MongoDB Rename Collection using script and Command Line
In this article, we shall see how to perform MongoDB Rename Collectionusing Command-Line like mongo shell. We shall also see a simple C# script to perform the same.
Today in this article, we will cover below aspects,
Please open the command prompt or PowerShell and log in to your database using a connection string.
Login to MongoDB
mongo < Connection string>
Example
>mongo mongodb+***://****:***********/thecodebuzz-*****-personal?authSource=admin&replicaSet=xxxxxxx-0&readPreference=primary&ssl=true
The above commands will let you log in to your MongoDB database cluster.
use <DBName>
Example:

The above command sets the given database as the current database. Example ‘CarTitles‘.
This database becomes the primary database for all respective commands executed.
MongoDB Rename Collection
Below is the command to rename Collection using Command-Line.
Command
db.<source-collection-name>.renameCollection("<new-collection-name>")
Example:
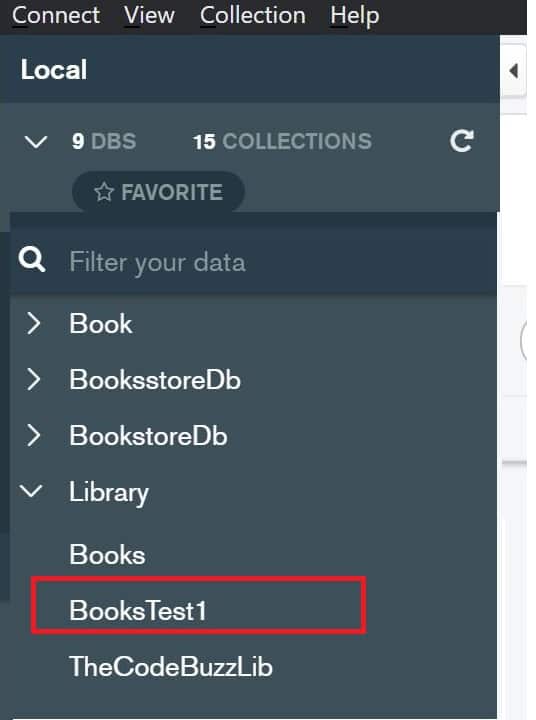
db.BooksTest1.renameCollection("TheCodeBuzzTest")
Post rename the collection will be updated as below,

The current database gets renamed and the user can see the success/failure of the commands.

The above shows successful operation with status as “OK” for the rename of the collection in the MongoDB database.
Rename MongoDB Collection using C#
Below is a sample code on the simple way to rename the collection.
class Program
{
static string connectionString = "mongodb://your connection string.";
private static MongoClient _mongoClient;
static async Task Main(string[] args)
{
_mongoClient = new MongoClient(connectionString);
var db = _mongoClient.GetDatabase("Library");
await db.RenameCollectionAsync("BooksTest1", "TheCodeBuzzTest");
}
}
Above C# sample, we are renaming Mongo Collection “BooksTest1” to target “TheCodeBuzzTest”.
Add Collection in MongoDB
The above command sets the given database as the current database.
db.TheCodeBuzzTest.insertOne( { x: 1 } ); OR db.createCollection('TheCodeBuzzTest');

References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.