Asynchronous Programming – Invoke Multiple Asynchronous and Combine Results
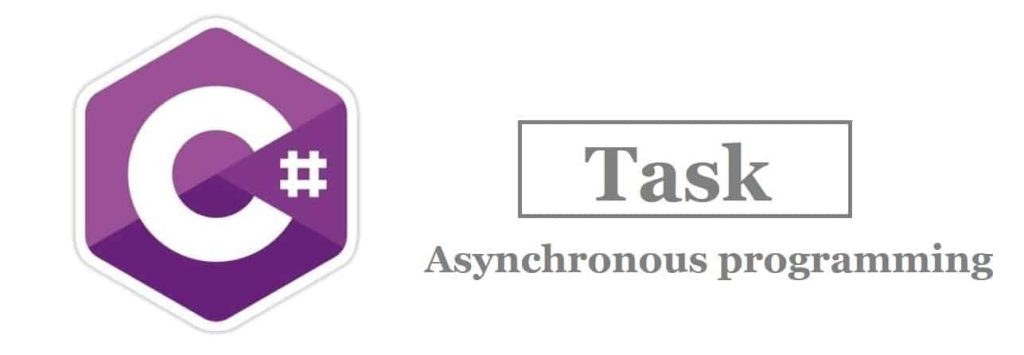
Today in this example we will learn how to perform C# .NET Multithreading – Invoke Asynchronous methods and Combine Results.
Asynchronous programming is an important programming technique that lets you perform multiple tasks at a time thereby increasing the throughput of your Application, API or Method, etc.
We will cover the below aspects in today’s article,
Getting Started – Multithreading with sync and async
Let’s take a simple example using a Console or Desktop application.
I have a requirement to invoke multiple service calls and combine their decisions to display to the user as if like a single operation.
However, the below concept discussed is generic enough and can be applied to ASP.NET or .NET Core (non-host) application business logic as well.
I have the below Ids to be processed asynchronously.
List<string> employeeIds = new List<string>
{
"101",
"102",
"103",
"104"
};
Each of above the IDs needs to be processed by calling an HTTP API method.
The below API processes the above IDs and provides the result,
private static EmployeeDetails GetEmployeeValues(string EmployeeID)
{
HttpResponseMessage response = client.GetAsync("https://localhost:44331/api/employees/" + EmployeeID).Result;
var reponseString = JsonConvert.DeserializeObject<EmployeeDetails>(response.Content.ReadAsStringAsync().Result);
return reponseString;
}
Let’s now see how to launch each request sequentially and parallelly and achieve the result.
Executing Synchronous method in C#.NET
I have a simple example where a service request takes a few seconds to execute.
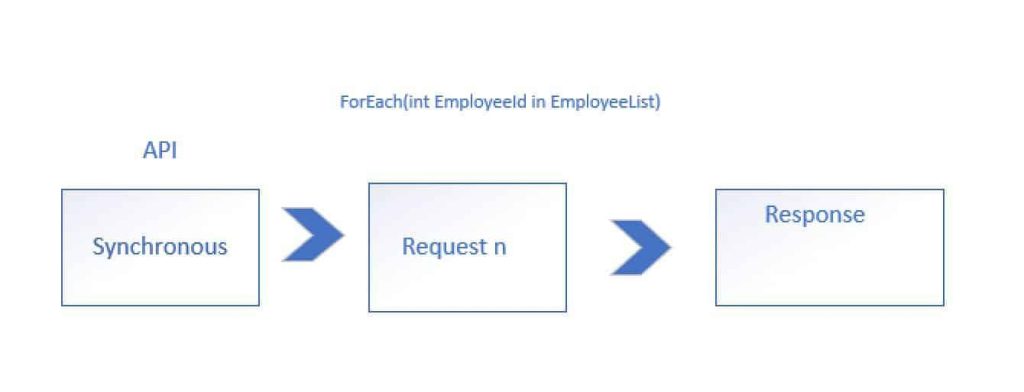
Each synchronous operation executes, so the total time required to complete the operation is equal to the sum of all requests processed operations.
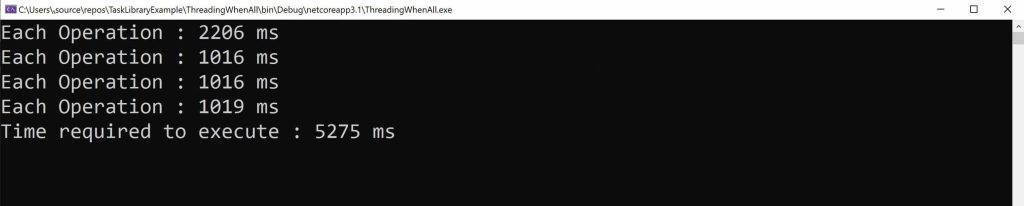
The total time required to complete all operations is ~ 5 ms
Below is the code base for Synchronous operation,

Asynchronous Task execution in C# .NET

Let’s declare the above operation using the Task library.
I have refactored the above GetEmployees() synchronous method as async as below,
private static async Task<EmployeeDetails> GetEmployeeValuesAsync(string EmployeeID)
{
HttpResponseMessage response = await client.GetAsync("https://localhost:44331/api/employees/"+ EmployeeID);
var reponseString = JsonConvert.DeserializeObject<EmployeeDetails>(response.Content.ReadAsStringAsync().Result);
return reponseString;
}
Let’s declare the Task query as below,
IEnumerable<Task<EmployeeDetails>> GetTaskQuery = from key in employeeIds select GetEmployeeValuesAsync(key);
We shall be using ToArray() to execute the query execution and get Employee Details.
Task<EmployeeDetails>[] completedTask = GetTaskQuery.ToArray();
Using Task.WhenAll
When used method asynchronously awaits multiple asynchronous operations, until all operations are completed.
It’s a very good means of handling a few collections of the Tasks and getting their results together as completion criteria.
Let’s use WhenAll to await the completion of all the running Tasks.
EmployeeDetails[] employeeList = await Task.WhenAll(completedTask);
Here below is the complete code,

Finally, the result is displayed below,

Each operation executes parallel and the maximum time taken by any operation would be the actual time taken by the whole operation. So all the API execution gets completed or content within the maximum time of one of the operations.
Increasing the load
Now even if I increase the request load by putting more request execution you will see the total time required would remain nearby the same time of execution.
However, this also depends on the CPU load at the time of code execution and also depends on the CPU used by the machine, and its hardware configuration

References:
That’s All.
Happy Coding!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Nice article . Could you provide a complete example?
Thank Sidhu. Glad you liked the article. Sure will share it asap. Thanks