Add custom headers to ASP.NET Core Request

Today in this article we shall see a few common approaches to Add custom headers to ASP.NET Core Request.
Below the discussed approach, you can very much use it for .NET Core 3.1 or .NET 5 or 6 version.
We shall see below a few approaches to add the headers,
If you are looking to add a custom header to a Response then please refer to the below article,
Use HTTPClientFactory to Add custom headers to ASP.NET Request
If you are using HTTPClientFactory-based HttpClient, you can send Custom headers in the request using
The below example shows we can use the DefaultRequestHeaders property to define the headers while using HTTPClientFactory.
Example:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddHttpClient("AccountClient", c => //Named Http Client
{
c.DefaultRequestHeaders.Add("X-Custom-Env", "TEST");
});
}
Or
For Typed HttpClient
services.AddHttpClient<AccountClient,IAccountClient>()
{
c.DefaultRequestHeaders.Add("X-Custom-Env", "TEST");
});
If you need to read and verify the custom header it can be done using Request. Headers which is dictionary objects and contain a collection of headers with Key and value pairs.
Here is an example of how we can read and verify the header on the receiving service/API side.
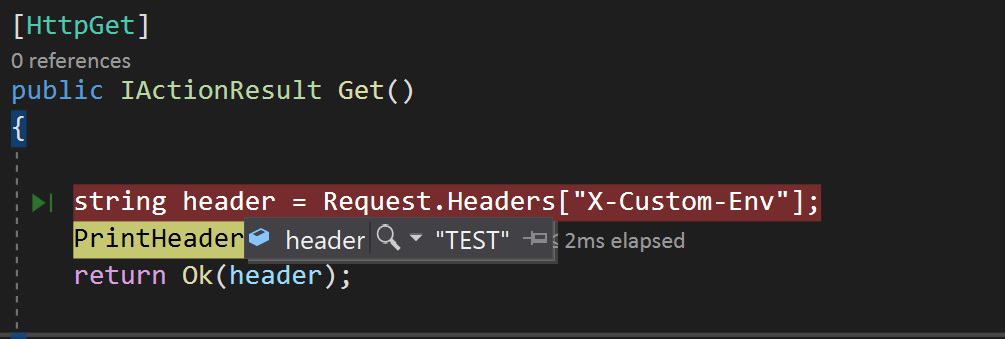
OR
One can also use HttpContext to access required headers and verify.
[HttpGet("employee")]
public IActionResult GetDetails()
{
string header = HttpContext.Request.Headers["X-Custom-Env"];
PrintHeader(Request.Headers);
return Ok(header);
}
Using HeaderPropogation Middleware to add header
Adding HeaderPropogation Middleware is also a very simple way of adding the headers and propagating them to the next request.
Please see here below article on how to use HeaderPropogation Middleware to send custom headers to outgoing requests.
Using HTTPClient to add Header
If you are using a basic HttpClient client in your code, then a custom header can be added as below in the outgoing request,
Sample Example:

Using IHTTPContextAccessor to fetch the custom header
The above–discussed HttpContext or Request class gives us access to metadata including headers of given HttpContext.
However, if you need to access headers or any HttpContext metadata in other services or modules like a library or other application project then please use IHTTPContextAccessor.
Example:
The below example shows how we can use IHttpContextAccessor in the repository class ann add required headers.
class EmployeeRepository : IEmployeeRepository
{
private readonly IHttpContextAccessor _httpContextAccessor;
public EmployeeRepository( IHttpContextAccessor httpContextAccessor)
{
_httpContextAccessor = httpContextAccessor;
}
public void GetEmployeeByID(int employeeID)
{
string header =
_httpContextAccessor.HttpContext.Request.Headers["X-Custom-Header"];
PerformBusinessLogic(header);
}
..
}
Using Middleware to add headers
Add custom or generic headers to REST API requests using the middleware approach as explained in the below article.
This article lists how to add an HTTP header to the response object but you can use the same approach for adding a header to the Request object too.
That’s All, Happy Coding !!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References :
Summary
Today in this article we learned a few common approaches to adding a Custom Header to an ASP.NET Core Request.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.