Get JSON Array using Configuration in ASP.NET Core
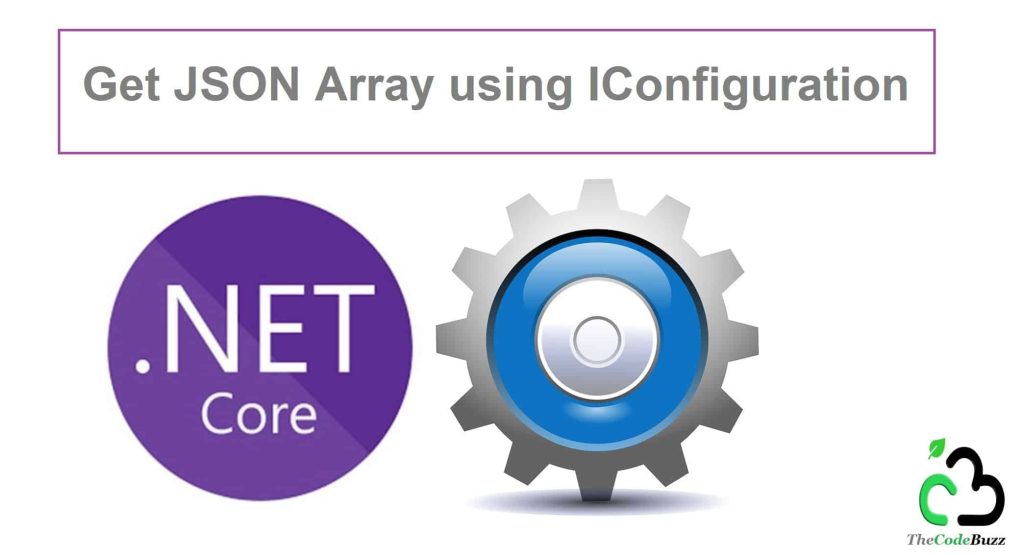
In this article, we shall see how to Get JSON Array using Configuration in ASP.NET Core.
This could be a requirement multiple times when using apsettings.json you may want to retrieve array values easily and consume them in the application.
Today in this article, we will cover below aspects,
Getting Started
Create ASP.NET Core API
Let’s create an ASP.NET core API application.
You can use Core 3.1 or .NET 6.0+ application.

Please choose any ASP.NET Core API project template. The filter is supported in most ASP.NET Core project templates.
I shall be using the below sample apsettings.json to demonstrate this,
apsettings.json
{
"Customer": {
"CustomerKeyurl": "http://customer/key",
"CustomerdetailsUrl": "http://customer/id",
"Agency": {
"AgencyID": "subvalue1_from_json",
"AccountKey": 200
},
"Agencies": [
"Agency1",
"Agency2",
"Agency4",
"Agency5",
"Agency6"
]
}
}
We shall now concentrate on how to access the Agencies field as JSON arrays.
We shall two basic approaches i.e using IConfiguration and IOption approach explained in detail below,
Using IConfiguration
Please note that there is inbuilt support in .NET Core to provide access to config details.
Once the application is hosted, config details are actually available via IConfiguration in the app’s Dependency Injection (DI) container.
This access is provided by CreateDefaultBuilder() in Program.cs.
_agencies = _configuration.GetSection("Customer:Agencies").Get<string[]>().ToList();
Using the above concept we can retrieve the Agencies array as below,

Alternatively, you can very much use another way using GetChildren() to fetch the array of JSON,
_agencies = _configuration.GetSection("Customer:Agencies") .GetChildren() .ToList();
Using IOption pattern to fetch the configuration
We can Map configuration to a POCO object by loading the configuration details into strongly typed global classes or can load them into related classes using IOptions.
Below is the equivalent Configuration model created representing the above apsettings.json. We shall try to fill string[] Agencies from the values in the config file.
public class ConfigDetails
{
public Customer Customer { get; set; }
}
public class Customer
{
public string CustomerKeyurl { get; set; }
public string CustomerdetailsUrl { get; set; }
public Agency Agency { get; set; }
public string[] Agencies { get; set; }
}
public class Agency
{
public string AgencyID { get; set; }
public int AccountKey { get; set; }
}
Here we will be using the IOption interface to load the configuration as needed in the application. Before using this option we need to perform the below few steps.
ConfigServices method can be used to inject this configuration object instantiated and DI this object objects in other parts of the application through the explicit dependency principle.
Please add the below line in the Configservices method,
services.Configure<ConfigDetails>(Configuration);
That’s all! Now you can access ConfigDetails defined with all apsettings.json values using IOption patterns as shown below,
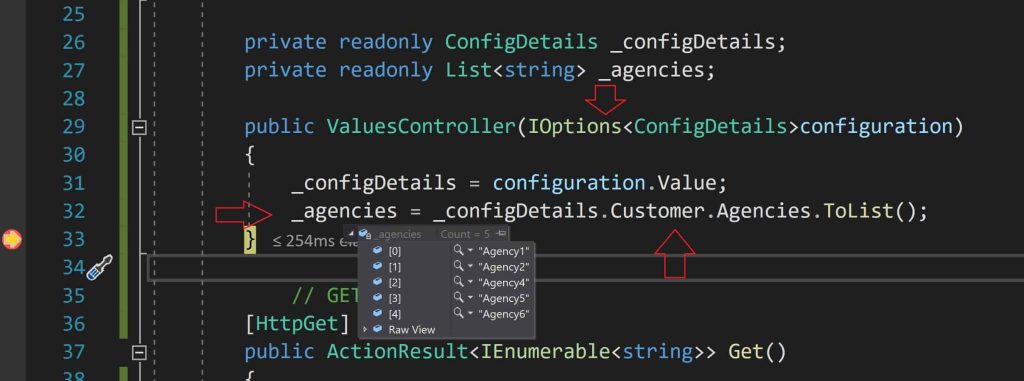
Finally, we can see the Agencies object filled with JSON array values from the configuration. This pattern gives access to configuration details in related classes and has the choice to load config data based on the need.
That’s all. Hope you find this article useful.
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.