Using Graph Neo4jClient Cypher query in C# ASP.NET Core
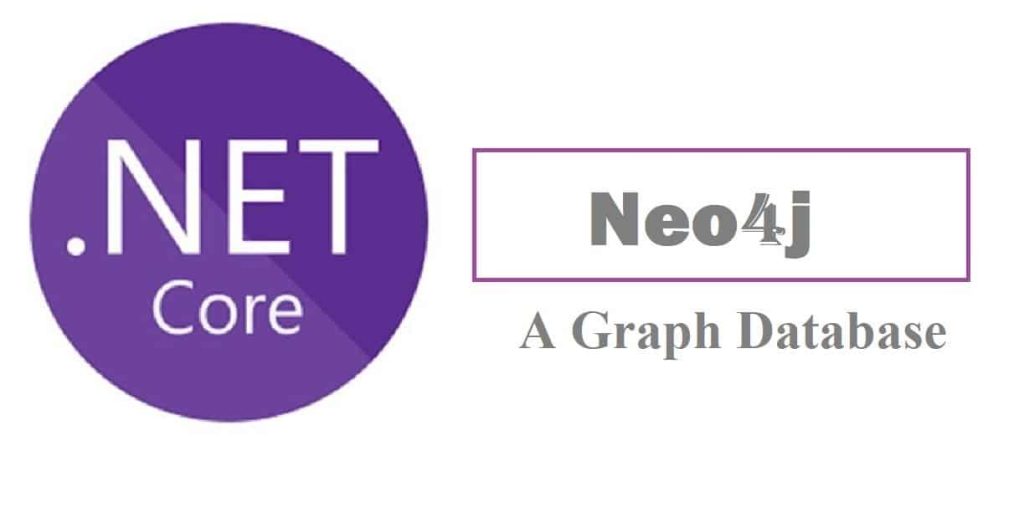
In this article, we will see how to use Neo4j Graph Cypher query using Neo4jClient within .NET examples.
We will look into Graph Neo4jClient Cypher query in C# ASP.NET Core with simple and complex queries with built-in IntelliSense support.
Today in this article, we will cover below aspects,
- Getting Started
- Create an ASP.NET Core API
- Add Neo4jClient Nuget package
- Schema Models for Database
- Neo4j Connection Configuration
- Connection Configuration NeO4j Driver DI
- Database credentials
- Register IGraphClient in API IoC Container
- Dependency Injection IGraphClient in Controller
- Performing CRUD operation on Graph Database
- Graph Cypher Query
- If not using Dependency injection
- Neo4j using Neo4j Bolt Driver
- Neo4jClient using Repository pattern
- Summary
In our last article on Getting started with Neo4j, we learned how to use the Neo4j Bolt driver to connect to the database and perform CRUD operations.
On a similar line, We shall keep this article very simple and will try to consume Neo4jClient within the Controller directly.
However, you can address the separation of concern and fit in this model using a repository pattern to abstract all DB interaction logic to a commonplace if needed as recommended.)
Neo4j is an open-source, NoSQL, native graph database that defines a set of nodes and the relationships that connect them.
Graphs represent entities as nodes and those entities relate to the world as relationships.

Getting Started
I shall be using the existing database to demonstrate Neo4j usage in ASP.NET Core API
Create an ASP.NET Core API
Here I have used ASP.NET Core 3.1 or .NET 6 framework.

Add Neo4jClient Nuget package
Please add the Neo4jClient NuGet package.
Using the NuGet Package Manager Console,
PM> Install-Package Neo4jClient -Version <version>
OR
Using .NET CLI
dotnet add package Neo4jClient --version <version>
We are all set with the setup, let’s now start using the existing database and connect it using the Neo4j driver.
Schema Models for Database
Below is the database schema we shall be using for performing CRUD operations.
Here I am using the existing database called “Movie Graph” and created corresponding model classes based on the database schema using the Database first approach.
Database Schema
Please create C# model entities as per the schema as below,

Neo4j Connection Configuration
Below is the Neo4j connection configuration defined in appsettings.json.
Connection string recommended being stored in an environment variable or Config Server or Secrete storage if any is available.
We shall be using the Dependency Injection technique using IConfiguration or IOption interface to read configuration details.
"NeO4jConnectionSettings": { "Server": "http://yourhost:7474", "UserName":"Neo", "Password":neo }
Connection Configuration NeO4j Driver DI
Location of Database:
I have a local existing database which is in general accessible using URL –
For HTTP Graph DB connection use port 7474
http://yourhost:7474 Or For secured HTTPS Graph DB connection use port 7473 https://yourhost:7473
You can check the connection configuration using the Neo4j desktop client if using,
How to view the HTTP configuration address for NeO4j
If you have access to the Neo4j desktop application then you can see the HTTP address for the database.
Please go to your Project -> Manage – > Details section to view the HTTP address.

Database credentials
After database configuration, you can use an existing user with the name “Neo4j“
Please make sure to use the correct username and password in the Basic method.
AuthTokens.Basic([Username], [Password])
Register IGraphClient in API IoC Container
Update ConfigureServices() to inject the Neo4j driver interface in the API pipeline as below,

As shown above, I have used the GraphClient instance as Singleton. That means the same driver instance will be used for every request from the API.
It’s recommended to create a single instance per application.
Above I have hardcoded the connection string but ideally, you can use IConfiguration or IOption interface to read the configuration or use it from any centralized storage.
Please see here for the implementation of IConfiguration using the Neo4j driver.
Dependency Injection IGraphClient in Controller
We shall now use the IGraphClient instance through Constructor Dependency Injection as below,

Performing CRUD operation on Graph Database
Let’s perform GET Operation using the Neo4j driver instance. To keep it simple, I have driver instance directly in the Controller. However one can look for Repository implementation for actual implementation.
Graph Cypher Query
Let’s write a simple query to get a list of the movies of an actor based on the name. Here we shall be using the relationship “ACTED_IN” to identify the list of movies acted by an actor called “Cuba Gooding Jr.”
@”(person: Person { name: ‘Cuba Gooding Jr.’})-[:ACTED_IN]->(movie: Movie)”
Below is the complete implementation for the Get or Reading record.

If not using Dependency injection
If you are not using Dependency injection, then the driver instance can be created directly as shown below,
var neo4jClient = new GraphClient(new Uri("http://localhost:7474/db/data"), "test", "test");
Note: If not using DI, then it will remain your responsibility to manage the lifetime of the instance.
Let’s perform a READ operation.

As you can see we are able to read all the movies of an actor along with its Personal details successfully!
The above is similar to the way other CRUD operations like Create, Delete or Update can also be defined.
Neo4j using Neo4j Bolt Driver
You can connect the Neo4j graph database using a bolt driver interface easily
Please see the below article for more details.
Neo4jClient using Repository pattern
You can use a Repository pattern to abstract the Database operation and address the Database query in a more generic way.
Please see the below article for more details,
That’s all!
All right friends! This was very basic on how to get started with Neo4jClient in ASP.NET Core API application.
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to use Neo4jClient to connect the Neo4j Graph DB server and execute Cypher queries with built-in IntelliSense support. We looked at simple and easy-to-understand ASP.NET Core implementation performing basic Read Cipher queries on the NeO4j database.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.