Unit Testing and dealing with List of Elements in Angular

In our last article on Unit Test Angular Component and Child Component, we got our Unit Test working with a component that has both the child component and injected services. We will cover Unit Test and mock List of Elements in Angular.
We will cover , Unit Test and mock List of Elements in Angular
Let’s write tests now that will let us see how to deal with lists of elements inside of our unit tests.
We’re going to write basically the same test that we’ve already written, except we’re going to count the DOM elements that are produced.
Open heroes.component.html, and notice down here on line 14 we have a li element, and that is created once for every hero in the heroes array. That heroes array is the property of the HeroesComponent that we’ve been dealing with.
- So, since we’re using our HEROES sample data that has four elements in it, we’re going to have four heroes, and therefore, four li elements are going to get created inside of this ul right here.
HEROES list is defined as below,
import { HeroesComponent } from "./heroes.component";
import { of } from "rxjs";
import { ComponentFixture, TestBed } from "@angular/core/testing";
import { Component, Input } from "@angular/core";
import { HeroService } from "../hero.service";
import { Hero } from "../hero";
import { By } from "@angular/platform-browser";
describe('Heroes Component', () => {
let fixture: ComponentFixture<heroescomponent>;
let mockHeroService;
let HEROES;
beforeEach(() =>{
mockHeroService = jasmine.createSpyObj(['getHeroes', 'addHero', 'deleteHero']);
HEROES = [
{ id: 1, name: 'IronMan', strength: 8 },
{ id: 2, name: 'Batman', strength: 8 },
{ id: 3, name: 'CaptainAmerica', strength: 7 },
{ id: 4, name: 'SuperMan', strength: 9 }
]
TestBed.configureTestingModule({
declarations: [HeroesComponent],
providers: [
{ provide: HeroService, useValue: mockHeroService }
]
})
fixture = TestBed.createComponent(HeroesComponent);
})
it('should set heroes correctly from the service', () => {
mockHeroService.getHeroes.and.returnValue(of(HEROES));
fixture.detectChanges();
expect(fixture.componentInstance.heroes.length).toBe(4);
})
})
- Let’s write the same test we wrote before, but instead of looking at the length of the array, let’s count the number of li elements that are created.
- Paste the below code in heroes.component.spec.ts:
it('should create one li for each hero', () => {
mockHeroService.getHeroes.and.returnValue(of(HEROES));
fixture.detectChanges();
expect(fixture.debugElement.queryAll(By.css('li')).length).toBe(4);
})
- Run the test using ng test command in the terminal. The tests pass.
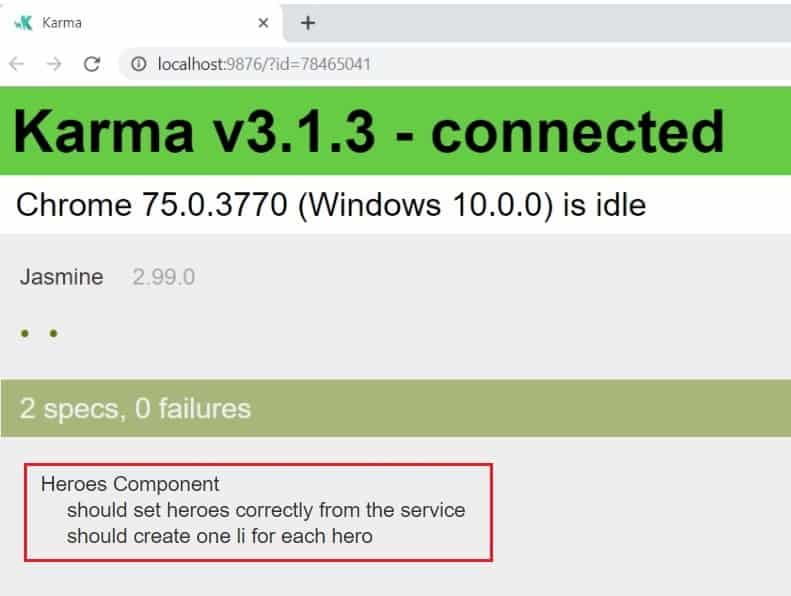
Refrenses,
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.