How to use C# HttpClientHandler with IHttpClientFactory
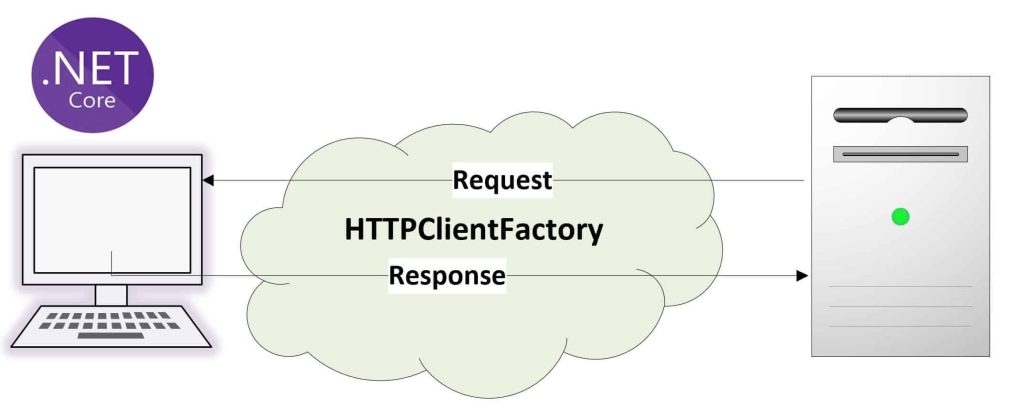
In today’s post, we will see how to use C# HttpClientHandler with HttpClientFactory for creating HTTPClient requests.
In general, HttpClientHandler can be used to configure a specific configuration like custom policy, headers, security mechanism, compression, certificates, etc.
We shall cover the below aspects in this article,
In our last article, we learned multiple approaches to create HTTPClient requests using like,
It is very simple to use HttpClientHandler with a named or typed HTTPClient.
C# HttpClientHandler with Named HTTPClient request
One can use ConfigurePrimaryHttpMessageHandler to add a delegate to configure the HttpMessageHandler for specific configurations like Network credentials or client certificates etc.
The sample code is as below,
HttpMessageHandler with Network credentials or Basic Authentication
Using HttpClientHandler with Network credentials or using HttpMessageHandler Basic Authentication can be achieved using the below,
services.AddHttpClient("PayBillClient", c =>
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("PayBillURL"));
// PayBill API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/xml");
}).ConfigurePrimaryHttpMessageHandler(() =>
{
return new HttpClientHandler()
{
UseDefaultCredentials = true,
Credentials = new NetworkCredential(Configuration.GetValue<string>("UserName"),
Configuration.GetValue<string>("Password")),
};
});
HttpClientHandler specifying compression configuration
You can configure HttpClientHandler to specify Compression/Decompression configuration as shown below,
services.AddHttpClient("AccountClient", c => //Named Http Client
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("AccountURL"));
// Account API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/json");
}).ConfigurePrimaryHttpMessageHandler(() => new HttpClientHandler
{
AllowAutoRedirect = false,
AutomaticDecompression = DecompressionMethods.Deflate |
DecompressionMethods.GZip
});
HttpClientHandler specifying ClientCertificate configuration
You can configure HttpMessageHandler to specify ClientCertificate configuration as shown below,
services.AddHttpClient("AccountClient", c => //Named Http Client
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("AccountURL"));
// Account API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/json");
}).
ConfigurePrimaryHttpMessageHandler(() =>
{
var handler = new HttpClientHandler();
handler.ClientCertificates.Add(new X509Certificate());
return handler;
});
One can specify multiple certificates using X509Certificate2Collection as shown in the below code,
services.AddHttpClient("AccountClient", c => //Named Http Client
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("AccountURL"));
// Account API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/json");
}).
ConfigurePrimaryHttpMessageHandler(() =>
{
var handler = new HttpClientHandler();
var clientCertificates = new X509Certificate2Collection {/*specify your certicates her*/};
handler.ClientCertificates.AddRange(clientCertificates);
});
If needed one handle call back event for certificate validation on the server by adding the below code,
handler.ServerCertificateCustomValidationCallback = ValidateServerCertificate;
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References:
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.