Remove extra space from a string C#.NET
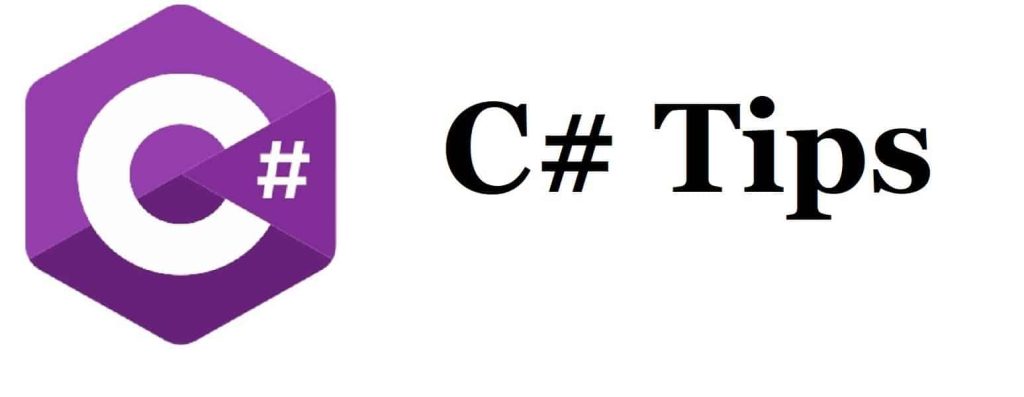
Today in this article, we will see how to Replace Extra Space With Single Space From A String.
You may want to remove any extra space between the string characters and keep just one space between the string words then we can use some handy techniques as discussed below.
Today in this article, we will cover below aspects,
One can use the Regex pattern or Without regex to receive the same.
Approach 1 – Replace Extra Space With Single Space Using Regex
It is simple to use regex and achieve this,
var myString = Regex.Replace(myString, @"\s+", " ").Trim;
- The above logic does all the tricks. Replace() method replaces the target string with the given provided string with the matching pattern specified.
- The trim() method will remove any leading or trailing whitespaces.
Example 1
"Best practices of software development"
Example 2
Below example uses leading and trailing occurrences of whitespace etc.
" Best practices of software development "
Output
Best practices of software
Approach 2 – Replace Extra Space With Single Space without Regex
Regex could affect the performance depending on how big the string value you process is.
If you are looking for an alternative to regex, you could try the below custom logic.
public static string ReplaceWhiteSpace(this string inputString)
{
StringBuilder stringBuild = new StringBuilder();
if (!string.IsNullOrEmpty(inputString))
{
bool IsWhiteSpace = false;
for (int i = 0; i < inputString.Length; i++)
{
if (char.IsWhiteSpace(inputString[i]))
{
if (!IsWhiteSpace)
{
stringBuild.Append(inputString[i]);
}
IsWhiteSpace = true;
}
else
{
IsWhiteSpace = false;
stringBuild.Append(inputString[i]);
}
}
}
return stringBuild.ToString().Trim();
}
Using any of the above approaches you can remove or replace extra space without any hassle.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.