Unit Test and Mock IOptions <T> .NET Core
Today in this article, we shall see how to Unit Test and Mock IOptions <T> instance often used in Controller or other modules for injecting the configuration details.
Today in this article, we will cover below aspects,
The IOptions interface pattern provides access to configuration details in related classes as well as
the option to load config data depending on the situation.
The IOptions interface will be used to load the configuration as needed in the program.
Example:
public class ProducerController : ControllerBase { readonly ProducerConfigSettings _producerConfigSetting; public ProducerController(IOptions producerConfig) { _producerConfigSetting = producerConfig.Value; } . . }
The above shows ProducerController which is a controller class injecting Apsettings.json key-value pair using the IOption pattern.
For more details on IOption Pattern, please visit the below article,
Unit test IOption without Mock
In this approach, you certainly need not have to mock the Apsettings object. You can send test settings details via the settings object as below and test all positive and negative scenarios as required.
Example
//Arrange
ProducerConfigSettings appSettings = new ProducerConfigSettings()
{
Key1 = "testkey1",
Key2 = "Testkey2"
};
IOptions<ProducerConfigSettings> options = Options.Create(appSettings);
ProducerController controller = new ProducerController(options);
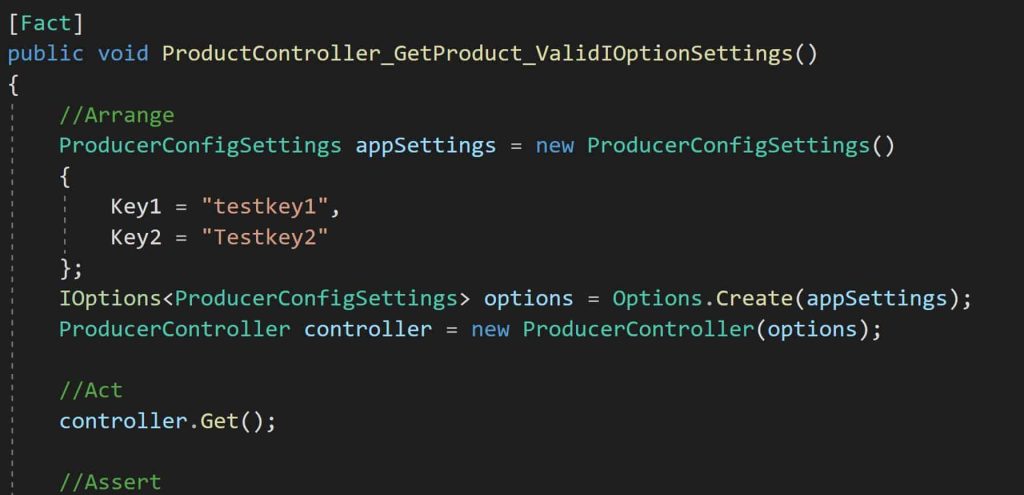
Unit Test IOption by Mock IOption
In this approach, you can mock the Apsettings object. You can send mocked settings details via the settings object as below and test all positive and negative scenarios as required.
//Arrange
ProducerConfigSettings appSettings = new ProducerConfigSettings()
{
Key1 = "testkey1",
Key2 = "Testkey2"
};
var mockIOption = new Mock<IOptions<ProducerConfigSettings>>();
// We need to set the Value of IOptions to be the SampleOptions Class
mockIOption.Setup(ap => ap.Value).Returns(appSettings);
ProducerController controller = new ProducerController(mockIOption.Object);

Finally, Using any of the above approaches when you run the test cases, it starts using test/mocked app settings, instead of pulling it from the actual apsettings.json file.

References:
That’s all! Happy coding!
Does this help you fix your issue?
Do you have any better solutions or suggestions? Please sound off your comments below.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Not working !
Hey Yehor – Thanks for your comments. May i know what issue your facing..