Getting Started with Entity Framework – ORM in ASP.NET Core

In this article, we will see how to get started using the Entity Framework – ORM in ASP.NET Core using the SQL database in ASP.NET Core applications.
We shall be using the Database First approach where I shall be using the existing SQL database to scaffold the required entities.
We shall keep this article very simple and will try to consume DBContext within the Controller however one can additional repository pattern to abstract all DB interaction logic to a commonplace if needed as recommended.
Entity Framework is an ORM-Object-relational mapping framework that helps to represent the Database into the object-oriented programming model in the .NET Core ecosystem helping to interact and perform CRUD operation on relational DB without hassle.
It’s also one of the good tools representing the modern initiative of the various enterprises towards moving away from Stored procedure(SP) based CRUD operation on Database as a possible use case.
EFCore brings a higher level of abstraction and helps in creating data-oriented applications with less code and in an efficient way.
Getting Started
Create an ASP.NET Core service
Please use ASP.NET Core 3.1 or .NET 6 framework as required.

Add EFCore Nuget package
Please add the EFCore driver NuGet package.
Using the NuGet Package manager Console,
PM> Install-Package Microsoft.EntityFrameworkCore.SqlServer -Version <version>
Note – Please use the latest available version
Additionally, please install below NuGet packages,
Example,
PM> Install-Package Microsoft.EntityFrameworkCore -Version 3.1.3
PM>Install-Package Microsoft.EntityFrameworkCore.Design -Version 3.1.3
PM> Install-Package Microsoft.EntityFrameworkCore.SqlServer -Version 3.1.3
We are all set with the setup, let’s now start using the existing database and scaffold it using EFCore
Database First – Scaffolding for Existing Database
Below is the database schema we shall be used for performing CRUD operation,

SQL Database Schema
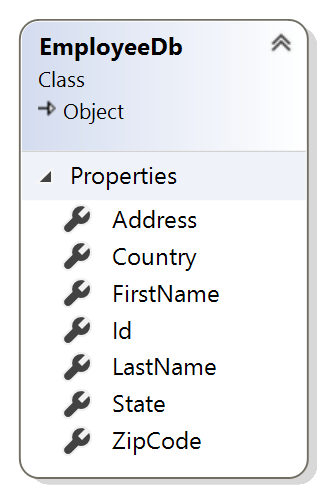
SQL Connection Configuration
Below is the SQL DB connection configuration defined in appsettings.json.
Connection string could be also be stored in an environment variable or Config Server or Secrete storage if any available.
We shall be using the Dependency Injection technique using IConfiguration or IOption interface to read configuration details.
{
"SQLConnectionSettings": {
"ConnectionString":
"Server=< your database host> ;Database=master;Trusted_Connection=True;",
}
}
Scaffolding SQL Database using EFCore
Example:
Scaffold-DbContext “Server=localhost\SQLEXPRESS;Database=master;Trusted_Connection=True;” Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -Tables “EmployeeDB” -ContextDir Context -Context EmployeeContext
After successful command, you shall scaffold DB as below,
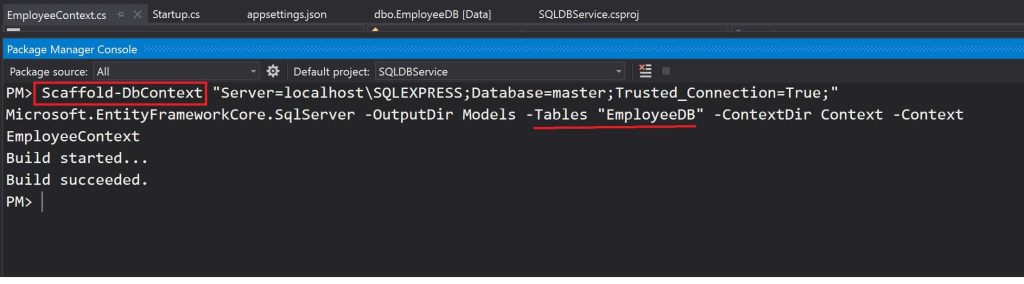
Reference: Scaffolding commands in the EFCore
Below is the Database schema model class created after running the above query,
public partial class EmployeeDb
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string Address { get; set; }
public string ZipCode { get; set; }
public string State { get; set; }
public string Country { get; set; }
public string Id { get; set; }
}
Update ConfigureServices for DBCOntext
Update ConfigureServices() method as below,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddDbContext<EmployeeContext>(options =>
{
options.UseSqlServer(Configuration.GetConnectionString("EmployeeDB")
});
}
Dependency Injection(DI) DBContext
We shall now use EmployeeContext through Constructor Injection as below,
public class EmployeeDetailsController : ControllerBase
{
private readonly EmployeeContext _employeeContext;
public EmployeeDetailsController(EmployeeContext context)
{
_employeeContext = context;
}
..
..
}
Performing CRUD operation
Let’s now perform the CRUD operation on the database. we shall first do the GET Operation.
Here for simplification, I am using context object directly in Controller API. But as a good practice, you can have layered architecture using a repository or another layer as required.
[HttpGet("{id}")]
public async Task<IActionResult> OnGetAsync(int? id)
{
if (id == null)
{
return NotFound();
}
EmployeeDb db = await _employeeContext.EmployeeDb.FindAsync(id);
if (db == null)
{
return NotFound();
}
return Ok(db);
}
Let’s now perform the POST method,
[HttpPost]
public async Task<ActionResult> Post([FromBody] EmployeeDb value)
{
try
{
if (ModelState.IsValid)
{
_employeeContext.Add(value);
await _employeeContext.SaveChangesAsync();
}
}
catch (Exception)
{
return StatusCode(StatusCodes.Status500InternalServerError, "Internal Server Error");
}
return Ok(value);
}
In a similar way, other CRUD operations like Delete or Update can also be defined.
EFCore scaffolding as Repository and UoW
Using tools like EFCore gives us DBContext which represents repository and UoW(Unit Of Work) implementation with very minimal effort.
Please see the below article for more details,
That’s all!
This was very much basic on how to get started with Entity Framework Core in ASP.NET Core application.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to connect to the SQL Relation DB database using the Entity Framework – ORM in ASP.NET Core. We also looked at simple and easy-to-understand ASP.NET Core implementation performing basic CRUD operations.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.