Bypass SSL Certificate in .NET – Guidelines
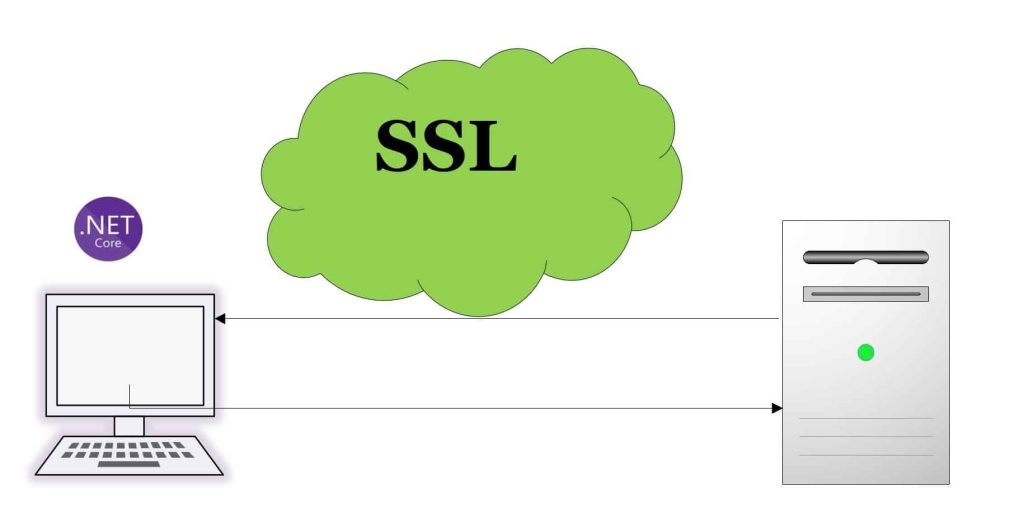
Today in this article, we will see how to enable Bypass SSL Certificate in ASP.NET Core or.NET framework (regular) based application.
This could be a very genuine use case for you and often useful in the lower environment, where you want to go ahead with your development or Test activity without having to worry about certificates.
Additionally, you may want to simply bypass invalid SSL certificates in the .NET Core application.
Today in this article, we will cover below aspects,
We will see how to enable SSL bypass DEV/TEST environment and how the below logic helped me resolve the issue.
The below solution could be a temporary workaround but it is often recommended to not bypass any certificates unless you know what you are doing and its impact.
Security issues are very important and small mistakes could cost you everything.
We shall see how to bypass certificates for the .NET core and regular .NET framework-based applications.
We shall be using below sample code for verifying this behavior.
If you are using regular .NET core, I have explained the same behavior below in detail.
[Route("api/[controller]")]
[ApiController]
public class AccountsController : ControllerBase
{
private readonly IHttpClientFactory _clientFactory;
public AccountsController(IHttpClientFactory clientFactory)
{
_clientFactory = clientFactory;
}
[HttpGet]
[Route("PersonalAccounts")]
public async Task<IActionResult> GetAccountDetails(int employeeId)
{
var client = _clientFactory.CreateClient("AccountClient");
var response = await client.GetAsync($"api/acounts/PersonalAccounts/{employeeId}");
if (response.IsSuccessStatusCode)
{
return Ok(response.Content.ReadAsStreamAsync().Result);
}
else
{
return StatusCode(500, "Something Went Wrong! Error Occured");
}
}
Enable SSL bypass using HTTPClient based on HttpClientFactory
ServerCertificateCustomValidationCallback helps you set the certificate validation flag easily.
By setting this delegate to true indicates that the certificate has passed validation.
services.AddHttpClient("AccountClient")
.ConfigurePrimaryHttpMessageHandler(() => new HttpClientHandler
{
ServerCertificateCustomValidationCallback = ValidateServerCertificattion
});
Below we are returning true for the callback which enables the bypass of any SSL validation,

Enable SSL bypass only for Dev/Staging environment,
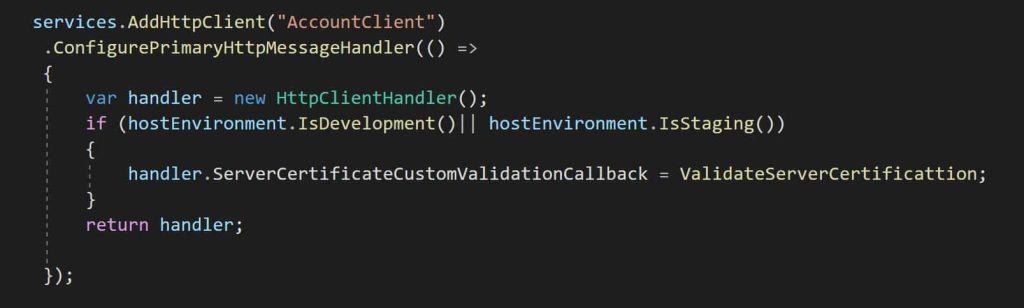
Note: Using HttpClientfactory gives you feasiblity of applying specific proerpty only for required clients instead of applying it at global level.
Alternatively, you use the below one-liner to enable SSL bypass,
ServerCertificateCustomValidationCallback =
(httpRequestMessage, cert, cetChain, policyErrors) =>
{
return true;
}
Enable SSL bypass using HTTPClient based on HttpClientHandler
If you are not using HttpClientFactory because of some reason, then please define the callback property as explained in the below article,

ByPass SSL certificate in the unsupported platform- iOS or Linux
As indicated above ServerCertificateCustomValidationCallback helps you set the certificate validation flag easily. One can set the delegate as true or false depending on the custom requirements.
Setting ServerCertificateCustomValidationCallback to true indicates that the certificate has passed validation.
But this callback option is not currently supported in iOS or Linux-based containerization of your application.
In such a platform, you might get errors like “PlatformNotSupportedException” indicating the error has occurred as the feature does not run on a particular platform.
In such scenarios, we can define certificate validation callback as below,
ServerCertificateCustomValidationCallback = HttpClientHandler.DangerousAcceptAnyServerCertificateValidator
Below are a few benefits of using delegate DangerousAcceptAnyServerCertificateValidator,
- Allows to set up appropriate delegate for the ServerCertificateCustomValidationCallback on the platform on iOS or Linux Based machines.
- Runtime SSL validation is disabled on the not supported platform.
- Avoid throwing PlatformNotSupportedException exception.
- Security is not an afterthought process and having this option will at least avoid accidental use by passing the SSL option in a higher environment like a Production environment.
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.