Upload and Download Files using Google Cloud Storage using C#

Today in this article we shall see how to Upload and Download files using C# – Google Cloud Storage.
We shall be using the C# Google storage library to upload files and also download files.
Today in this article, we will cover below aspects,
Getting Started
Create any .NET or .NET Core application.
Please Install Nuget package for Google.Apis.Auth
PM> Install-Package Google.Apis.Auth -Version 1.50.0
Install Google.Cloud.Storage.V1
Install Nuget package for Google.Cloud.Storage.V1
PM> Install-Package Google.Cloud.Storage.V1 -Version 3.4.0
Please add below using statements to your class files,
using Google.Apis.Storage.v1.Data;
using Google.Cloud.Storage.V1;
Upload a file to Google Cloud Storage
Below is a sample example for uploading a file to Google Cloud Storage.
We shall be uploading sample files from the local machine “CloudBlobTest.pdf” to google cloud storage.
string filePath = @"C:\Test\gcp\cloud\CloudBlobTest.pdf";
var gcsStorage = StorageClient.Create();
using (var f = File.OpenRead(filePath))
{
string objectName = Path.GetFileName(filePath);
gcsStorage.UploadObject(bucketName, objectName, null, f);
Console.WriteLine($"Uploaded {objectName}.");
}
The above code will upload the blob with the name “CloudBlobTest.pdf” to the google cloud storage location “thecodebuzz“.
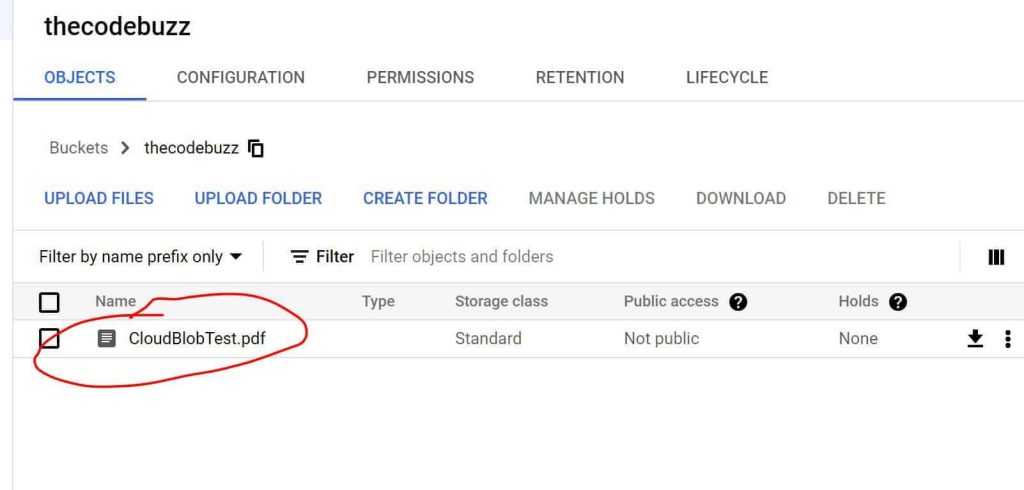
Download a file from Google Storage Bucket
Blob can be downloaded from google storage bucket as a file easily by using the below storage class methods.
Sample example demonstrates how to download a file from google cloud storage bucket to the local machine file path.
Let’s download the same file i.e CloudBlobTest.pdf from the GCS bucket.
Downloaded blob from GCS will be saved to below output path,
string fileDownloadPath = @"C:\Test\gcp\cloud\CloudBlobTest_Out.pdf";
string objectBlobName = "CloudBlobTest.pdf";
var gcsStorage = StorageClient.Create();
using var outputFile = File.OpenWrite(fileDownloadPath);
gcsStorage.DownloadObject(bucketName, objectBlobName, outputFile);
Console.WriteLine($"Downloaded {objectBlobName} to {fileDownloadPath}.");
Once executed the file will be downloaded to the local path successfully.
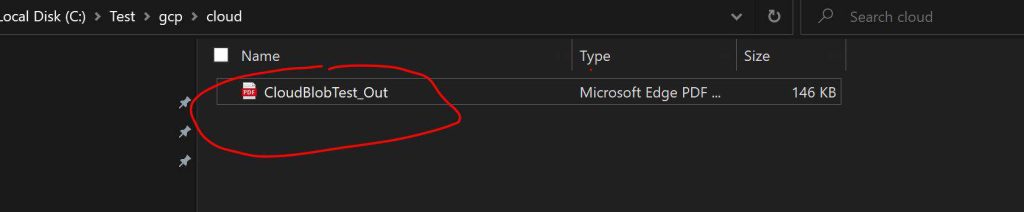
Please make sure to set up Environment Variable “GOOGLE_APPLICATION_CREDENTIALS” using the service account JSON file.
Environment.SetEnvironmentVariable("GOOGLE_APPLICATION_CREDENTIALS", "secured-key.json");
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.