Getting Started with Neo4j GraphDB in C# ASP.NET Core
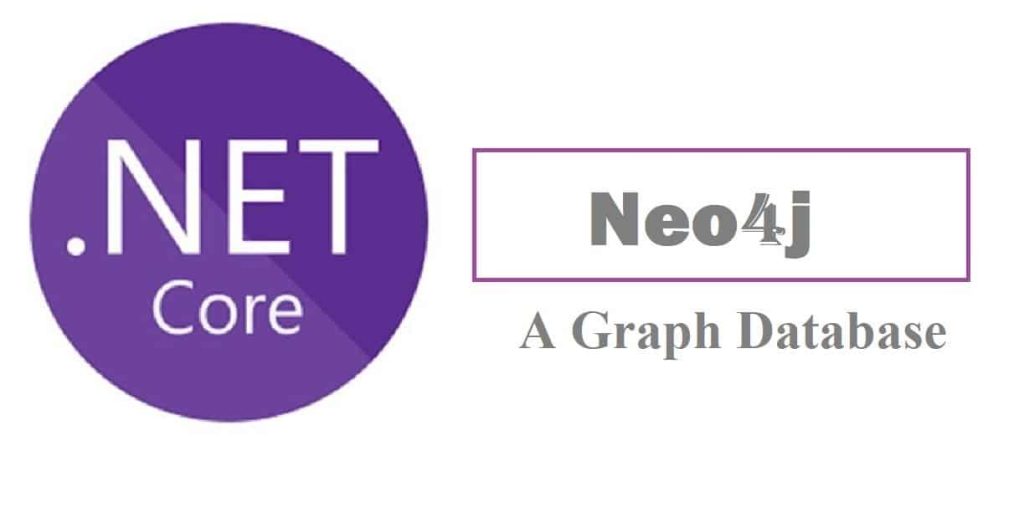
In this article, we will see how to get started using the Neo4j in C# using ASP.NET Core applications with simple and easy-to-understand steps.
We shall keep this article very simple and will try to consume Database Context within the Controller directly however you can address the separation of concern and fit in this model using a repository pattern to abstract all DB interaction logic to a commonplace if needed as recommended.
Today in this article, we will cover below aspects,
- Getting Started
- Create an ASP.NET Core API
- Add Neo4j Bolt Driver Nuget package
- Schema Models for Database
- Neo4j Connection Configuration
- Update ConfigureServices for Neo4j Driver – Dependency Injection
- Database credentials
- Register IDriver and Perform Dependency Injection (IoC)
- Depedency injection- Neo4j bolt IDriver in Controller
- Performing CRUD operation on Neo4j
- Query
- Using Neo4j driver without Dependency injection
- Neo4j using Neo4jClient Cypher
- Neo4j using Repository
- Summary
Neo4j is an open-source, NoSQL, native graph database that defines a set of nodes and the relationships that connect them.
Graphs represent entities as nodes and those entities relate to the world as relationships.
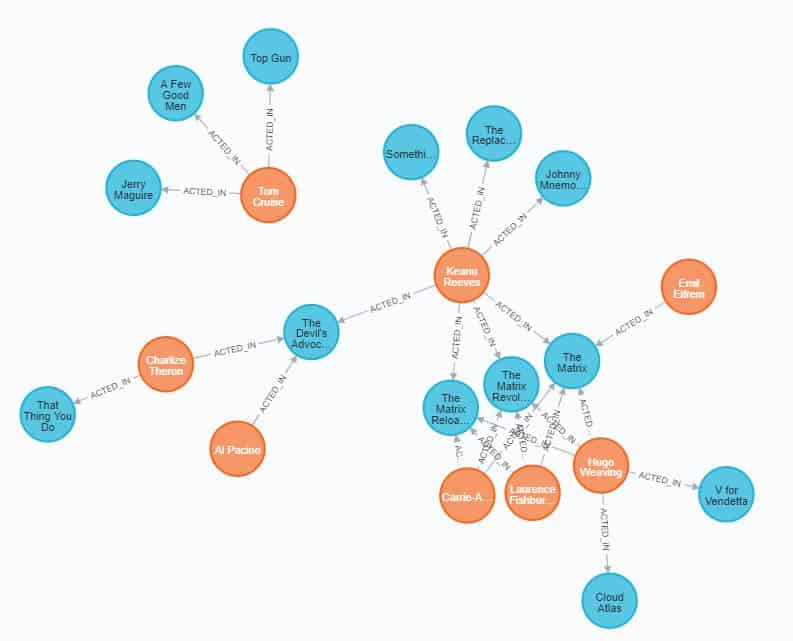
Getting Started
I shall be using the existing database to demonstrate Neo4j usage in ASP.NET Core API
Create an ASP.NET Core API
Here I have used ASP.NET Core 3.1 or .NET 5 framework.

