HTTP GET with Request Body Example in ASP.NET Core

In this article, we shall see an example of HTTP GET with the Request Body support in the ASP.NET Core application.
Please note that as per RFC 7231 specifications, I found the .NET Core framework has added support for the GET method with the Body parameter.
When I tested using ASP.NET Core3.1, a RESTFul HTTP GET with Request Body was successful. Request with [FromBody] parameters seems to prove it is possible to use this concept.
To verify the same, I simply created an ASP.NET Core application and verified this support.
Before using HTTP GET with request Body, please be cautious about the few limitation and issues associated with it. I have listed them in the guidelines section of this article in detail.
Create ASP.NET Core API
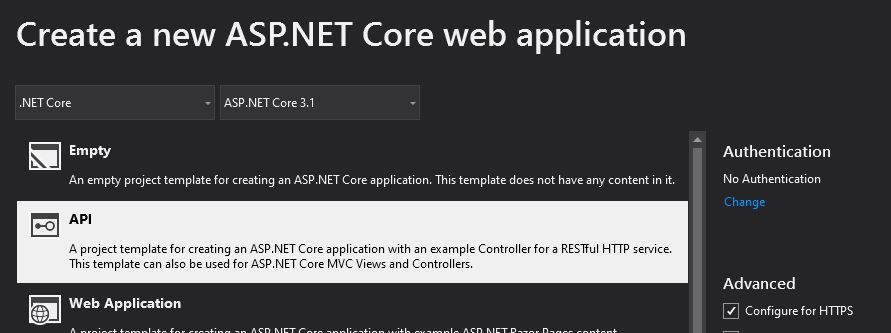
Please add the below method to your Controller implementation.
Using [FromBody] parameter in HTTP GET
Please note that we are able to send the [FromBody] parameter in HTTP GET Request input.
[HttpGet]
[AllowAnonymous]
public IActionResult Token([FromBody]LoginModel login)
{
if (login == null)
{
return BadRequest();
}
return Ok(login.Username);
}
Let’s execute the method from Fiddler UI.
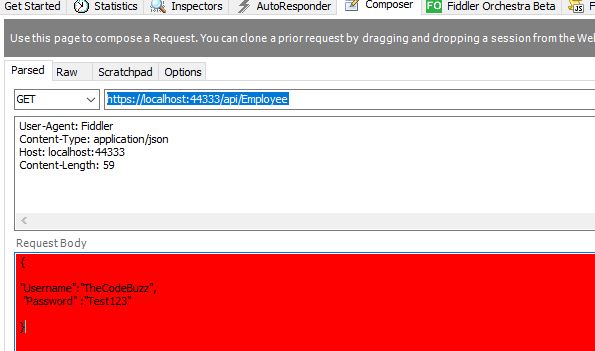
Fiddler returns us a successful response but also gives a warning while using the body in the GET request.
Here is the client-side sample code to execute the GET method with Body parameter just for example,
private static void CallGetMethod()
{
var login = new LoginModel();
login.Username = "TheCodeBuzz";
login.Password = "*******";
var json = JsonConvert.SerializeObject(login);
var request = new HttpRequestMessage
{
Method = HttpMethod.Get,
RequestUri = new Uri("https://localhost:44333/api/Employee"),
Content = new StringContent(json, Encoding.UTF8, "application/json")
};
var response = client.SendAsync(request).ConfigureAwait(false);
var responseInfo = response.GetAwaiter().GetResult();
}
Let’s debug the code with the above client call,

Finally, It proves that the GET method can be called with [FromBody] parameters sending larger data to the request.
HTTP DELETE with Request Body
A similar example also works for the HTTP DELETE method as well in ASP.NET Core.
[HttpDelete]
[AllowAnonymous]
public IActionResult DeleteEmployee([FromBody]LoginModel login)
{
if (login == null)
{
return BadRequest();
}
return Ok(login.Username);
}
However, as I mentioned above, there are a few guidelines before using this option.
Kindly see additional guidelines on using HTTP GET Request with body parameter in below article,
Reference: HTTP GET with Request body – Guidelines
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.