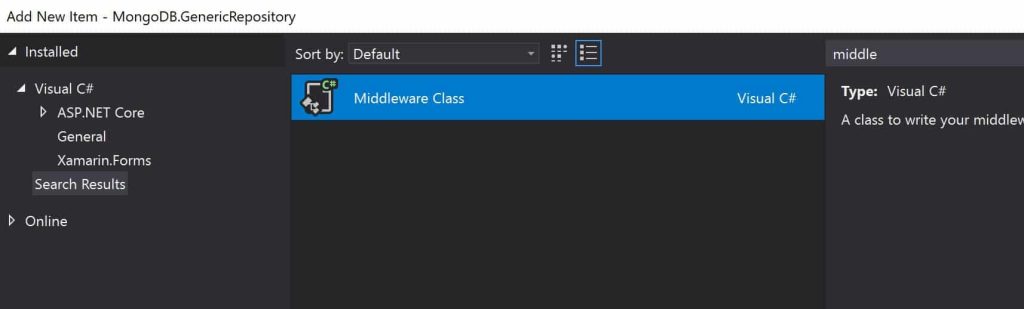
For more details: Add Custom Middleware in ASP.NET Core
One can use either the IOption interface or the IConfiguration interface to read the settings file.
Lets use below configuration file,
"CustomerConfig": {
"CustomerKeyurl": "http://customer/key",
"CustomerdetailsUrl": "http://customer/id",
"Agency": {
"AgencyID": "subvalue1_from_json",
"AccountKey": 200
}
}
Below is the generic custom middleware implementation.
public class CustomMiddleware
{
private readonly RequestDelegate _next;
public CustomMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext httpContext)
{
try
{
await _next(httpContext);
}
catch (Exception ex)
{
_logger.LogError($"Something went wrong: {ex.Message}");
}
}
}
Approach 1- Using IConfiguration to load the Config settings in Middleware
This is one of the simple ways of loading configuration. This doesn’t require you to write any custom interface etc.
Please note that when you define your host with CreateDefaultBuilder, It loads Application Configuration defined ASP.NET Core appsettings.json to be the default and will be available to use in middleware or any other component.
I am here injecting RequestDelegate and IConfiguration from the constructor of CustomMiddleware.
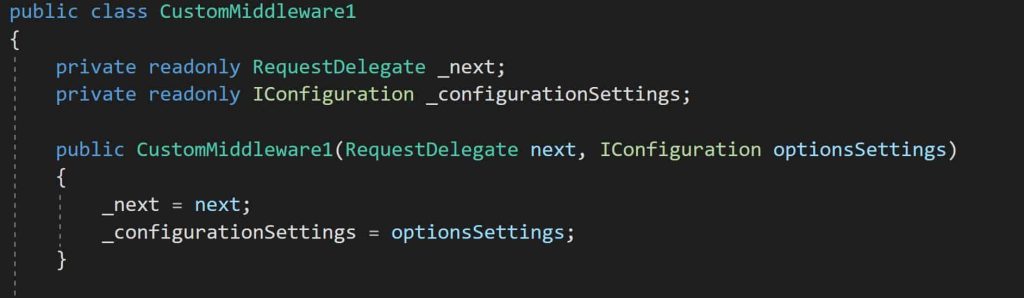
Let’s now add the InvokeAsync(Assuming we need asynchronous middleware behavior) method.
InvokeAsync method is defined as below,

Approach 2 – Using IOption to load the Config settings in Middleware
I am here injecting RequestDelegate and IConfiguration from the constructor of CustomMiddleware.

Below is how you can read and use the configuration values within the middleware component.

Below is how I created the Configuration mapping object i.e GlobalConfigurationSettings for the above appsettings.json file
public class GlobalConfigurationSettings
{
public Logging Logging { get; set; }
public CustomerConfig CustomerConfig { get; set; }
}
public class CustomerConfig
{
public string CustomerKeyurl { get; set; }
public string CustomerdetailsUrl { get; set; }
public Agency Agency { get; set; }
}
If you are using a non-prod or Prod environment like DEV, TEST or PROD then such environment-specific configuration details will also be available from appsettings.[EnvironmentName].json.
This will be taken care of by CreateDefaultBuilder a generic host builder interface in ASP.NET Core.
References:
- CreateDefaultBuilder and Configuration Management in .NET/ASP.NET Core
- Creating Typesafe Configuration in ASP.NET Core
That’s All! Happy Coding!
Do you see any improvement to the above code? Do you have any suggestions or thoughts? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.