HttpClientFactory in ASP.NET Core with examples
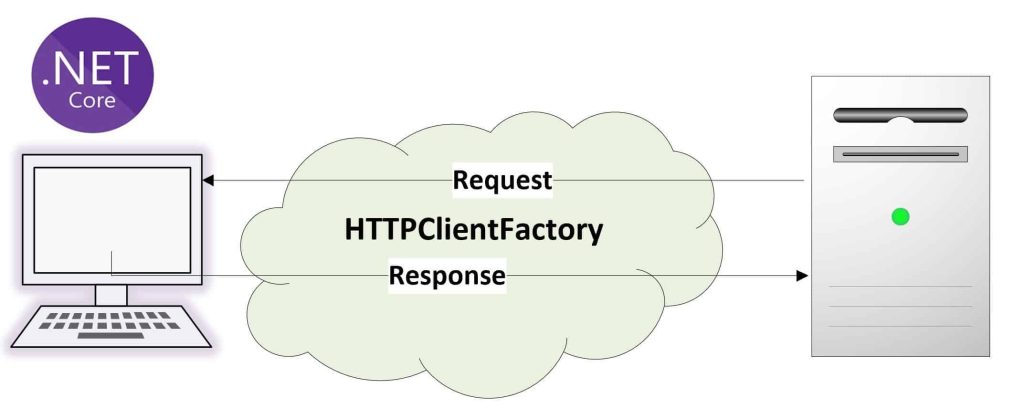
In today’s post, we will see how to use HttpClientFactory in ASP.NET Core with examples in ASP.NET Core and invoke HTTP Services in .NET Core or ASP.NET Core.
It’s a best practice to use the HTTPClientFactory object to create an HTTPClient object as it addresses known issues related to HTTPClient usage in the traditional way in .NET Core.
Today in this article, we will cover below aspects,
- HTTPClient in .NET Core – Common Issues
- Resource Exhaustion Problem
- Stale DNS (Domain Name System) problems
- Create an HTTP request using HTTPClientFactory – Guidelines
- Basic HTTPClient Request
- HttpClientFactory -Named HTTPClient usage with examples
- HttpClientFactory -Typed HTTPClient usage with examples
- Summary
We shall also understand a few approaches to create HTTPClient requests using the below techniques,
- Basic HTTPClient
All the above techniques leverage the IHTTPClientFactory interface to DI (Dependency Injection) required client object.
DI with middleware framework lets the API pipeline control the lifetime management of HTTPClient request objects instead of doing it manually.
Over the years it has been observed a few common issues like resource exhaustion caused by multiple invocations of the HTTP request object.
This was also followed by the realization that one should create Static or Singleton HTTP Client instances but which again ultimately found to be an issue in the recent .NET Core framework in the form of Stale DNS problems.
HTTPClient in .NET Core – Common Issues
Let’s discuss a few issues associated with using HttpClient in the old way.
Resource Exhaustion Problem
This was a commonly known issue while using the HTTPClient objects.
Please note that the HTTPClient implements IDisposable.
So basic understanding is to use ‘using(..)’ blocks to dispose of the objects but using blocks doesn’t release the socket immediately when heavy load operations are performed. Let’s not forget that socket objects are unmanaged resources.
Characteristics and Limitations:
- Each new HTTPClient object creates a new socket instance.
- Instantiating HTTPClient objects for each request might exhaust the number of sockets available. Example – Async operation with heavy loads.
- HTTPClient object doesn’t release the sockets immediately even if it is called using the “using” (IDisposable) block.
- This may lead to Socket exceptions.
HTTPClient creating using ‘new’ and ‘using’ blocks,

As per Microsoft guidelines,
HttpClient is intended to be instantiated once and re-used throughout the life of an application. Especially in server applications, creating a new HttpClient instance for every request will exhaust the number of sockets available under heavy loads. This will result in SocketException errors.

See here for more details.
Stale DNS (Domain Name System) problems
As discussed above a Singleton or Static HTTPClient object as specified above should help to resolve the most issues.
But in .NET Core, it was found that Singleton or Static HTTPClient object doesn’t respect the DNS update or change in the .NET core.
This is an additional problem that was found in the .NET Core framework. I am not sure if this issue exists in the regular .NET framework
The above common problems can be easily resolved by using HTTPClientfactory introduced recently in .NET Core ( 2.1 and above).
Top 10 benefits pf HttpClientFactory
Create an HTTP request using HTTPClientFactory – Guidelines
Create an ASP.NET Core Project

Let’s look at the basic HttpClient approach where we shall be creating plain HTTPClient using HTTPClientFactory.
Basic HTTPClient Request
You create an HTTPClient object using HTTPClientFactory.
HTTPClientFactory lets you DI inject the HTTPClient objects using an explicit dependency principle. Required instances can be enabled and disabled as required from the API pipeline as middleware.
Update ConfigureServices method
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddHttpClient();
}
Once injected HTTpClientFactory from the Your data Service Constructor, the instance can be used for creating the HTTPClient object as shown below,
The below example shows HttpClientFactory injected through Controller but ideally you can inject it in your DataService model wherever appropriate.

HttpClientFactory -Named HTTPClient usage with examples
I have discussed this technique in detail in the below post,
This technique has an advantage you can configure multiple HTTP clients with custom configuration as required like using policy, network credentials, specific headers, or security tokens as needed.
HttpClientFactory -Typed HTTPClient usage with examples
This is also one of the most preferred and easy techniques to create type HTTP Client objects.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
In this article, we looked at how to use HTTPClientFactory for creating HTTPClient request objects to invoke HTTP Services in .NET Core or ASP.NET Core. We saw three basic approaches to creating HTTPClient using HTTPClientFactorylike Basic HTTPClient, Named HTTPClient, and Typed HTTPClient request.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.