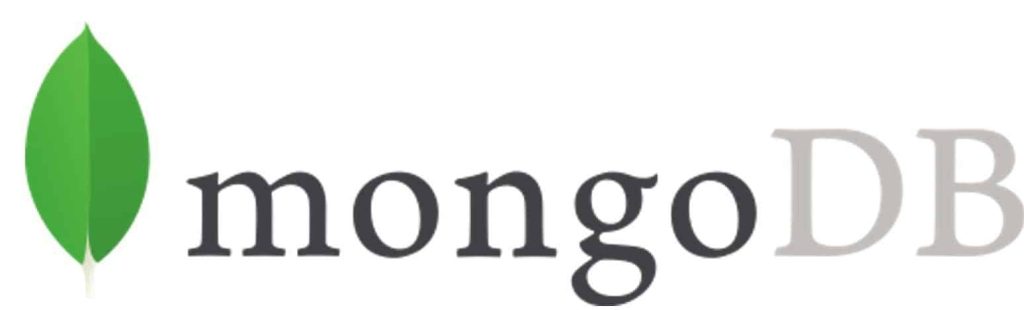
Today in this article, we will learn about MongoDB C# .NET – Change The Type of a field.
We will write a simple C# program to update the data type of field for all documents using MongoDB C#,
There are multiple approaches to achieving the data type changes.
We will cover below aspects in this article,
The below approach discussed is very useful when you don’t want to delete or drop the mongo fields but rather want to change the type of a field.
Getting started
Please create any .NET/.NET Core application.
Please add the MongoDB driver NuGet package using the Nuget Package manager.
PM> Install-Package MongoDB.Driver -Version 2.9.3
Note – Please use the latest version available.
Again, I shall keep the Mongo driver interface use simple here to concentrate on how to rename fields from existing documents.
Please visit for better approaches like using DI or IoC here,
Step I – Establish the connection with the MongoDB driver,
var _mongoClient = new MongoClient("mongodb://your connection string"); var db = _mongoClient.GetDatabase("Book");
Step II – Get the collection object for the given Mongo Database.
var collection = db.GetCollection("employee");
I have below Mongo documents where mongo schema and their fields are defined below.
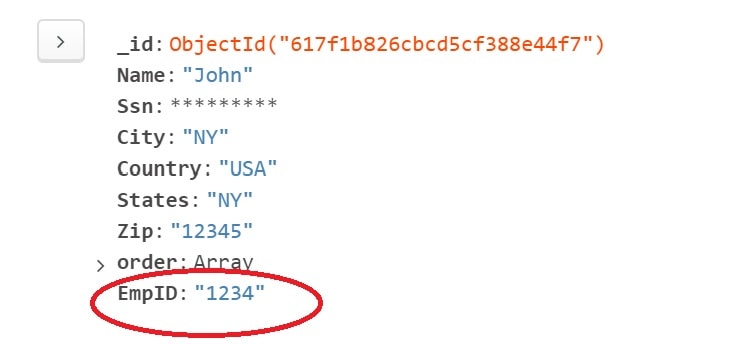
The above field “price” is defined as string which we will convert into int type
Command Pattern
{$set:{ "<field-name>" : {$<field type>: "$<field-name>"}}}
Example
Below is the C# equalavalnt code to update the field type from string to Int.
_mongoClient = new MongoClient(connectionString);
var db = _mongoClient.GetDatabase("TheCodeBuzz");
var collection = db.GetCollection<BsonDocument>("Books");
var filter = new BsonDocument("EmpID", new BsonDocument("$type", BsonType.String));
var query = new BsonDocument { { "$set", new BsonDocument { { "EmpID", new BsonDocument { { "$toInt", "$EmpID" } } } } } };
var pipeline = PipelineDefinition<BsonDocument, BsonDocument>.Create(stage);
var update = Builders<BsonDocument>.Update.Pipeline(pipeline);
var result = await collection.UpdateManyAsync(filter, update);
What is $toInt
$toInt is an Aggregation Type Expression Operator that Converts a given value to an integer. If the value is null or missing, $toInt
returns null. It returns errors when the value can not be converted
After a successful conversion, the data type will be changed to int as below,

Change Numeric int type to String
Similarly, one can use the below way to perform Int to string data type changes
var filter = new BsonDocument("EmpID", new BsonDocument("$type", BsonType.Int32));
var query = new BsonDocument { { "$set", new BsonDocument { { "EmpID", new BsonDocument { { "$toString", "$EmpID" } } } } } };
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.