How to call a Service with Basic Authentication in .NET/.NET Core
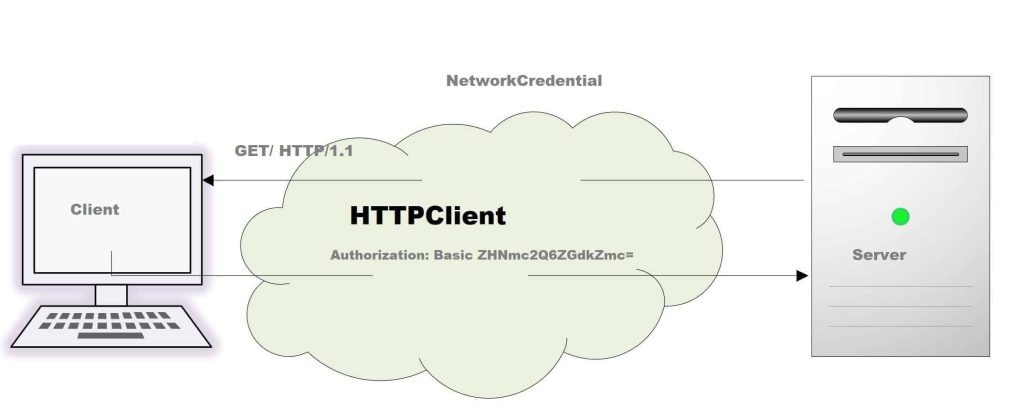
Today in this article, we will learn How to call a Service with Basic Authentication in the C# .NET/ASP.NET Core application.
Basic Authentication scheme transmits credentials like user ID/password encoded using the base64 string. We shall few below approaches for calling service with basic authentication.
There are multiple ways to connect to a service with Basic authentication.
Using HTTPClientFactory with Basic Authentication
If you are using HTTPClientFactory to create an HTTPClient request object then you can use Named HttpClient or Typed HttpClient to configure the Basic Authentication by using NetworkCredential.
Define ConfigurePrimaryHttpMessageHandler to add a delegate to configure the primary HttpClientHandler.
You can use NetworkCredential objects to credentials it to HttpClientHandler.
services.AddHttpClient("PayBillClient", c =>
{
c.BaseAddress = new Uri(Configuration.GetValue<string>("PayBillURL"));
// PayBill API ContentType
c.DefaultRequestHeaders.Add("Accept", "application/xml");
}).ConfigurePrimaryHttpMessageHandler(() =>
{
return new HttpClientHandler()
{
UseDefaultCredentials = true,
Credentials = new NetworkCredential(Configuration.GetValue<string>("UserName"),
Configuration.GetValue<string>("Password")),
};
});
As shown above, HttpClientFactory allows you to centralize the configuration for each HttpClient.
Ultimately above credentials like username and password might come from your Secrete Storage manager or Vault storage more precisely
Using HTTPClient( Regular) Handler for Basic Authentication
If using the general HttpClient request object, please define HttpClientHandler with the required NetworkCredential credentials.
For Basic authentication, it’s simple to use NetworkCredential objects to pass it to HttpClientHandler.
[HttpGet]
public IActionResult Get()
{
HttpClientHandler httpClientHandler = new HttpClientHandler()
{
Credentials = new NetworkCredential("username", "****"),
};
_client = new HttpClient(httpClientHandler);
HttpResponseMessage response =
_client.GetAsync("https://www.thecodebuzz.com/api/").Result;
return Ok(response.Content.ReadAsStringAsync().Result);
}
Here please define the HttpClient instance as a shared instance by declaring it as Static as below,
[Route("api/[controller]")]
[ApiController]
public class PaymentController : ControllerBase
{
private static HttpClient _client = new HttpClient();
...
}
Note: Using HttpClient static shared instance or instance injected through HttpClientFacotry is a good practice. Please visit for more guidelines on HTTPClient HttpClient Best Practices
Using the ‘Authorization’ Header in HTTP Request
The UserName and password as discussed above can be converted to Base64 string and can be passed using Authorization header as below,
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray));
Where byteArray is obtained using basic auth credentials i.e username and password,
Example:
var byteArray = Encoding.ASCII.GetBytes("TheCodeBuzz:password1234");
One can easily pass the Authorization header in the form of below,
“Authorization: Basic ZHNmc2Q6ZGdkZmc=”
Example:
curl -X GET "https://localhost:5001/api/Values" -H "accept: text/plain" -H "Authorization: Basic ZHNmc2Q6ZGdkZmc="

CURL command can be run using Bash CLI
[HttpGet]
public IActionResult GetAccountDetails()
{
var byteArray = Encoding.ASCII.GetBytes("user:password1234");
client.DefaultRequestHeaders.Authorization = new
AuthenticationHeaderValue("Basic",
Convert.ToBase64String(byteArray));
HttpResponseMessage response = client.GetAsync("https://www.thecodebuzz.com").Result;
return Ok(response.Content.ReadAsStringAsync().Result);
}
So with all of the above examples today we looked at almost 3 ways of passing Basic authentication credentials to the calling service.
That’s All! Happy coding !!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
References:
Summary
Today in this article we learned different techniques of calling service with Basic authentication. We looked at how to use HttpClientfactory for passing usernames and passwords. We also looked at basic HttpClient with HttpHandler and direct Authorization header usage for the same.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.