Typed HTTPClient using HttpClientFactory in ASP.NET Core
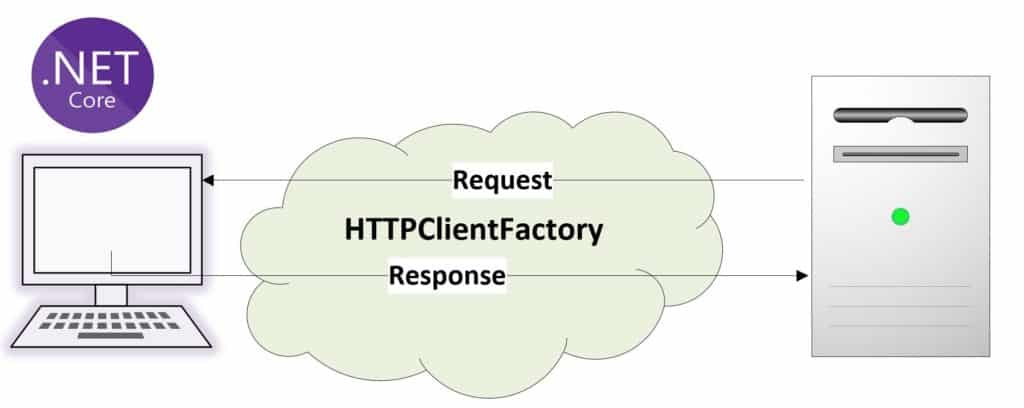
In today’s post, we will see how to create typed HTTPClient requests using HTTPClientFactory in .NET Core or ASP.NET Core.
In our last article, we understood the Differences between HTTPClient and HTTPClientFactory and also learned the Best practices for using HTTPClientFactory to create an HTTPClient object addressing the known issues of HTTPClient usage in the traditional way in ASP.NET Core.
Today in this article, we will cover below aspects,
In our previous article, we already also looked at Basic HTTPClient and Named HTTPClient approaches to create HTTPClient requests using a similar concept.
Create Typed HTTPClient
Let’s look at the step by step to understand and create a Typed HTTPClient approach where we shall be creating typed HTTPClient using the IHTTPClientFactory interface.
Create an ASP.NET Core Project

Add using namespace ‘System.Net.Http’ in the code to access HTTPClient and IHTTPClientFactory
Please update the ConfigureServices method in Startup.cs as below,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
//Create Typed client
services.AddHttpClient<AccountClient>();
}
Below is our typed HTTPClient i.e AccountClient implementation
AccountClient class here not just addressing basic class responsibility. It is mainly addressing HttpClient creation through DI. This class will make sure you get the best available HttpClient object from the pool and address your calls.
Defining AccountClient using services.AddHttpClient will let you attach any specific requirements like a custom header certificate or compression logic easily.
public class AccountClient
{
private readonly HttpClient _client;
private readonly IConfiguration _configuration;
public AccountClient(HttpClient client, IConfiguration configuration)
{
_client = client;
_configuration = configuration;
}
public async Task<string>GetAccount()
{
var uri = new Uri(_configuration.GetValue<string>("AccountURL"));
var response = await _client.GetAsync(uri);
if (response.IsSuccessStatusCode)
{
return response.Content.ReadAsStringAsync().Result;
}
else
{
return null;
}
}
}
Typed HTTPClient via Controller DI
Once defined the typed HTTPClient AccountClient can be injected from the Constructor in any other module or class where we need to call HTTP services.
This instance can be used for invoking the required operations as shown below,
Below is an example using Controller but ideally your actual implementation, you might call it in Service layer or Data access layer,or Infra layer.
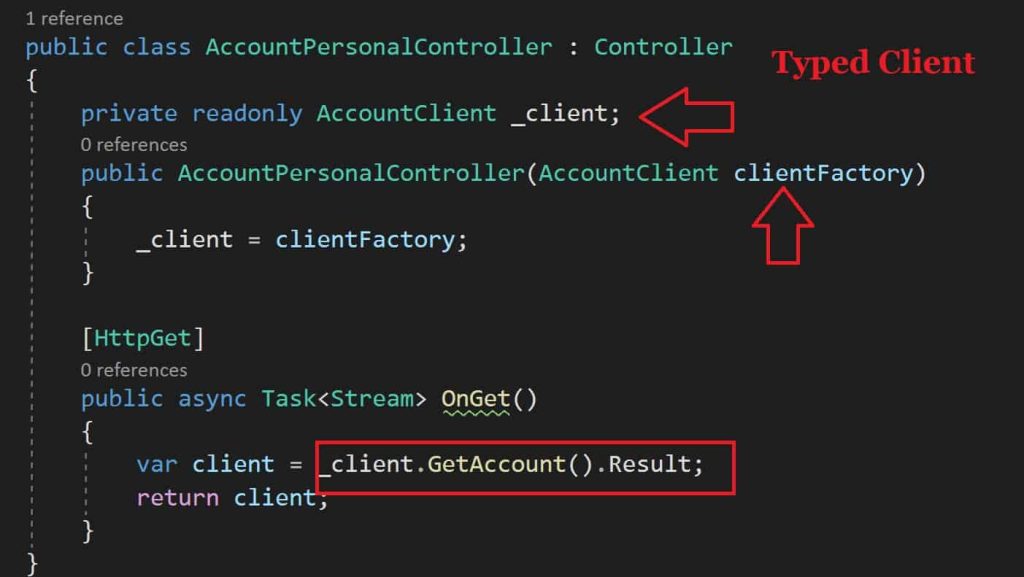
AccountClient will be available to use in every class/module in a project as required. Lifetime management of injected HTTPClient instances will be done through the API pipeline itself.
Additionally, this technique lets you control and configure HTTPClient’s request with custom configuration of Policy, Security, or delegates as required.
References:
- Using HTTPClient Best Practices and Anti-Patterns
- Create Named HTTPClient using IHttpClientFactory in ASP.NET Core
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
In this article, we looked at how to use HTTPClientFactory to create a Typed HTTPClient request object to invoke HTTP services in ASP.NET Core. This technique also lets you control lifetime and configure HTTPClient’s request with custom Policy, Security, or delegates as required.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.