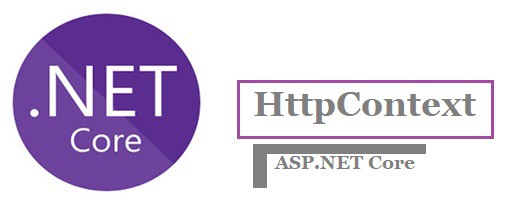
Today in this article will see how to access HttpContext in ASP.NET Core or .NET C# application.
You might find multiple needs to access HTTP Context in your application example, reading or adding custom headers for credentials, secured token, API version, etc.
You may find this metadata access needed in various applications including MVC or ASP.NET Core (.NET 3.1 or .NET 6) etc.
Today in this article, we will cover below aspects,
Today we will see a few approaches to achieve the same.
Access HttpContext in .NET from Controller
Accessing HTTPContext through the Controller is easy. You get direct access to HttpContext and its Request or Response metadata easily.
Example
[HttpGet]
public IActionResult GetData()
{
HttpContext.Request.Headers.Add("X-reponse-from-controller","test123");
return Ok();
}
Access HTTPContext from the Repository or custom module
If you need to access headers or any HttpContext metadata in other services or modules then please use HttpContextAccessor.
Below we are passing the IHttpContextAccessor instance via constructor injection and later using the same instances to access the HttpContext
class EmployeeRepository : IEmployeeRepository
{
private readonly IHttpContextAccessor _httpContextAccessor;
public EmployeeRepository( IHttpContextAccessor httpContextAccessor)
{
_httpContextAccessor = httpContextAccessor;
}
public void GetEmployeeByID(int employeeID)
{
string header =
_httpContextAccessor.HttpContext.Request.Headers["X-Custom-Header"];
PerformBusinessLogic(header);
}
..
}
To achieve the above please register IHttpContextAccessor in IoC container by updating the ConfigureServices method as below.

Access HTTPContext in ASP.NET using Custom Middleware
If you want to access HttpContext with intention of doing more meaningful stuff then the Adding Custom Middleware ASP.NET Core approach is preferable.
This middleware approach help centralizes your logic and can be made more generic. This is also a very good means of addressing your cross-cutting concerns.

Access HTTPContext in ASP.NET Core using Inline Middleware
You can also easily access HttpContext using lightweight inline middleware as below,

Thread Safety of HTTPContext
HttpContext or HttpContext.Current object is meant to be created and consumed for the current Request and Response processing only.
Passing HttpContext object into a multi-threading task could lead to unexpected behavior.
Instead, always pass the metadata, reading it from the Context and passing it along.
Please read more on : HttpContext Best Practices in .NET
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Reference:
Summary
Today in this article we learned how to access the HttpContext in the ASP.NET Core application. We looked at adding multiple approaches to accessing the context using example Controller, Middleware, and using the IHttpContextAccessor interface approach.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Can access Credentials in Context Class which is inherited from DbContext?
Hello Imy – Thanks for your query. As per my understanding, you can use the HttpContext in the class which is inherited from DBContext also. You will need to inject IHttpContextAccessor HttpContext to access as explained already above.
Nice one ! Thanks.
Thanks Felix , Glad you liked it