Add Neo4j Bolt Driver Nuget package
Please add the Neo4j driver NuGet package.
Using the NuGet Package manager Console,
PM> Install-Package Neo4j.Driver -Version 4.1.0
OR
Using .NET CLI
dotnet add package Neo4j.Driver --version 4.1.0
We are all set with the setup, let’s now start using the existing database and connect it using the Neo4j driver.
Schema Models for Database
Below is the database schema we shall be using to perform CRUD operations.
Here I am using the existing database called “Movie Graph” and created corresponding model classes based on the database schema using the Database first approach.
Database Schema

Neo4j Connection Configuration
Below is the Neo4j connection configuration defined in appsettings.json.
Connection string recommended being stored in an environment variable or Config Server or Secrete storage if any available.
We shall be using the Dependency Injection technique using IConfiguration or IOption interface to read configuration details.
"NeO4jConnectionSettings": { "Server": "bolt://yourhost:7687"", "UserName":"Neo", "Password":"****8" }
Update ConfigureServices for Neo4j Driver – Dependency Injection
Location of Database:
I have a local existing database which is in general accessible using URL –“bolt://localhost:7687”
You can check the connection configuration using Neo4j desktop client if using,

Database credentials
After database configuration, you can use an existing user with the name “Neo4j“
Please make sure to use the correct username and password in the Basic method.
AuthTokens.Basic([Username], [Password])
Register IDriver and Perform Dependency Injection (IoC)
Update ConfigureServices() to inject Neo4j driver interface IDriver in the API pipeline as below,
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSingleton(GraphDatabase.Driver("bolt://localhost:7687", AuthTokens.Basic("Neo", "neo")));
}
As shown above, I have used the driver instance as Singleton. That means the same driver instance will be used for every request from the API.
Above I have hardcoded the connection string but ideally, you can use IConfiguration or IOption interface to read configuration or use it from any centralized storage.
Please see here for the implementation of IConfiguration using the Neo4j driver.
Depedency injection- Neo4j bolt IDriver in Controller
We shall now use IDriver instance through Constructor dependency Injection as below,

[ApiController]
[Route("[controller]")]
public class MovieController : ControllerBase
{
private readonly ILogger<MovieController> _logger;
private readonly IDriver _driver;
public MovieController(ILogger<MovieController> logger, IDriver driver)
{
_logger = logger;
_driver = driver;
}
..
..
}
Performing CRUD operation on Neo4j
Let’s perform GET Operation using the Neo4j driver instance. To keep it simple, I have the driver instance directly in the Controller. However one can look for Repository implementation for actual implementation.
Query
We shall be using the session object to get the list of names using node Person and printing the result for 10 person names,
cursor = await session.RunAsync(@"MATCH (a:Person) RETURN a.name as name limit 10");
Below is the complete implementation for the Get or Reading record.

Using Neo4j driver without Dependency injection
If you are not using Dependency injection, then the driver instance can be created directly as shown below.
IDriver driver = GraphDatabase.Driver("neo4j://localhost:7687", AuthTokens.Basic("test", "password")); .. //Perform create or read or update or delete on DB .. await driver.CloseAsync();
Note: If creating the instance without DI framework then you must dispose of it explicitly.
Let’s perform a READ operation.

As you can see above, we are able to read 10 movie actor names successfully!
The above similar way other CRUD operations like Create, Delete, or Update can also be defined.
Neo4j using Neo4jClient Cypher
You can connect the Neo4j graph database using another interface called Neo4jClient. Neo4jClient is a .NET client helping you to write Cypher queries with built-in IntelliSense support
Please see the below article for more details,
Neo4j using Repository
You can use the Repository pattern to abstract the Database operation and address the Database query in a more generic way.
Please see the below article for more details,
That’s all!
This was very much basic on how to get started with Neo4J in ASP.NET Core API application.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to connect to the Neo4j Graph Database. We looked at simple and easy-to-understand ASP.NET Core implementation performing basic CRUD operation on Neo4j database.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
Thanks.Very helpful Exactly what I need for my API.
How do you render the graph (circles and connecting lines) in C# ?
Hey Verum- Thanks for your query. Those are actually graph nodes that are available to view on the Neo4j Desktop dashboards.