Using Neo4j Database in a C# Console .NET application

In this post, we shall learn how to use the Neo4j database in a Console C# application using .NET or .NET Core.
We shall be leveraging Generic HostBuilder to register the Neo4j driver interface in the small IoC container and then use it to perform CRUD operations on the database.
We shall also see how to consume Neo4j objects without Dependency injection (DI) in the Console application.
We already looked at how to Use a Generic host builder for Dependency injection in .NET Core console applications.
Neo4j is an open-source, NoSQL, native graph database that defines a set of nodes and the relationships that connect them.
Today in this article, we will cover below aspects,
Let’s look into how to use generic HostBuilder to create DI Container and inject the required dependency within the Console app .NET Core application.
Getting started
Lets create .NET Core 3.1 or .NET 5 Console application,
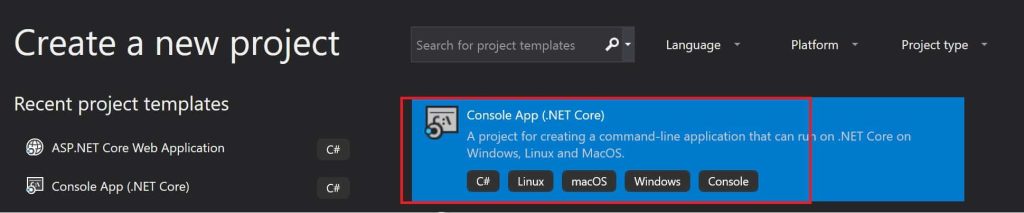
As references, this implementation more revolves around the approach I discussed in my previous article. Please refer to the below article,
Creating Generic HostBuilder
The HostBuilder class is available from the following namespace,
using Microsoft.Extensions.Hosting;
HostBuilder implements the IHostBuilder interface.
Please install the NuGet package from Nuget Package manager or PMC,
PM> Install-Package Microsoft.Extensions.Hosting -Version 3.1.1
Create DI Container and Initialize Host
Please create Generic HosBuilder and register the dependencies that need to be injected inject.
These changes can be implemented in the Main() method.
var builder = Host.CreateDefaultBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddTransient<MyApplication>();
services.AddSingleton(GraphDatabase.Driver("bolt://localhost:7687", AuthTokens.Basic("test", "test")));
}).UseConsoleLifetime();
var host = builder.Build();
MyApplication class is the entry point for all business functionalities which use the Neo4J interface within.
Initialize the CreateScope
Please add the below code in the Main() method,
using (var serviceScope = host.Services.CreateScope())
{
var services = serviceScope.ServiceProvider;
try
{
var myService = services.GetRequiredService<MyApplication>();
var result = await myService.Run();
Console.WriteLine($"Persons Names are {string.Join(", ", result)}");
}
catch (Exception ex)
{
Console.WriteLine("Error Occured");
}
}
Below is the class MyApplication executing the business workflow.
We are injecting logger and Neo4J IDriver objects as defined and registered in the scope using the HostBuilder DI container.
public class MyApplication
{
private readonly Microsoft.Extensions.Logging.ILogger _logger;
private readonly IDriver _driver;
public MyApplication(ILogger<MyApplication> logger, IDriver driver)
{
_logger = logger;
_driver = driver;
}
.
..
..
}
Lets define the Execute method as below,

The above method does the following,
- Uses the Neo4J driver instance and create a session.
- Using the session object executes a query.
- The query returns the top 10 personas names from the given Neo4j database.
- Close the session.
Let’s execute the application and verify the result.

Using Neo4j without Dependency Injection
You can use the Neo4J driver instance directly as below but it’s recommended to use only a singleton instance of the driver.

- References: Getting Started with Neo4j
That’s all! Happy coding.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
In this post, we learned how to use the Neo4j graph database in a Console application in the .NET Core. We learned how to execute simple and easy-to-understand queries and perform CRUD operations.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.