Serilog – File/Rolling File Logging in .NET Core Console Application
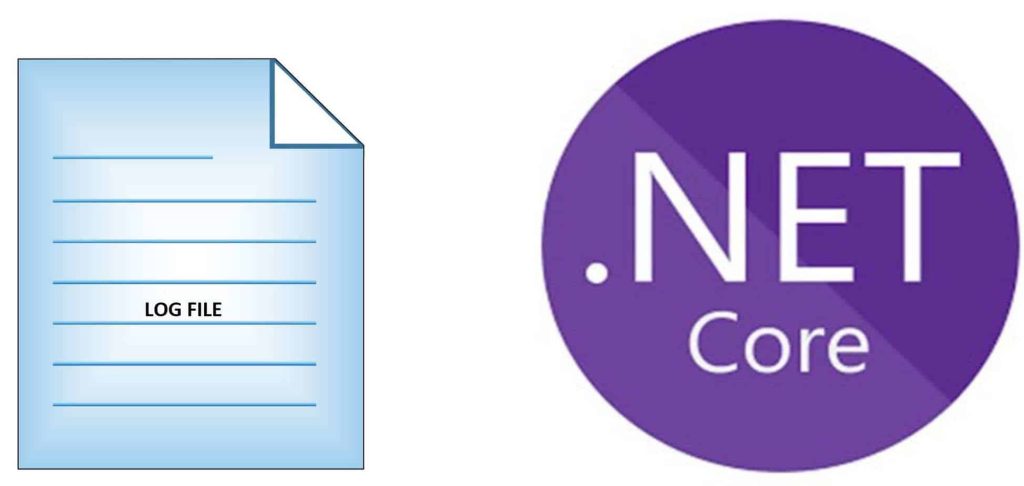
Today in this article, we will see how to do file/rolling file logging using Serilog in a .NET Core Console application.
In our last article, we already learned how to enable File logging for the ASP.NET Core API template using Serilog and NLog
We shall be leveraging the DI( Dependency Injection) framework to inject the Serilog logger object into the Console application pipeline.
Today in this article, we will cover below aspects,
Unlike ASP.NET Core the Console application doesn’t have dependency injection by default.
In ASP.NET WebAPI it was easy to configure and use ILogger through DI.
ILogger interface works very nicely with the .NET Core ecosystem and today in this post we will learn how to enable logging in to a .NET Core Console application.
File/Rolling file logging providers are still not available through the .NET Core Framework. We need to rely on external solutions for high-end logging requirements like file or database logging.
Microsoft recommends using a third-party logger framework like a Serilog or NLog and many other frameworks for other high-end logging requirements like Database or File/Rolling File logging.
Getting Started
Let’s create a .NET Core Console application
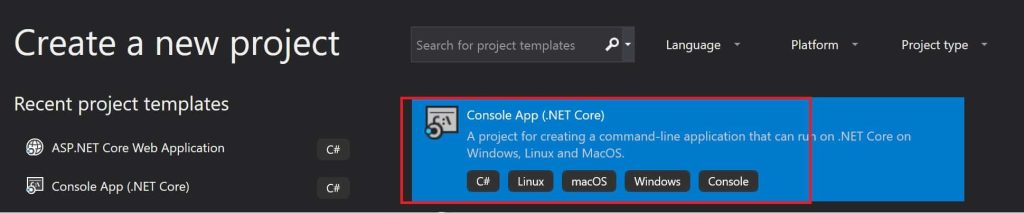
Here I have used the .NET Core 3.1 or .NET 5 Console application but the below technique will work in any lower version also.
Update the Main() method as below,
static void Main(string[] args)
{
var services = new ServiceCollection();
ConfigureServices(services);
using (ServiceProvider serviceProvider = services.BuildServiceProvider())
{
MyApplication app = serviceProvider.GetService<MyApplication>();
app.Run();
}
}
Above is a basic DI Container code which will help us in doing DI( Dependency Injection) of logger or business objects.
Please add below NuGet packages explicitly to your application.
