Configure Certificates with HttpClient Authentication

In today’s post, we will see how to Configure certificates with HttpClient requests using .NET or ASP.NET Core applications.
We shall cover certificate Authentication for the below HttpClient types,
- Regular HttpClient using a certificate and the HttpClientHandler
- HttpClient from IHttpClientFactory and HttpClientHandler to configure Certificates
Client calling services with certificates enabled have to pass required certificates with every HTTP request made.
Certificate authentication happens at the TLS level on the service side using an authentication handler that validates the certificate service level for a given HTTP request.
In our last article, we learned multiple approaches to create HTTPClient requests using like,
It is very simple to use HttpClientHandler with regular or named or typed HTTPClient.
A certificate with HttpClient using IHttpClientFactory
If using HttpClient IHttpClientFactory then you can add a client certificate to HttpClientHandler.
HttpClientHandler gives you a property called ClientCertificates to set up the certificates.
This handler can then be used in a named instance of a HttpClient using the ConfigurePrimaryHttpMessageHandler method.
Specify client certificate manually
By default, the property “ClientCertificateOptions ” will be set as manual if not specified.
This means the application manually provides the client certificates to the RequestHandler.
ClientCertificateOptions = ClientCertificateOption.Manual
Example,
Below HttpClient used the manual “ClientCertificateOption.Manual” option and specify the certificate.
services.AddHttpClient("AccountClient", c => //Named Http Client
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("AccountURL"));
// Account API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/json");
}).
ConfigurePrimaryHttpMessageHandler(() =>
{
var handler = new HttpClientHandler();
/*specify your certicates here, if any*/
handler.ClientCertificates.Add(new X509Certificate());
return handler;
});
Specify HTTP client certificate Automatically
Property “ClientCertificateOptions ” if it is set to Automatic, HttpClientHandler will attempt to provide all available client certificates automatically and the certificate is automatically picked from the certificate store.
ClientCertificateOptions = ClientCertificateOption.Automatic
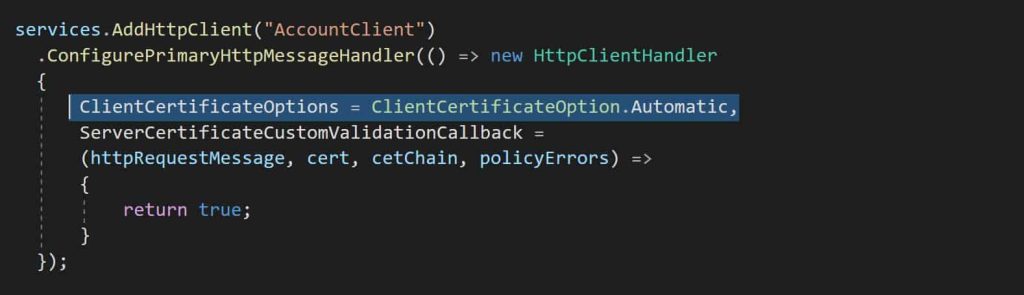
Kindly see the article Named HTTPClient on using the above-named HttpClient with a request example.
Additionally if needed you can multiple certificates using X509Certificate2Collection as below,
ConfigurePrimaryHttpMessageHandler(() =>
{
var handler = new HttpClientHandler();
var clientCertificates = new X509Certificate2Collection {/*specify your certicates here*/};
handler.ClientCertificates.AddRange(clientCertificates);
});
Certificate Validation Callback
If you need to handle any certificate validation callback event, please specify and register the callback event using ServerCertificateCustomValidationCallback as below,
ConfigurePrimaryHttpMessageHandler(() =>
{
var handler = new HttpClientHandler();
var clientCertificates = new X509Certificate2Collection {/*specify your certicates her*/};
handler.ClientCertificates.AddRange(clientCertificates);
handler.ServerCertificateCustomValidationCallback = ValidateServerCertificattion;
});
ServerCertificateCustomValidationCallback event is defined as below,

Regular HttpClient using a certificate and the HttpClientHandler
If you are using regular HttpClient using HttpClientHandler could be added directly in the constructor of the HttpClient class as shown in the below example.
Here is how I am setting up the HttpClient,
HttpClientHandler handler = new HttpClientHandler();
handler.ServerCertificateCustomValidationCallback = ValidateServerCertificattion;
_client = new HttpClient(handler);
try
{
HttpResponseMessage response = await _client.GetAsync("https://www.thecodebuzz.com/");
response.EnsureSuccessStatusCode();
string responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine($"Read {responseBody.Length} characters");
}
catch (HttpRequestException e)
{
Console.WriteLine($"Message: {e.Message} ");
}
That’s all! Happy coding!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
In today’s post, we saw how to configure certificate authentication for HttpClient requests. We looked at two approaches using regular HttpClient and HttpClient using IHttpClientFactory and configured it for certificate authentication.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.