Log4Net File Logging in ASP.NET Core
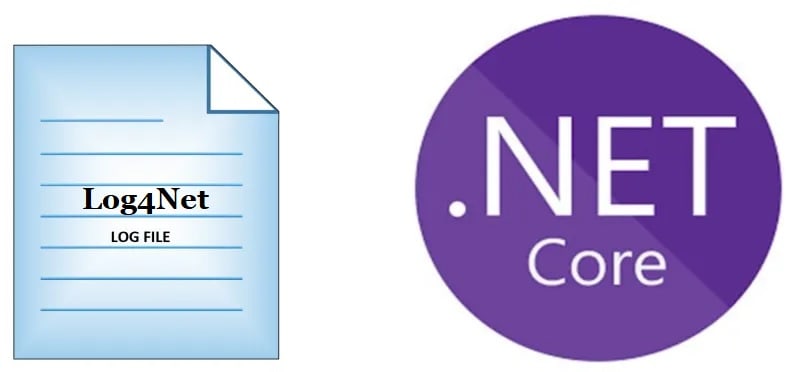
Today in this article, we will see how to perform File/Rolling File logging and Console logging using Log4Net in ASP.NET Core API application.
Today in this article, we will cover below aspects,
Logging in .NET Core 2.2 and .NET Core 3.1 + onwards framework is simplified further. In the last article, we saw how to Enable Logging in ASP.NET Core WebAPI using Built-in Providers.
We shall be leveraging DI( Dependency Injection) framework to inject the Log4Net logger object into the ASP.NET Core API pipeline.
Please note that the File/Rolling File logging provider is still not available through the .NET Core framework and we need to rely on external solutions.
Today we shall see how to use Log4Net to address the same requirement.
Microsoft recommends using a third-party logger framework like a Serlilog or Log4Net or NLog for other high-end logging requirements like Database or File/Rolling File logging.
In this post, we will understand, how to enable file/ rolling file logging in using Log4Net and do customization to existing behavior using an example.
Getting started
Create ASP.NET Core 3.1 or .NET 5 application,

Log4Net For ASP.NET Core is available through NuGet packages.
Please use the latest available version for the ASP.NET Core version.
Please add below NuGet Packages,
PM> Install-Package Microsoft.Extensions.Logging.Log4Net.AspNetCore -Version 3.1.0
Or
Please install the package through Nuget Package Manager,
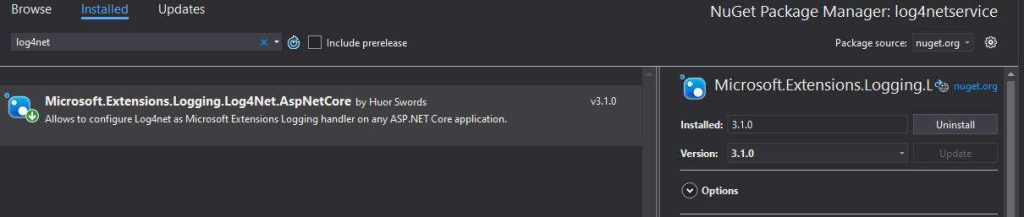
Update Main() method
Please update the Main method for adding the rolling file as shown in the below-highlighted code in Program.cs
Enable File Logging in ASP.NET Core
Update CreateHostBuilder() method for Log4Net as below using ConfigureLogging().
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
}).ConfigureLogging(builder =>
{
builder.SetMinimumLevel(LogLevel.Trace);
builder.AddLog4Net("log4net.config");
});
Let’s now log in to Controller using the regular ILogger Interface. Here below Controller uses the ILogger interface using Dependency injection.

Please see below the sample implementation of our Controller,
[Route("api/[controller]")]
[ApiController]
public class LoggingController : ControllerBase
{
private readonly ILogger _logger;
public LoggingController(ILogger<LoggingController> logger)
{
_logger = logger;
}
// GET api/values
[HttpGet("{id}")]
public ActionResult<IEnumerable<string>> Get(int id)
{
_logger.LogInformation("Start : Getting item details for {ID}", id);
List<string> list = new List<string>();
list.Add("A");
list.Add("B");
list.Add("C");
_logger.LogInformation($"Completed : Item details are { string.Join(", ", list) }");
return list;
}
Let’s execute the code and verify the generated file.
The log file will be created in the project directory and logging will be captured as shown below figure.
You can enable logging on any controller or any other layer of your API like the Business or domain layer easily. Also as shown above logging can be enabled on Startup.cs and Program.cs files as well.
Below is an example of file logging created by Log4Net,

Log4Net supports different types of logging using different types of logging appender. Please use the required appenders as appropriate.
File Logging Appender
If you wish to enable only the File/Rolling File logging appender, please use the File appender in the log4net file as explained below.
Sample log4net.config file used is as below,
<log4net>
<appender name="RollingFile" type="log4net.Appender.RollingFileAppender">
<file value="TheCodeBuzz.log" />
<appendToFile value="true" />
<maximumFileSize value="100KB" />
<maxSizeRollBackups value="2" />
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date %5level %logger.%method [%line] - MESSAGE: %message%newline %exception" />
</layout>
</appender>
<root>
<level value="TRACE" />
<appender-ref ref="RollingFile" />
</root>
</log4net>
What is a Rolling file?
By configuring the log file as rolling, you get the capability to create a logging file based on dates or file size or name format, and many more custom options. Your file keeps rolling as per provided criteria.
Console Logging Appender
If you wish to enable only Console logging with the customized format of log message please use the Console appender as below,
<appender name="Console" type="log4net.Appender.ConsoleAppender">
<layout type="log4net.Layout.PatternLayout">
<!-- Pattern to output the caller's file name and line number -->
<conversionPattern value="%date %5level %logger.%method [%line] - MESSAGE: %message%newline %exception" />
</layout>
</appender>
<appender-ref ref="ConsoleAppender" />
</root>
Console logging will be displayed on the console as below,

That’s all, Hope this article was helpful.
One can use the same concept for .NET Core Console/Desktop Application logging as well.
Summary
It’s very easy to configure file logging in ASP.NET Core using the Log4Net NuGet package. Log4Net helps us enable logging in a few simple steps and addresses the file-based and other types of logging requirements easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Great Example for an API based application
but any example for a windows service ?
Specifically where the service starts different classes and those classes need to log information on their own log (RollingFileAppender)
Perhaps using the ILoggerFactory ?
Hello Fidel – You can very much use the approach for Windows service using technique as explained here in this article for references Logging Using Log4Net in .NET Core Console Application
how about a simple console app version …
Hey Andy – Thanks for your query.Yes log4net very much can be used for Console app too. I have discussed an approach here. Hope this helps.