- Microsoft.Extensions.DependencyInjection
- Microsoft.Extensions.Logging
- Serilog.Extensions.Logging
Add basic File Logging
Install-Package Serilog.Sinks.File -Version 4.1.0
private static void ConfigureServices(ServiceCollection services)
{
services.AddTransient<MyApplication>()
.AddScoped<IBusinessLayer, CBusinessLayer>()
.AddSingleton<IDataAccessLayer, CDataAccessLayer>();
var serilogLogger = new LoggerConfiguration()
.WriteTo.File("TheCodeBuzz.txt")
.CreateLogger();
services.AddLogging(builder =>
{
builder.SetMinimumLevel(LogLevel.Information);
builder.AddSerilog(logger: serilogLogger, dispose: true);
});
In the above code, we added logging and custom Startup MyApplication to the services collection and built the ServiceProvider for the required services which include the Serilog object and other business objects.
Add Rolling File Logging
If you need to enable rolling file logging please install below NuGet package.
Install-Package Serilog.Sinks.RollingFile -Version 3.3.0
Updated ConfigureServices method as below,
private static void ConfigureServices(ServiceCollection services)
{
services.AddTransient<MyApplication>()
.AddScoped<IBusinessLayer, CBusinessLayer>()
.AddSingleton<IDataAccessLayer, CDataAccessLayer>();
var serilogLogger = new LoggerConfiguration()
.WriteTo.RollingFile("Rolling-TheCodeBuzz.txt")
.CreateLogger();
services.AddLogging(builder =>
{
builder.SetMinimumLevel(LogLevel.Information);
builder.AddSerilog(logger: serilogLogger, dispose: true);
});
}
Implementation for class ‘MyApplication’ as below,
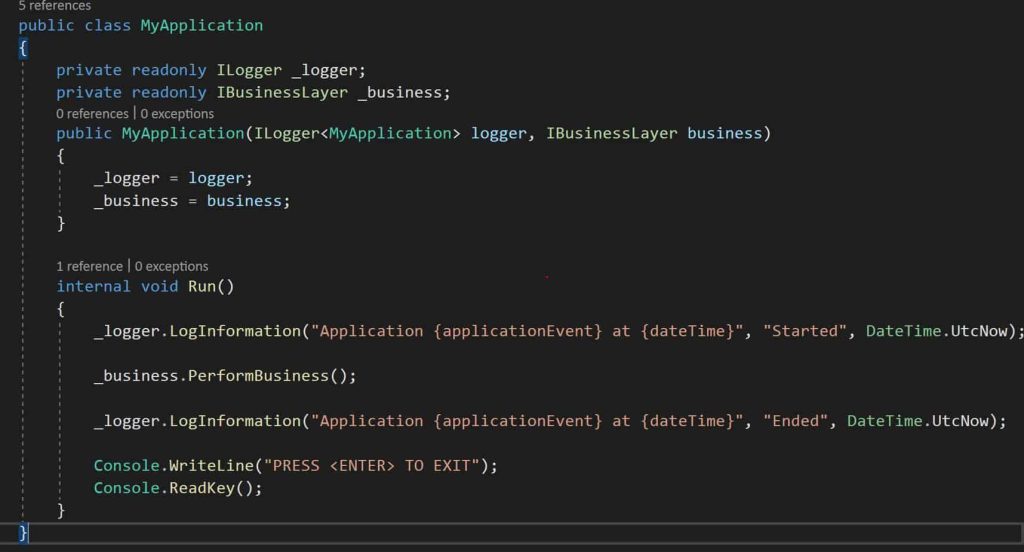
Let’s run the application and check the log file generated in a file.
Log files shall be generated in the app root folder itself by default (if the path is not specified).

Alternatively, you can also use NLog for Console Application file logging requirements.
That’s all, Hope this is helpful. Please sound off your comments below.
Summary
File/Rolling file logging provider is not yet available through the .NET Core framework and certainly, we need to depend on custom or any existing external solutions. Serilog helps us enable logging in a few simple steps and addresses the file-logging requirement easily.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.