Read apsettings.json Configuration without dependency injection – Part II
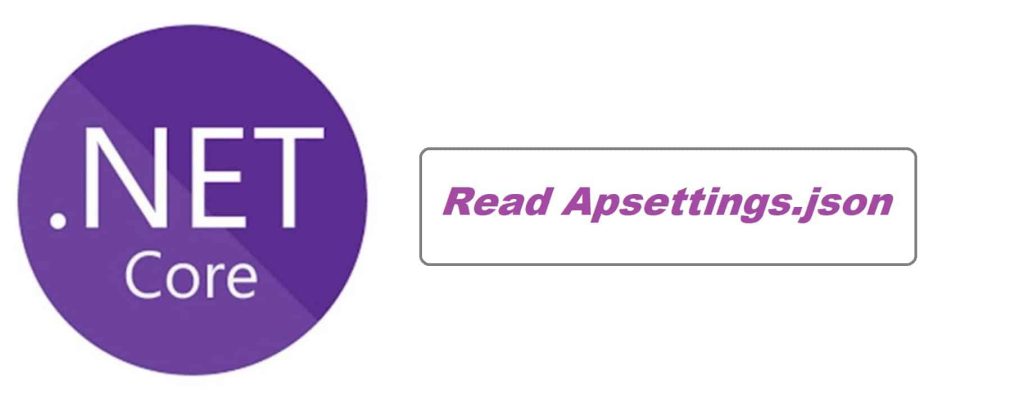
In this article, we shall see how to Read apsettings.json Configuration without dependency injection. We will read apsettings.json or .ini or XML or app.config etc without dependency injection(DI).
In our last article, we already learned how to load configuration from the apsettings.json file class library or external project (which could be.NET Standards or any other class library or DLL). We had leveraged the IoC container and Dependency Injection supported in the ASP.NET Core application.
Today in this article, we will cover below aspects,
Please refer to the below article for more details,
We shall be using the below appsettings configuration file to load the key-value pairs.
appsettings.json
{
"ServerURL": "https://localhost:44347/api/employee"
"Customer": {
"CustomerKeyurl": "https://www.thecodebuzz",
"CustomerdetailsUrl": "https://www.thecodebuzz",
"Agency": {
"AgencyID": "subvalue1_from_json",
"AccountKey": 200
}
}
}
Let’s use the Console application for this configuration loading.
However, this approach can also be used in the Class library or ASP.NET ( API or MVC) project template easily. It works fine for all the above project templates.
However, you should dispose of the configuration object additionally which is different from the DI approach where IoC takes care of the lifetime management of injected services.
Install NuGet package
dotnet add package Microsoft.Extensions.Configuration --version 6.0.1
Using ConfigurationBuilder to load the configuration
You can easily load the configuration using ConfigurationBuilder class. This class is used to build key/value-based configuration settings for use in an application.
Let’s see how to use it.
Step 1 – Create an instance of ConfigurationBuilder
Create an instance of ConfigurationBuilder class. Please use SetBasepath() method to set the base path so that configure the key-value pair according to the given environment.
Please add below the NuGet package called Microsoft.Extensions.Configuration to your application.
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile($"appsettings.{Environment.GetEnvironmentVariable("ASPNETCORE_ENVIRONMENT")}.json", optional: true);
Step2: Build the IConfiguration object
Builds a Configuration with keys and values from the set of providers registered in ConfigurationBuilder.Sources.
_configuration = builder.Build();
In the above code, we are setting the BasePath as the current directory using Directory.GetCurrentDirectory().
However, it’s not a good practice to use this directory method in some contexts. However, you can very much use the AppContext-supported method like,
Getting the correct directory in other project templates like test project etc might be different and there might also be some issues if using OS like Mac etc. This is just FYI.
Please add namespace using using Microsoft.Extensions.Configuration to use ConfigurationBuilder
In the above code, the _configuration object will be filled with the required configuration key and values from apsettings.json.
Once executed when you instantiate the above class in the Main() method or any other caller, the configuration will be set.
Once loaded configuration can however be DI further to the downstream usage as required.
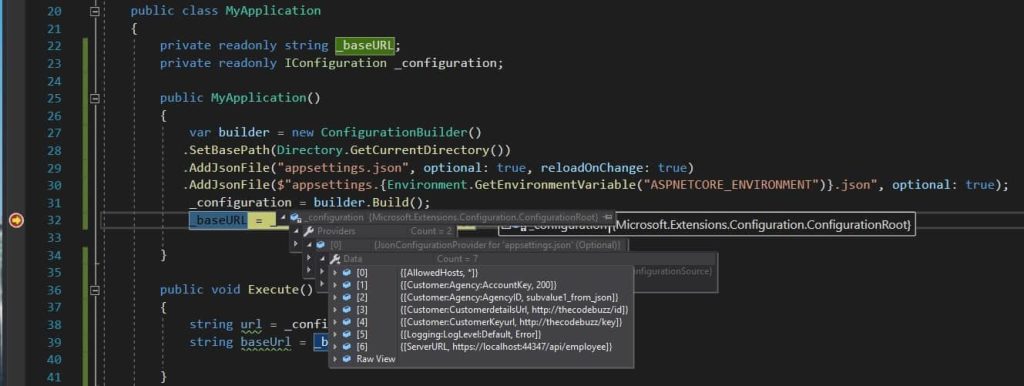
So with the above solution, it becomes very easy to resolve the basis required configuration.
Now whenever you create a new instance of MyApplication = new MyApplication() in a main() method or any other place, you shall get the required configuration settings automatically.
In host application types like ASP.NET Core CreateDefaultBuilder, a generic host builder in .NET and ASP.NET Core plays an important role in initializing the Host and its configuration out of the box using DI or IOC.
Please visit the article for more details: CreateDefaultBuilder and Configuration Management in .NET/ASP.NET Core
Loading XML, INI file Configuration
One can use the above solution to load the configuration available in other source files like XML, INI, or other JSON files, etc. as well.
Please refer to the below article,
Hope you find this article useful.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article, we looked at a possible solution for loading the configuration from the apsetting.json using the ConfigurationBuilder object. This solution works for any project like a class library or external project or ASP.NET app where you want to load application configuration without using DI.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
is the file appSettings.json cached after being loaded by ConfigurationBuilder? or Everytime we need to read the settings, the file should be loaded?
Hi Jamil – The above-discussed approach appSettings.json will be loaded in application memory based on way of instantiating “MyApplication” in the application. Ex. If using a singleton instance, it will be loaded once.