Use IHostedService to perform background tasks in Console .NET C# application
Today in this article we shall see how to use IHostedService in console applications.
We will see how to use IHostedService to perform background tasks and handle the application lifetime events using IHostApplicationLifetime.
The typical idea of having to use IHostedService in the host or non-host application is to be able to perform multiple background tasks similar to WebHost workloads which we generally see in HTTP WebAPI or MVC applications.
Today in this article, we will cover below aspects,
IHostedService interface helps you to easily implement hosted services applications. You can register multiple background tasks that run in the background while the host is running.
How to Use IHostedService
Step 1 – Register IHostedServices
Add one or multiple IHostedServices
into the Host is by registering them through the AddHostedService extension method. Please see the below steps,

Step 2 – Implement the IHostedService interface
Implement the IHostedService interface and override the supported method.
public class SQLDataBatchProcessorService : IHostedService
{
private readonly ILogger _logger;
private readonly IBusinessLayer _business;
public SQLDataBatchProcessorService(
ILogger<SQLDataBatchProcessorService> logger,
IBusinessLayer business)
{
_logger = logger;
_business = business;
}
public Task StartAsync(CancellationToken cancellationToken)
{
_appLifetime.ApplicationStarted.Register(OnStarted);
_appLifetime.ApplicationStopping.Register(OnStopping);
_appLifetime.ApplicationStopped.Register(OnStopped);
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return Task.CompletedTask;
}
private void OnStarted()
{
}
private void OnStopping()
{
}
private void OnStopped()
{
}
}
Step3: Inject IHostApplicationLifetime
Inject the IHostApplicationLifetime service into class to get better control over how to perform pre-post start-up operations. This interface lets you handle post-startup and graceful shutdown of tasks.
The interface also gives you a StopApplication
the method that lets you perform a graceful shutdown of the application.
Below is an example of HostedService
implementation that registers IHostApplicationLifetime
events,
public class SQLDataBatchProcessorService : IHostedService
{
private readonly ILogger _logger;
private readonly IHostApplicationLifetime _appLifetime;
private readonly IBusinessLayer _business;
public SQLDataBatchProcessorService(
ILogger<SQLDataBatchProcessorService> logger,
IBusinessLayer business,
IHostApplicationLifetime appLifetime)
{
_logger = logger;
_appLifetime = appLifetime;
_business = business;
}
public Task StartAsync(CancellationToken cancellationToken)
{
_appLifetime.ApplicationStarted.Register(OnStarted);
_appLifetime.ApplicationStopping.Register(OnStopping);
_appLifetime.ApplicationStopped.Register(OnStopped);
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return Task.CompletedTask;
}
private void OnStarted()
{
// Perform post - startup activities here
try
{
_logger.LogInformation("OnStarted has been called.");
_logger.LogInformation("Application {applicationEvent} at {dateTime}", "Started", DateTime.UtcNow);
_business.PerformBusiness();
_logger.LogInformation("Application {applicationEvent} at {dateTime}", "Ended", DateTime.UtcNow);
_appLifetime.StopApplication();
}
catch (Exception ex)
{
_appLifetime.StopApplication();
}
}
/// <summary>
///
/// </summary>
private void OnStopping()
{
// Perform on-stopping activities here
_logger.LogInformation("OnStopping has been called.");
}
/// <summary>
///
/// </summary>
private void OnStopped()
{
// Perform post-stopped activities here
_logger.LogInformation("OnStopped has been called.");
}
}
Once the application is configured successfully, the Application will be executed in the background and will execute all application start and shutdown events in order.
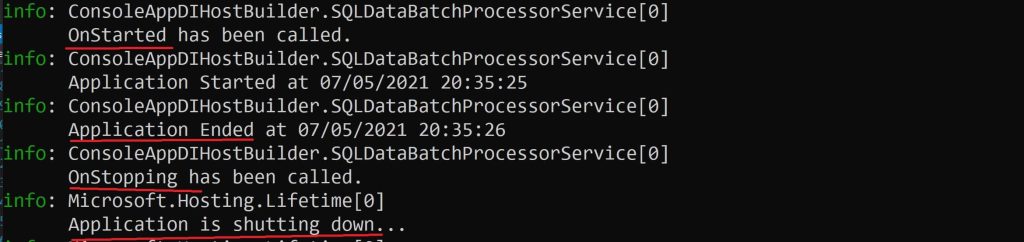
References :
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.