Instantiate DBContext in ASP.NET Core
Today in this article we shall see how to Instantiate DBContext in ASP.NET Core.
Today in this article, we will cover below aspects,
Instantiating DBContext in Entity Framework is important for establishing the connection and performing efficient CRUD operations on the datastore of your choice.
We shall see both approaches i.e using DbContext with Dependency Injection and without Dependency Injection.
Please note that the use of DbContext using a dependency injection container is recommended.
This ensures that DbContext is created as per request by the API pipeline and disposed of based on lifetime management used while registering it.
Please configure your DBContext as below in ASP.NET Core API,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddDbContext<EmployeeContext>(options =>
{
options.UseSqlServer(Configuration.GetConnectionString("EmployeeDB"));
});
}
Instantiating DBContext in Entity Framework using DI
Once you configure your DBContext as above in ASP.NET Core API, once can use DI and inject the required DBContext class and instantiate it.
public class EmployeeDetailsController : ControllerBase
{
private readonly EmployeeContext _employeeContext;
public EmployeeDetailsController(EmployeeContext context)
{
_employeeContext = context;
}
[HttpPost]
public async Task<ActionResult> Post([FromBody] EmployeeDb value)
{
try
{
if (ModelState.IsValid)
{
_employeeContext.Add(value);
await _employeeContext.SaveChangesAsync();
}
}
catch (Exception)
{
return StatusCode(StatusCodes.Status500InternalServerError, "Internal Server Error");
}
return Ok(value);
}
}
Above a similar technique of constructor injection, you can use it in any module or class. For example, below is an example of instantiating DBContext in the Repository class.
public class EmployeeRepository : IEmployeeRepository
{
private readonly EmployeeContext _context;
public EmployeeRepository(EmployeeContext context)
{
_context = context;
}
public IEnumerable<Employee> GetEmployees()
{
return _context.Employee.ToList();
}
.
.
.
}
Please visit the below article for more details:
Using DBContext without Dependency Injection
If you do not want to use DI, it is pretty straightforward to use DBContext anywhere in the project.
Above IoC usage of DBContext takes care of creating DBContext as and when needed. Framework-based IoC containers also dispose of those objects when their usage is done.
Below example, EmployeeContext DbContext instantiated within the ‘using‘ statement as it becomes your responsibility to dispose of such instances.
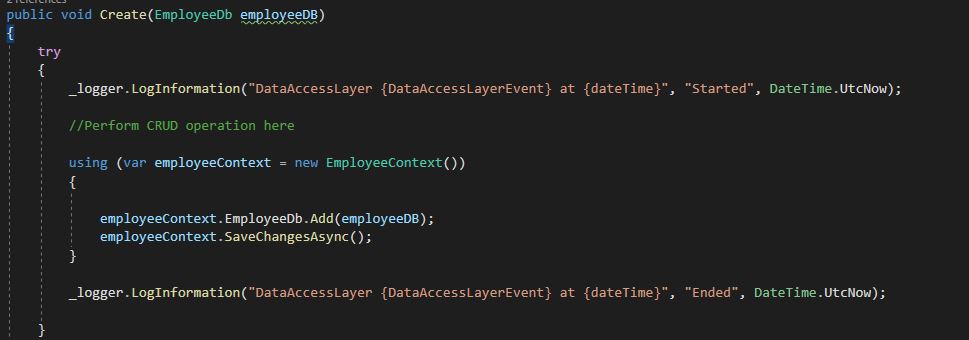
Additional References :
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.