Multithreading Async and Await methods – Asynchronous Programming
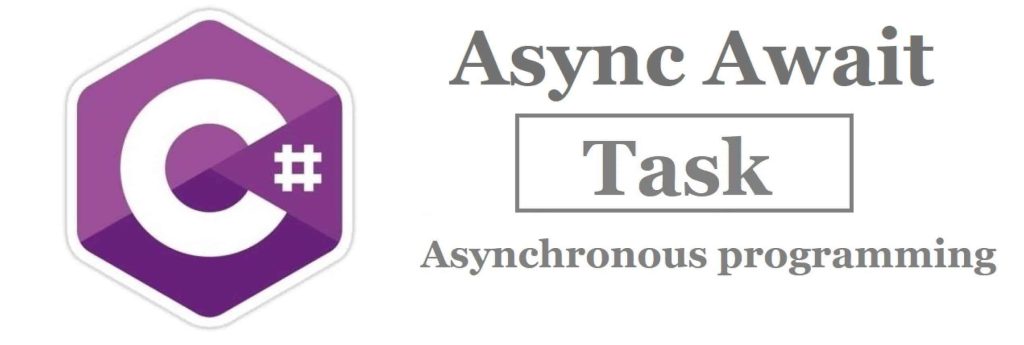
Today in this article, we will go over Sync Vs Async Multithreading – Asynchronous Programming concepts. Asynchronous programming is an important programming technique that lets you perform multiple tasks at a time thereby increasing the throughput of your Application, API, Services, Method, etc.
In this article, we shall cover below,
- Sync Vs Async differences – Synchronous method execution
- Each operation takes around 1000 ms and if you call this API for 4 Employees then the result is commutative ~ 5275 ms
- Sync Vs Async differences – Asynchronous method execution
- Each operation takes around 1800 ms. API for 4 Employees still results within ~2000 ms
- Execution Flow
- Thread Pooling and Task
- Guidelines- Multithreading
Asynchronous call executes in a nonblocking way without affecting other tasks, leveraging parallel processing, and providing multiple benefits including responsive UI design and experience.
You don’t really block your main thread, in fact, launch multiple tasks to perform logic execution in a non-blocking way.
Today in this article we shall see more examples to understand how and when to use async and await.
Today in this article, we will learn the basics of Async and Await keywords in C# programming and how to leverage them including some best practices on its usage.
Async and await usage are common in every CPU and I/O-bound operation. Example Restful API, UI – Winform or WPF, and most other types of applications.
To demonstrate, I have used a simple Windows Form with a button click event as below which executes sync and then async methods that return Employee details.
Form Button Click Example,
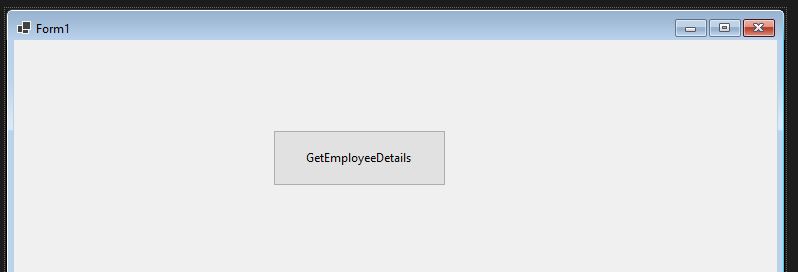
Sync Vs Async differences – Synchronous method execution
Below is the normal synchronous method defined. I shall be calling the below method to get the result and show the result on the console.
(Note: I got the result for the Winform app on the console by changing application type to Console application)
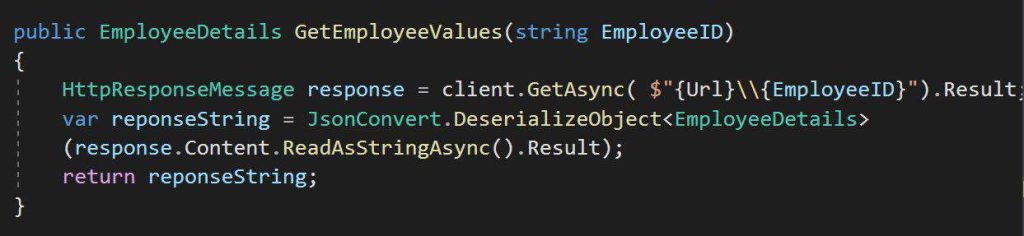
Here is the log captured for this API,

Each operation takes around 1000 ms and if you call this API for 4 Employees then the result is commutative ~ 5275 ms
It’s simple to understand, each method gets called synchronously and execute in sequence. It takes around 5 seconds to execute the operation.
Sync Vs Async differences – Asynchronous method execution
Below is a slight modification to make the API Asynchronous,

Let’s execute the Method again for 4 employees, below is the result,

That means we have simply improved results.
(For each method execution I have added the Timer to verify the result)
Each operation takes around 1800 ms. API for 4 Employees still results within ~2000 ms
Execution Flow

Thread Pooling and Task
A Thread pool is a great concept for sharing and recycling threads, allowing multithreading to be applied at a very granular level without a performance penalty.
All the Tasks are queued to the ThreadPool, which optimizes the number of threads and provides the proper load balancing to maximize throughput for any operations.
Guidelines- Multithreading
– Async keyword through the call stack
- Once you start using the Async operation at any part of your code, it should be set as async in the entire subsequent flow in your application.
- That mean’s call should be marked as async from the start of the async operation until the end to get the proper result.
- It’s important for each async method is combined with await operation to allow the nonblocking method execution.
- await stops the calling method and yields control back to its caller until the awaited task is complete.
– Void operations with the Async keyword
- A void return async function becomes a “fire and forgets” method.
- Async keyword propagation stops when your methods return voids
– Mark your event handler Async
- Mark your event handler delegate with the async keyword in a UI app
That’s All.
Happy Coding!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Other references:
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.