Unit Test and Mock Automapper ASP.NET Core
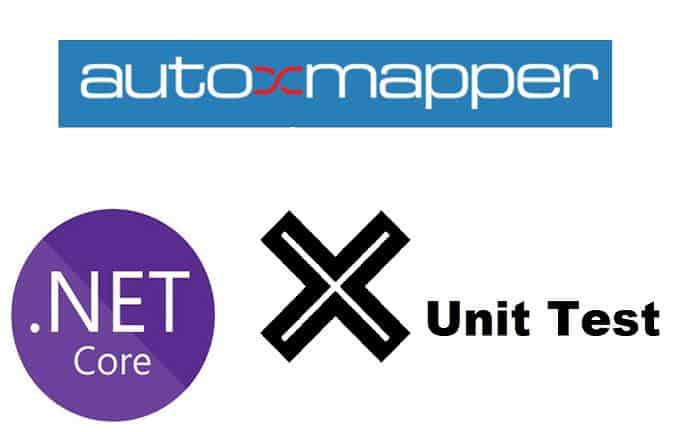
Today in this article we will see how to write Unit Test and Mock Automapper in ASP.NET Core application.
As we learned in our last article on Automapper, it was very easy to configure Automapper in the API pipeline using the Dependency Injection (DI) principle.
Although I found the IMapper interface does provide DI and allows us to program by Abstraction but still requires us to Initialize the Mapping configuration at startup.
Due to the same reason, IMapper can not be completely mocked by setting up a mock extension method in a regular way.
But we can very much use a declarative auto-mapper profile and inject it into our module.
Today in this article, we will cover below aspects,
Today I will talk about simple techniques to overcome this issue in this article.
Let’s first look at the SUT i.e. Software under test example.
Original Method where Automapper is used,

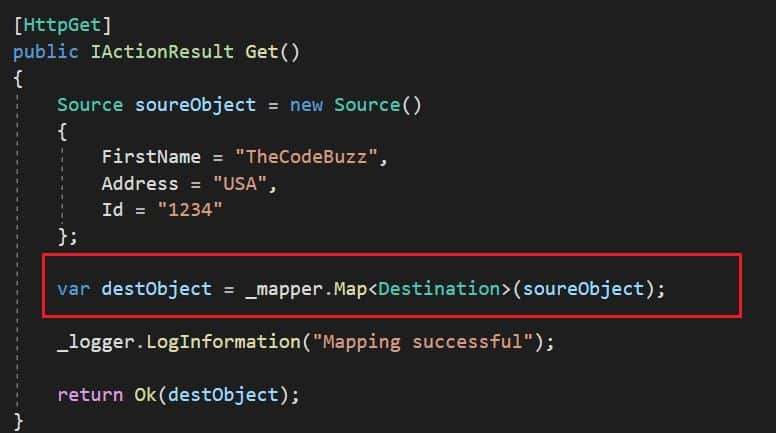
Please visit the below article for the complete code,
Below is how the profile is defined,
public class SourceMappingProfile : Profile
{
public SourceMappingProfile()
{
CreateMap<Source, Destination>()
.ForMember(dest => dest.Name, o => o.MapFrom(src => src.FirstName))
.ForMember(dest => dest.Identity, o => o.MapFrom(src => src.Id))
.ForMember(dest => dest.Location, o => o.MapFrom(src => src.Address));
}
}
The test project does not have an inbuilt IoC hence we will have to initialize the Automapper configuration manually i.e. by instance invocation.
Test Initialize Mapping Profile
Here in the XUnit Test class, the constructor of TestClass gets called before the execution of any Test method. (which is equivalent to TestInitialize in MS Test terminology.)
Here we will instantiate the Automapper interface i.e. IMapper as Singleton so that the same instance will be used as-is for all Test methods.
public class AutomapperASPNETCoreTests
{
private static IMapper _mapper;
public AutomapperASPNETCoreTests()
{
if (_mapper == null)
{
var mappingConfig = new MapperConfiguration(mc =>
{
mc.AddProfile(new SourceMappingProfile());
});
IMapper mapper = mappingConfig.CreateMapper();
_mapper = mapper;
}
}
[Fact]
public void AutomapperASPNETCoreTest_Get_Valid_Mock_Automapper()
{
..
..
..
Injecting Mapper profile in Test method
Here below is the implementation of TestMethod AutomapperASPNETCoreTest_Get_Valid_Mock_Automapper.
This method when gets executed will initialize the IMapper instance with the given configuration and profile.
All the test methods will use the same instance when for one execution to simplify test method executions.
Below is the final implementation of the Test method,

The above method uses the IMapper instance as defined in the Constructor of Test class.
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.