MongoDB Auto-Increment fields in document using C#
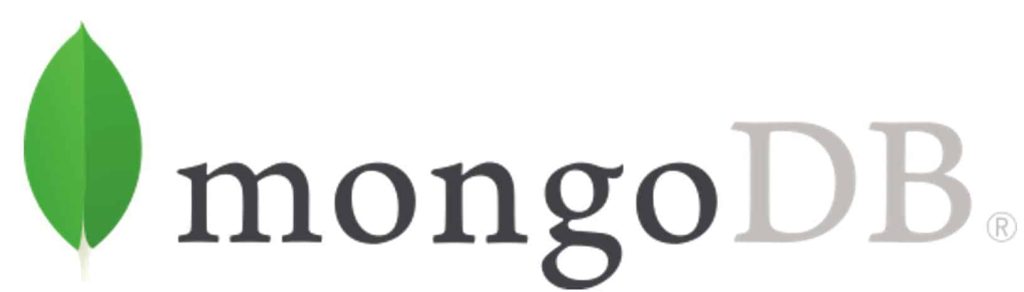
Today in this article will see how to perform a MongoDB C# driver Auto-Increment fields while update or inserting a document int the collection.
This auto-increment could be needed to keep the uniqueness of similar updates or there could be multiple use cases where you need these features.
Today in this article, we will cover below aspects,
We shall see C# MongoDB driver in .NET or .NET Core example to perform the same.
We will also how to return the mongo document after and before the modification to check if our updates are applied properly.
This could be a useful feature for many other use cases including versioning the data based on any updates to the mongo document.
The below query used and explained is just for demonstration purposes, however, the concept can be used for any of your custom query requirements.
Let’s use the below sample JSON document. We will update the document for one of the fields in the array i.e. ‘orderNumber‘.
Once updated successfully, we will return the document that got updated.
{
"userId": "12345",
"orderNumber": "100",
"Name": "Categories",
"Books": [
{
"name": "C# Book",
"catId": "5555",
},
{
"name": "Typescript Book",
"catId": "44444",
}
],
"orderDate":"2020-09-20T21:07:42.313+00:00"
}
In the above example, we shall concentrate on the orderNumber fields which we want to increment by counter 1 every time I receive a book order.
Establish the connection to the Database
Below is a basic skeleton code to establish the connection to the Database using the MongoDB C# driver.
var _mongoClient = new MongoClient("mongodb://your connection string");
var db = _mongoClient.GetDatabase("Library");
ar collection = db.GetCollection("Books");
Auto Increment Field in Mongodb
Let’s update the records using FindOneAndUpdateAsync and autoincrement the “OrderNumber” field,
var filter = Builders<BsonDocument>.Filter.And(
Builders<BsonDocument>.Filter.Eq("userid", userId));
var updates = Builders<BsonDocument>.Update.Inc("orderNumber", 1);
await collection.FindOneAndUpdateAsync(filter, updates);
Query explained,
- userid field will be matched with the input userId as filter criteria.
- FindOneAndUpdateOptions with options updates the document and increment the field “orderNumber” with incremental of 1 count.
Guidelines
- The
$inc
operator update or insert the fields as required. If the field does not exist,$inc
creates the field and sets the field to the specified value.
- It accepts positive and negative values.
- Use of the
$
inc
operator on a field with a null value might generate an error. New mongodb version might support this , please check this.
- This atomic operation within a single document update
After successful execution of the query, we shall see the document is updated with a new order number successfully.

References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.