Node – MongoDB Date Time query with examples

Today, in this article will cover Node.js – MongoDB Date Time query with examples.
We will see how to get records using a date filter like ‘greater than’ OR ‘less than’ or get the records between 2 dates.
We will use the MongoDB Node.js Driver package to install the Mongo driver and query MongoDB.
For more details – https://mongodb.github.io/node-mongodb-native
We will cover the below aspects in today’s article,
Getting started
Here below is a sample schema or document we shall use for the date range query,
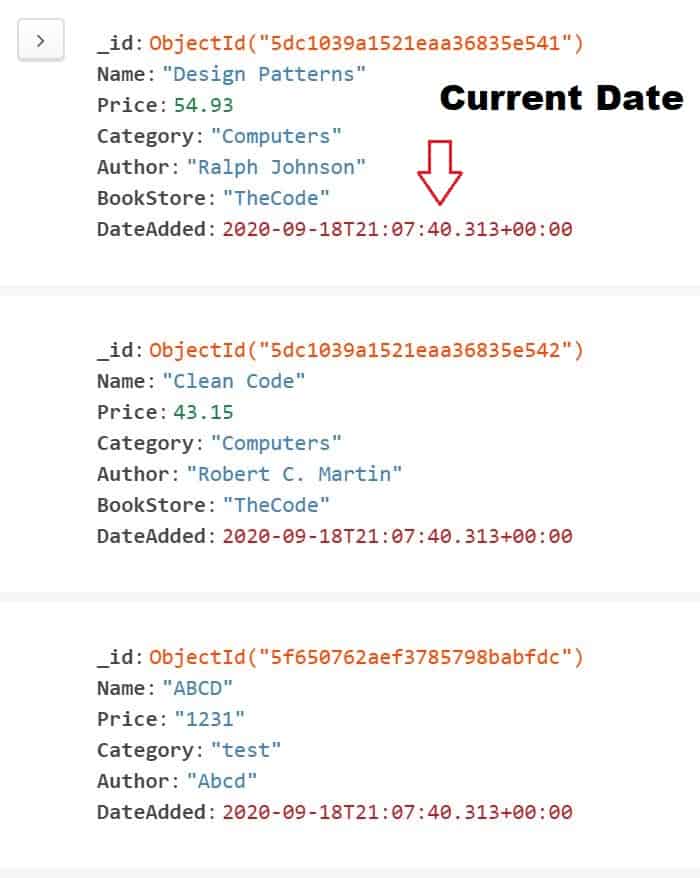
Install Node.js MongoDB driver
Please install the Node.js MongoDB driver package to get support for the MongoClient connection class and properties.
For more details visit- https://mongodb.github.io/node-mongodb-native
Add MongoDB Import statements
Please add below using namespaces
import { MongoClient } from 'mongodb';
Define MongoDB Connection
Using MongoClient class define the MongoDB Connection
const client = await MongoClient.connect(
'mongodb://your server:27017/',
{ useNewUrlParser: true, useUnifiedTopology: true }
);
Here we will be using the below query to get the documents between two dates in MongoDB Collection.
Node.js- Mongo Date greater than query (‘gt’)
Define the date
const queryDate = new Date('2023-11-01');
Define the filter
Lets define the date filter as below,
const filter = {
'DateAdded': {
'$gt': queryDate
}
};
Get the results
const coll = client.db('TheCodeBuzz').collection('Books');
const cursor = coll.find(filter);
const result = await cursor.toArray();
await client.close();
Complete sample code,
import { MongoClient } from 'mongodb';
# Define Node Mongo Connection
const client = await MongoClient.connect(
'mongodb://your server:27017/',
{ useNewUrlParser: true, useUnifiedTopology: true }
);
# define date for the query
const queryDate = new Date('2023-11-01');
# define filter for the query
const filter = {
'DateAdded': {
'$gt': queryDate
}
};
const coll = client.db('TheCodeBuzz').collection('Books');
const cursor = coll.find(filter);
const result = await cursor.toArray();
await client.close();
Mongo Atlas UI
The above query produces the same result as indicated below using ATLAS UI,
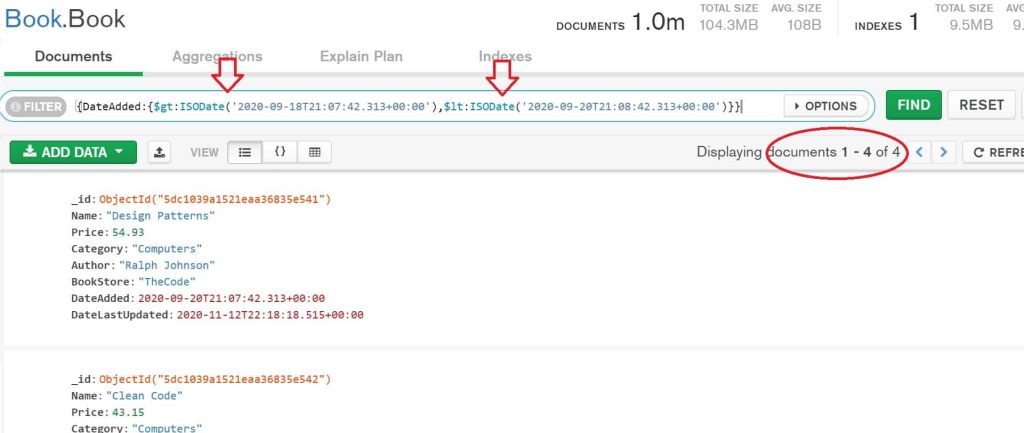
Node js- Mongo Date less than query (‘lt’)
Let’s define Node.js Mongo Datetime less than query (‘lt’)
# define date for the query
const queryDate = new Date('2023-11-01');
# define filter for the query
const filter = {
'DateAdded': {
'$lt': queryDate
}
};
Node js- Mongo Date less than and greater than date query
# define date for the query
const queryStartDate = new Date('2023-11-01');
const queryEndDate = new Date('2024-01-01');
# define filter for the query
filter = {
'DateAdded': {
'$gte': queryStartDate,
'$lt': queryEndDate
}
}
For more details on queries where no date field in the schema is available , please visit this article,
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.