MongoDB C# Driver Date range query greater than or less than

Today in this article will cover MongoDB C# .NET – Date Range query greater than or less than scenarios.
We will see how to get records based on timestamp or date range queries like greater than or less than time or date etc.
We shall see date range queries using C# MongoDB driver in .NET or .NET Core application.
Today in this article, we will cover below aspects,
We already looked at a simple way of adding or updating MongoDB document a new field to the document in our previous MongoDB sample series.
Please visit the below article to understand the basics of adding MongoDB driver and performing CRUD operations with examples scenarios,
Getting started
Please create any .NET/.NET Core application.
Please add the MongoDB driver NuGet package using the Nuget Package manager.
PM> Install-Package MongoDB.Driver -Version <version> Note: Please use the latest driver available
I shall keep the MongoDB driver interface used here simple enough to concentrate on how to query documents based on date range query
Please visit for better approaches like using DI or IOC here,
Step I – Establish the connection to the Database using the MongoDB driver,
var _mongoClient = new MongoClient("mongodb://your connection string"); var db = _mongoClient.GetDatabase("your database name");
Step II – Get all the Records from the given collection,
More Typesafe way,
Example
IMongoCollection<Book> collection = db.GetCollection<Book>("Book");
Or
Example
Generic way
var collection = db.GetCollection<BsonDocument>("Book");
Note: If you need to implement batch update or chunk update asynchronously, please visit the below article.
Here below is the actual sample schema or document we shall use for the date range query,
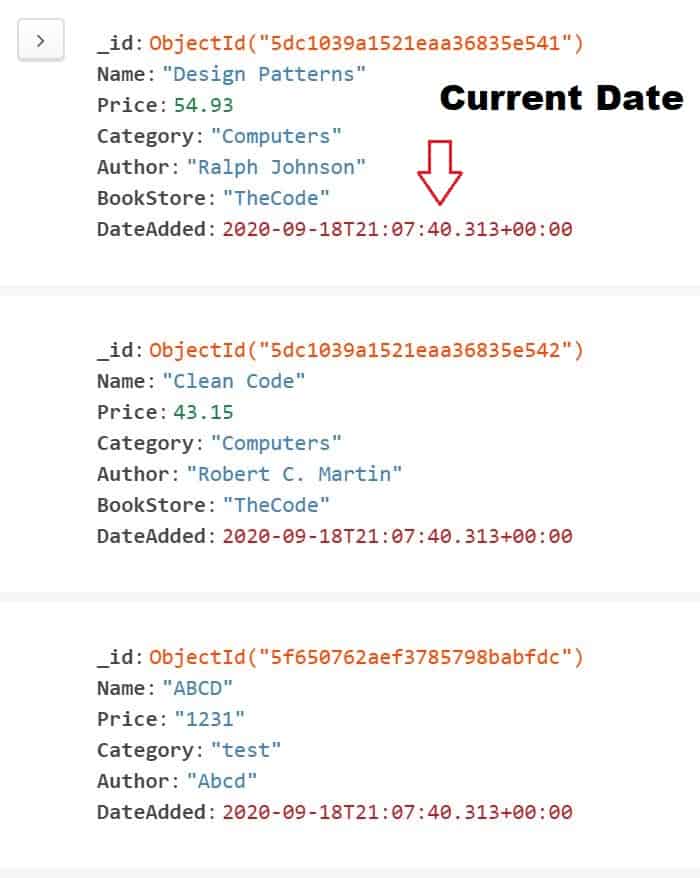
Here we will be using the below query pattern to get the documents between two dates in MongoDB Collection using the C# query.
Pattern:
{ DateAdded : { $gt:ISODate('Date here'), $lt:ISODate('Date here')}}
Above query, the pattern can be created using C# code easily as explained below.
Let’s prepare a similar query as below,
C# query greater than date
Below is the query to get the records based on greater than dates.
var dateQuery = new BsonDocument
{
{"DateAdded" , new BsonDocument {
{ "$gt" , fromDate}
}}
};
C# mongo query less than date
Below is the query to get the records based on greater than dates.
var dateQuery = new BsonDocument
{
{"DateAdded" , new BsonDocument {
{ "$lt" , toDate}
}}
};
Get the records between two dates
Below is the query to get the records based on greater than dates.
var dateQuery = new BsonDocument
{
{"DateAdded" , new BsonDocument {
{ "$gt" , fromDate},
{ "$lt" , toDate}
}}
};
What is the difference between gt and gte or lt and lte in MongoDB ?
There is simple differences between these commands
gte
gte = greater than or equal to i.e > =
gt
gt = greater than i.e >
lte
lte = less than or equal to i.e < =
lt
lt = less than i.e <
Example:
Let’s use the above date range query for C# code as below,
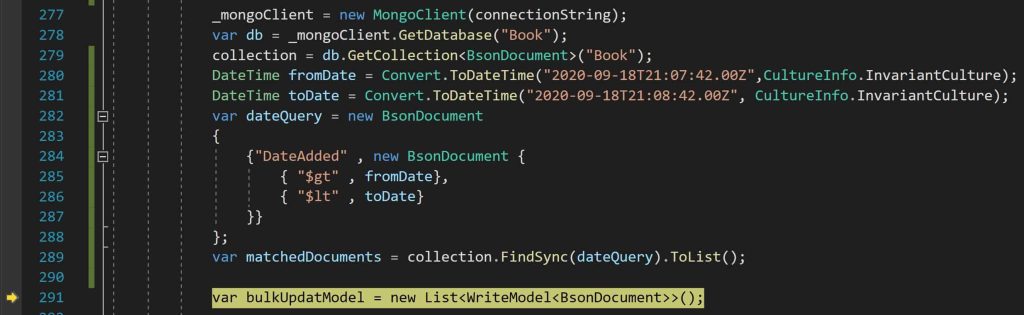
Finally, we shall see the successful results.
If your schema, doesn’t have the “DateAdded” field then you can use the ObjectID field as well i.e _id fields to get time and query using a timestamp.
However using these fields does have some limitations. Please see below article for more details,
References:
Do you have any comments or ideas or any better suggestions to share?
Please sound off your comments below.
Happy Coding !!
Please bookmark this page and share it with your friends. Please Subscribe to the blog to receive notifications on freshly published(2024) best practices and guidelines for software design and development.
temos algum parametro para igual ignorando a hora.
This was very helpful, Thank you
Thanks Andrew, Glad that the article was helpful.