MongoDB – How to verify if the update successful
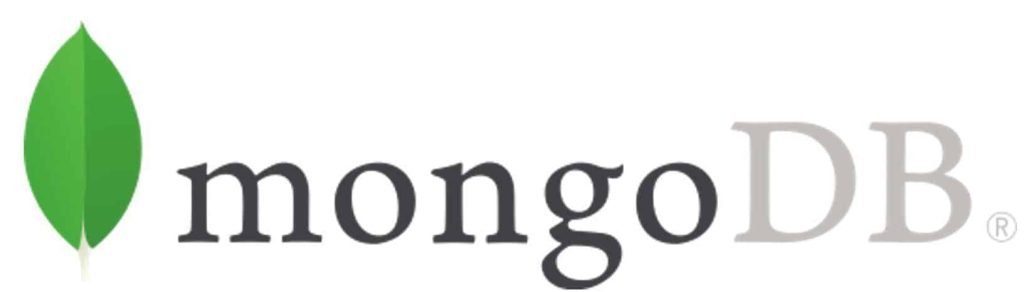
Today in this article, we will see in MongoDB – How to verify if the update successful
Mongo Driver provides an easy way to identify if the documents have been updated in the operations using the below basic properties,
Above properties or similar properties can be found in node.js or python or C# mongo driver
In C# Mongo driver, the above abstract properties are available for UpdateMany or UpdateManyAsync or BulkWriteAsync operation supported.
Get Updated count – UpdateMany() or UpdateManyAsync()
If using UpdateMany() or UpdateManyAsync() please use any of the properties like,
- MatchedCount or
- ModifiedCount.
Discussed properties not only confirm if an update is successful but also give a count of records updated in the given operations.
var updateBuilder = Builders<Library>.Update;
var update = updateBuilder.Set(doc => doc.books[-1].price, inputPriceBook);
var resultMongo = collection.UpdateMany(filter, update);
if (resultMongo.ModifiedCount > 0)
{
/// Your logic here
}
Get Updated count – UpdateOne() or UpdateOneAsync
If using UpdateOne or UpdateOneAsync, please use any of the properties like
- MatchedCount or
- ModifiedCount.
var updateBuilder = Builders<Library>.Update;
var update = updateBuilder.Set(doc => doc.books[-1].price, inputPriceBook);
var resultMongo = collection.UpdateOne(filter, update);
if (resultMongo.ModifiedCount > 0)
{
/// Your logic here
}
Get Updated count – BulkWrite or BulkWriteAsync
If using BulkWrite or BulkWriteAsync, please use any of the properties like
MatchedCount or
ModifiedCount.
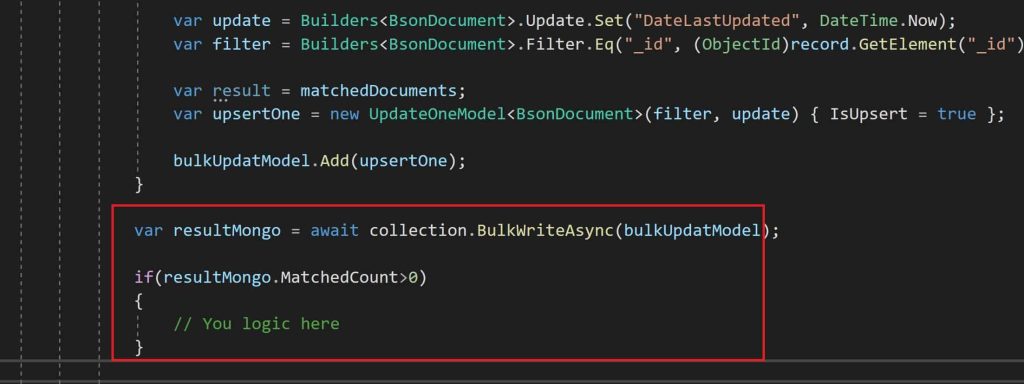
FindOneAndUpdateAsync verify Update
If using FindOneAndUpdateAsync, one can use the result and verify it with null to check if any updates or insert happen.
var returnDoc = await collectionnew.FindOneAndUpdateAsync<Library>(
filter: Builders<Library>.Filter.Eq("_id", userId),
update: update.Combine(updates),
options: new FindOneAndUpdateOptions<Library, Library>
{
IsUpsert = true,
ReturnDocument = ReturnDocument.After,
});
if(returnDoc!=null)
{
//your logic here
}
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.