Hosting ASP.NET Core App as Service and Window Service -Part I

In this article, we shall see how to enable Create ASP.NET Core App as Windows Service.
We will enable ASP.NET Core 3.1 or 6.0 API as a Service that will act as a traditional service listening to business events continuously.
This approach is especially useful if your API design follows Choreography or Orchestration architecture for microservices development.
Today in this article, we will cover below aspects,
Alternatively, background tasks and scheduled jobs are also useful even if you don’t follow the microservices architecture pattern.
In the below example, I am going to demonstrate both use cases in detail.
Recently I had a requirement to create an ASP.NET Core API with the ability to subscribe to messages/events.
The service needed to be hosted in the cloud with the ability to subscribe to a business event/messaging to happen.
This I wanted to achieve aside from controller HTTP endpoints exposed for other responsibilities.
Basically, the producer will produce a message and the service will act as a consumer will consume these messages as and when those events are available.

Once it receives the message the messages would be flown to the downstream database and respective services.
Getting started
Create ASP.NET Core API using 3.1 or 6.0 .NET Core version.
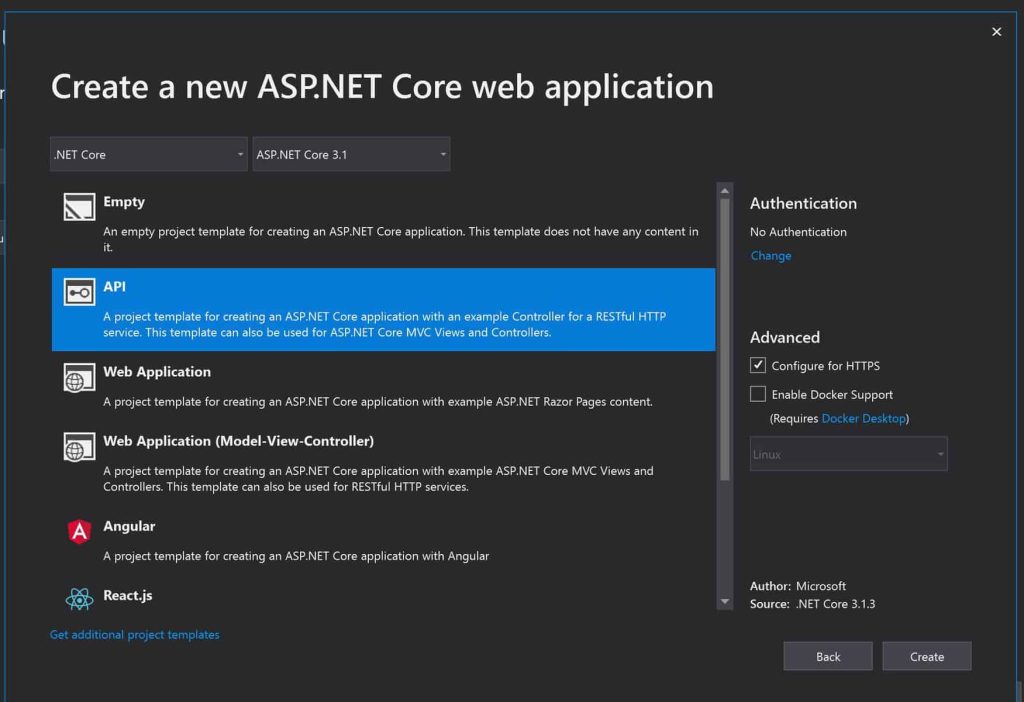
Create service using IHostedService
Please update the Startup.cs for the following code.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddHostedService<TheCodeBuzzConsumer>();
}
In the above code, we have used AddHostedService() an extension to register Microsoft.Extensions.Hosting.IHostedService to register.
This was using class TheCodeBuzzConsumer which ultimately indirectly implements IHostedService which is explained below.
Alternatively, you can register HostedService below as well,
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
})
.ConfigureServices((hostContext, services) =>
{
services.AddHostedService<TheCodeBuzzConsumer>();
});
}
Create Backgroundworker class
Create a worker class called TheCodeBuzzConsumer.
This class needs to be derived from the class abstract BackgroundService.
Here With .NET Core 3.0, a background worker i.e here our custom class TheCodeBuzzConsumer can be created using Visual Studio or the dotnet CLI command
dotnet new worker
The default Worker templates look as below. You can customize it as per your requirements.
public class Worker: BackgroundService
{
private readonly ILogger<Worker> _logger;
public Worker(ILogger<Worker> logger)
{
_logger = logger;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
Console.WriteLine($"Consumed messages");
await Task.Delay(1000, stoppingToken);
}
}
}
I have renamed Worker class above as TheCodeBuzzConsumer.
StartAsync and StopAsync method
This abstract class exposes a method called ExecuteAsync() which needs to be derived by our worker class.
BackgroundService class also gives you access to a few other virtual methods like which can be used as needed.
- StartAsync() and
- StopAsync()
Understanding ExecuteAsync
ExecuteAsync is an abstract method that is needed to implement the derived class.
This method gets called as soon as IHostedService starts.
The implementation should return a task that represents the lifetime of the long-running operation(s) being performed.
Use this method using a while loop i.e until cancellation is requested.
Once executed the method starts invoking and executes every after 1 second,

Above is the plain vanilla implementation for you to get started.
You can extend the above implementation for any of the use cases you are dealing with.
For more details on how I am implementing it for the Kafka messaging pattern see the article here
If you want to create ASP.NET Core API as Service using another approach i.e by using UseWindowsService please do visit the below article for more information.
- Create ASP.NET Core App as Windows Service using UseWindowsService
- Getting Started with Kafka in ASP.NET Core
That’s All! Thank you!
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Summary
Today in this article we learned how to enable ASP.NET Core API or MVC application as a microservice so that one can leverage Pub-Sub capability or Event-driven capability into your existing application.
This technique is useful when dealing with creating Message/Event-driven architecture-based applications using example MQ( RabbitMQ or IBM MQ) or Kafka.
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.