Add Custom Headers to Response and Request in ASP.NET Core

In this article, we will see how to add custom headers to Response in ASP.NET Core
If you are interested to learn on how to add custom headers to Request then please refer to the below article:
Add Custom Header to Request in ASP.NET Core
Today in this article, we will cover below aspects,
Inline Middleware to add custom header in Response
Easily add a custom header to all ASP.NET Core API responses using the below lightweight middleware as discussed.
Please add the below code to your API pipeline by updating the Configure() method in Startup.cs
app.Use(async (context, next) =>
{
context.Response.Headers.Add("X-Response-Header", "Header-from-the-Pipeline");
await next.Invoke();
});
Below is the client receiving the API responses with the added custom headers.
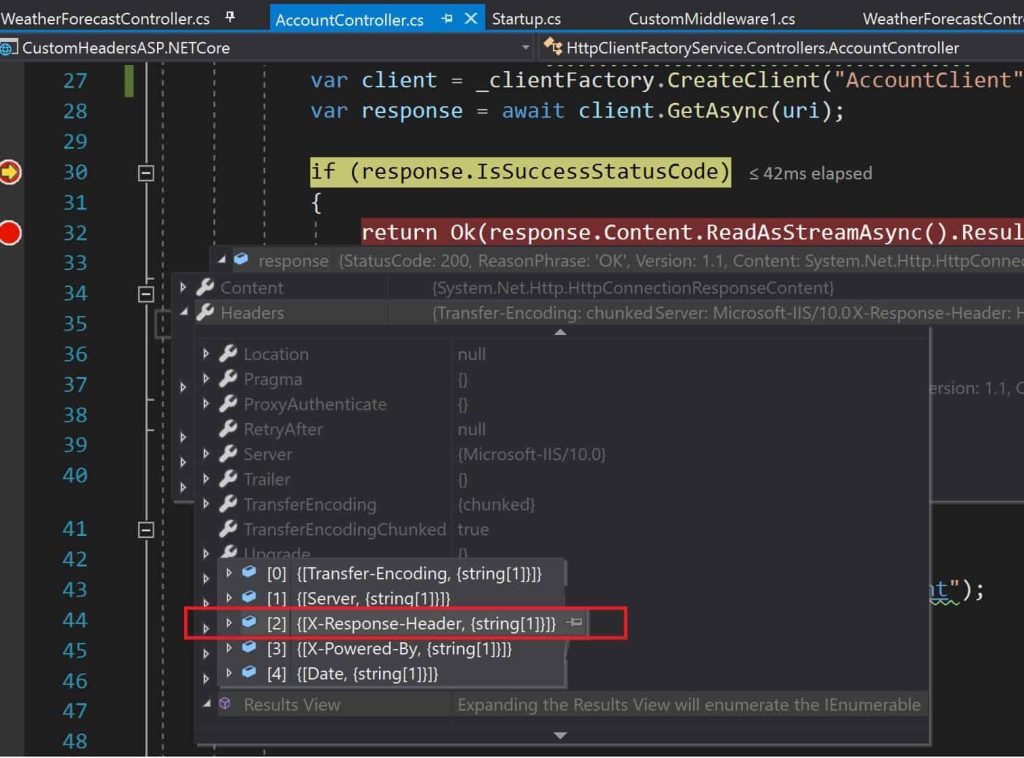
Custom Middleware to add a custom header in ASP.NET Core Response
You can also easily centralize sending headers to all API responses in using Custom Middleware in ASP.NET Core.
Here we will make use of httpContext.Response.OnStarting() delegate to achieve the same.
OnStarting() method here adds a delegate that invokes just before response headers are sent to the client.
Example:
public class CustomMiddleware
{
private readonly RequestDelegate _next;
public CustomMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task Invoke(HttpContext httpContext)
{
httpContext.Request.Headers.TryGetValue("thecodebuzz", out var traceValue);
if (string.IsNullOrWhiteSpace(traceValue))
{
traceValue = new Guid().ToString();
httpContext.Response.OnStarting((state) =>
{
httpContext.Response.Headers.Add("x-the-code-buzz", traceValue);
return Task.FromResult(0);
}, httpContext);
}
await _next(httpContext);
}
}
This way all clients will receive the API responses with the added custom headers.
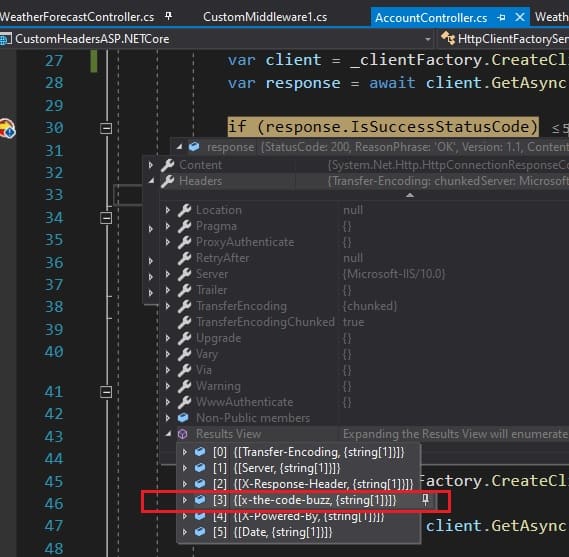
Both above-discussed middleware approaches are most preferable. However, you can also use Filters in ASP.NET Core.
Add Response Header in Controller
Adding custom headers through the controller is easier. Please use a Response object to add the required custom headers.
[HttpGet]
public IActionResult GetData()
{
///Your logic +
Response.Headers.Add("X-reponse-from-controller", "test123");
return Ok();
}
Or Use with HttpContext,
HttpContext.Response.Headers.Add("X-reponse-from-controller","test123");
That’s all ! Happy Coding !
References :
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Thanks , this was very helpful
Sally – Thanks for your inputs and comments !